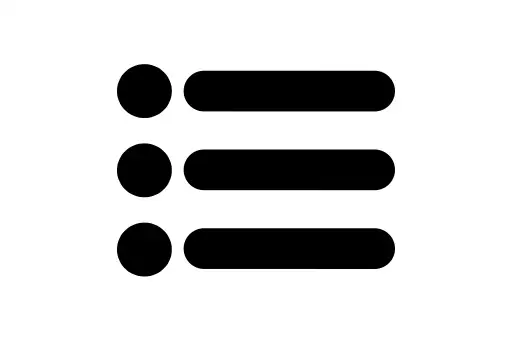
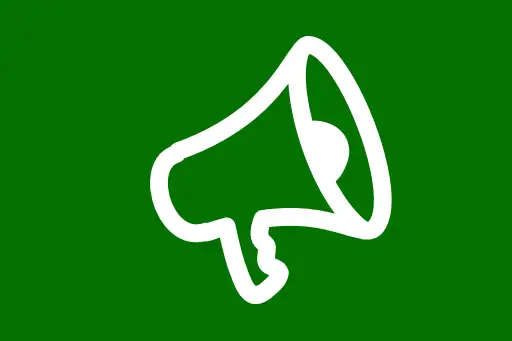
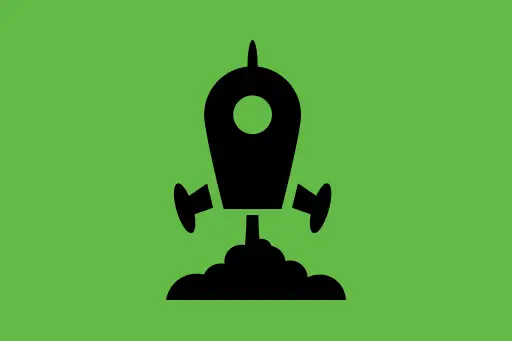
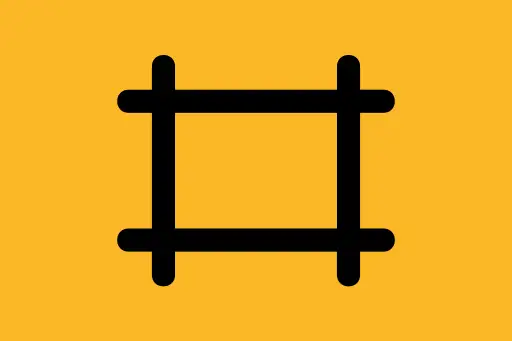
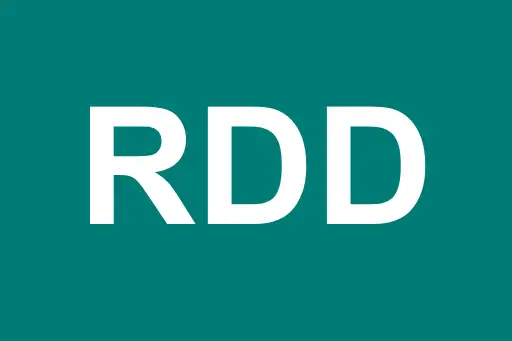
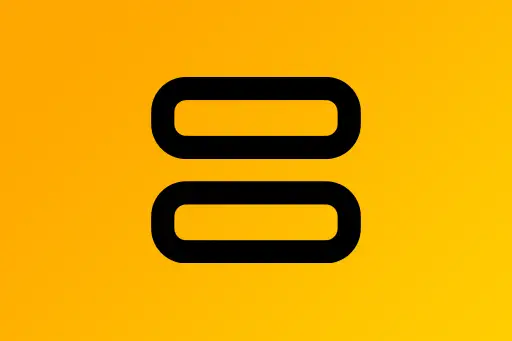
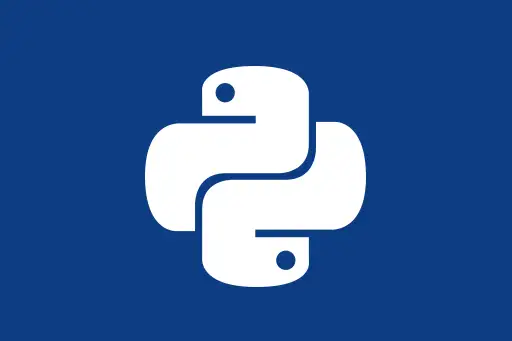
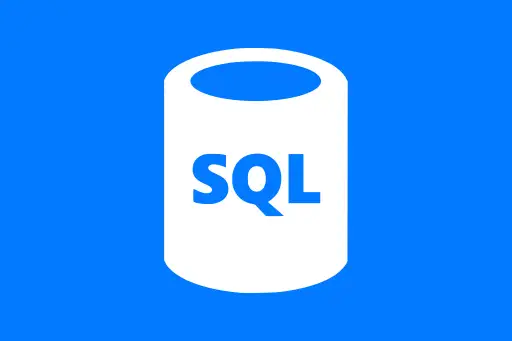
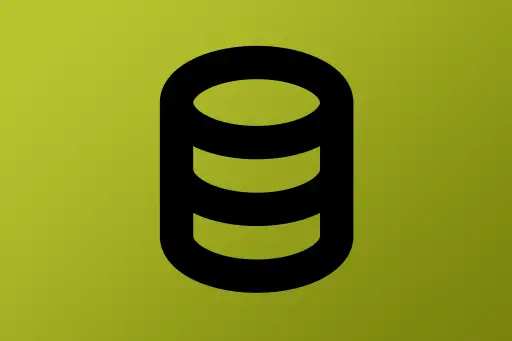
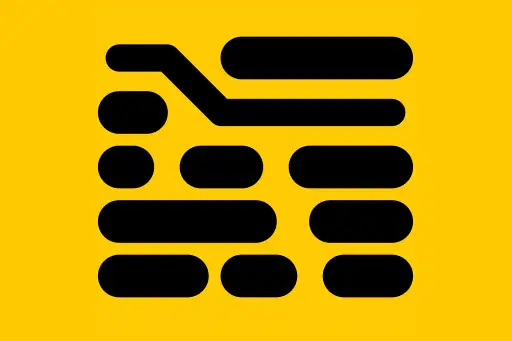
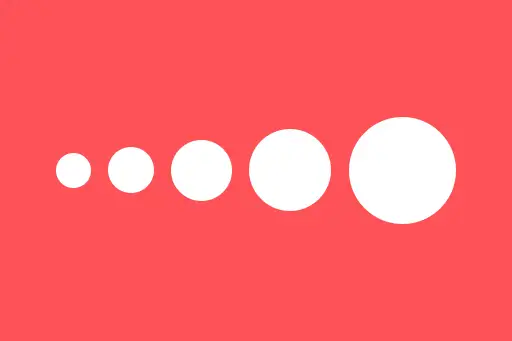
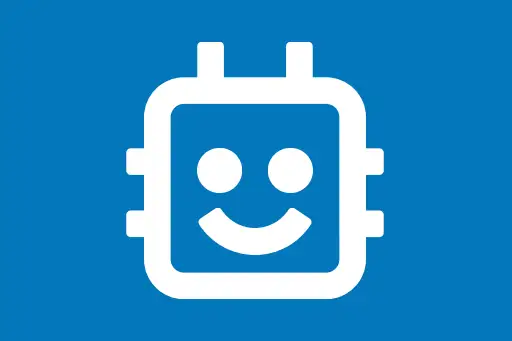
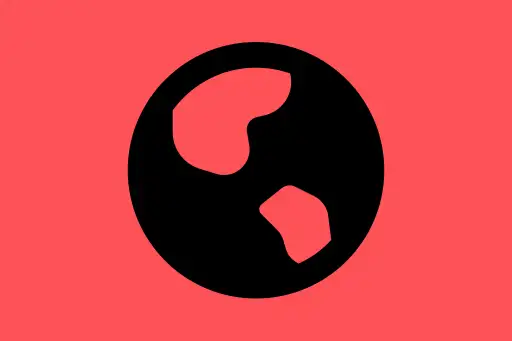
Why DataFrames over RDDs?
Why DataFrames over RDDs?
In Apache Spark, both RDDs (Resilient Distributed Datasets) and DataFrames are used to handle large-scale data. However, DataFrames are preferred in most practical scenarios today because they are more expressive, optimized, and easier to work with, especially for beginners.
Understanding the Difference
Let’s start by comparing RDDs and DataFrames on some key points:
Aspect | RDD | DataFrame |
---|---|---|
Data Structure | Low-level object-oriented API | Higher-level tabular data abstraction (like a table) |
Ease of Use | Requires more code | Fewer lines, more readable |
Optimization | No automatic optimization | Optimized by Catalyst engine |
Performance | Slower for structured data | Faster with built-in optimizations |
Use Case | Low-level transformations, unstructured data | Structured data analytics |
Real-Life Analogy
Imagine you are managing data in two different ways:
- RDD is like working with a pile of documents. You can process each manually, but it takes effort and time.
- DataFrame is like using an Excel sheet where data is neatly organized in rows and columns — and you can apply filters, calculations, and groupings very easily.
Example: Analyzing Sales Data with RDD
Let’s say we have sales data stored as plain text and we want to compute the total sales per product.
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("RDD vs DataFrame").getOrCreate()
rdd = spark.sparkContext.textFile("sales.txt")
# sales.txt contains lines like: "ProductA,100"
rdd_parsed = rdd.map(lambda line: line.split(","))
sales = rdd_parsed.map(lambda x: (x[0], int(x[1])))
total_sales = sales.reduceByKey(lambda x, y: x + y)
print(total_sales.collect())
[('ProductA', 300), ('ProductB', 450), ('ProductC', 200)]
Question:
What problems can you spot here?
Answer:
We have to manually parse and typecast data, and write custom functions. There’s no schema, and debugging errors is hard.
Same Task Using DataFrame
Let’s see how much easier this becomes with a DataFrame.
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("DataFrame Example").getOrCreate()
# Create DataFrame from a CSV file
df = spark.read.option("header", "true").csv("sales.csv")
# Convert sales column to integer
df = df.withColumn("amount", df["amount"].cast("int"))
# Group by product and get total sales
df.groupBy("product").sum("amount").show()
+--------+-----------+ | product|sum(amount)| +--------+-----------+ |ProductA| 300| |ProductB| 450| |ProductC| 200| +--------+-----------+
Why This Matters for Beginners
- With DataFrames, you don’t need to write transformation logic line-by-line.
- You can easily query data like SQL.
- DataFrames automatically optimize your code behind the scenes using the Catalyst optimizer.
Question:
Can I still use functions and logic with DataFrames?
Answer:
Yes! You can use SQL-like queries and also integrate Python logic using withColumn
, filter
, groupBy
, and user-defined functions (UDFs).
Performance Benefits
Spark's Catalyst optimizer helps DataFrames run faster by generating optimized execution plans. RDDs don’t have this advantage, which leads to slower performance, especially with large datasets.
Conclusion
DataFrames are better than RDDs for most practical use cases, especially when working with structured data. They are faster, easier to code, and integrate seamlessly with Spark SQL and ML pipelines.
As a beginner, starting with DataFrames will help you write clean, optimized, and scalable code more easily.