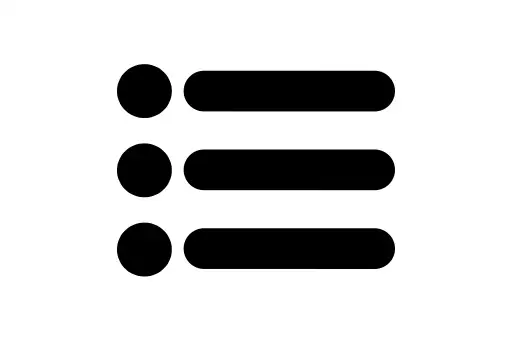
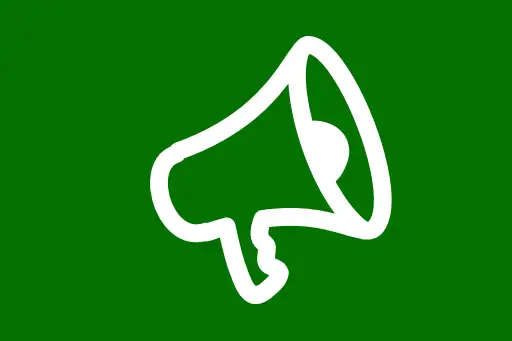
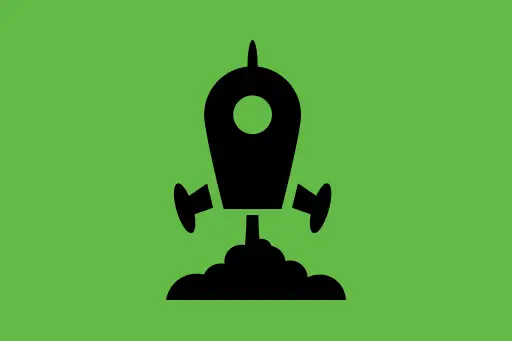
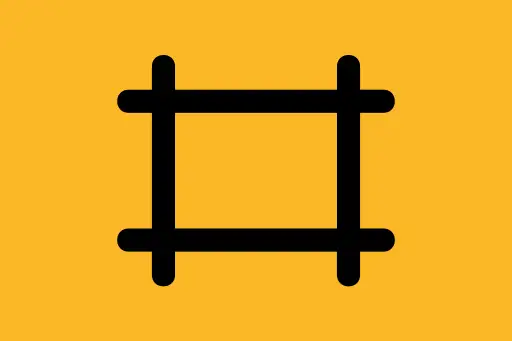
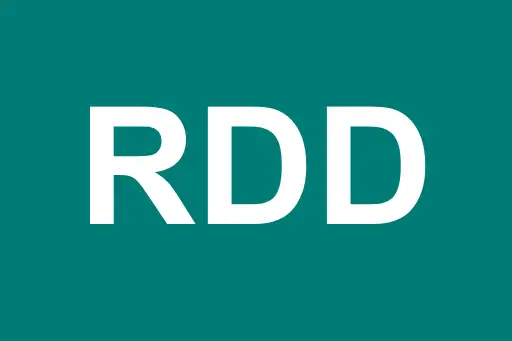
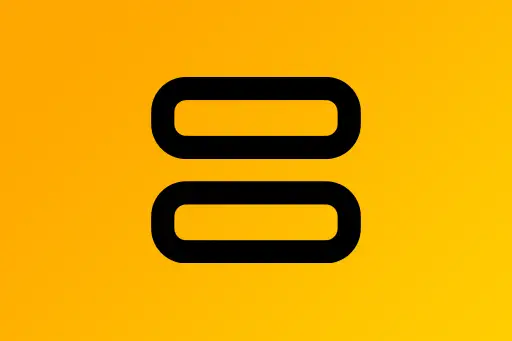
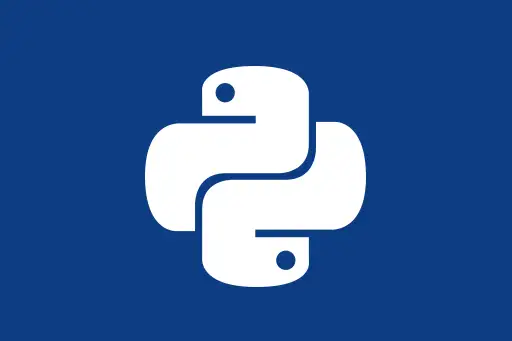
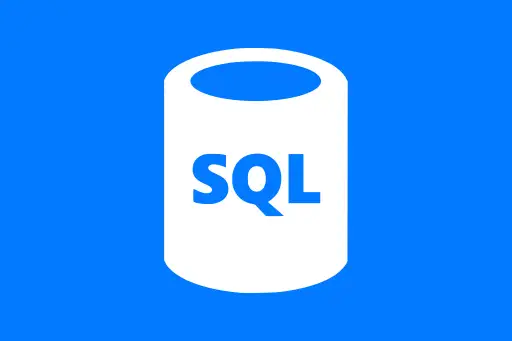
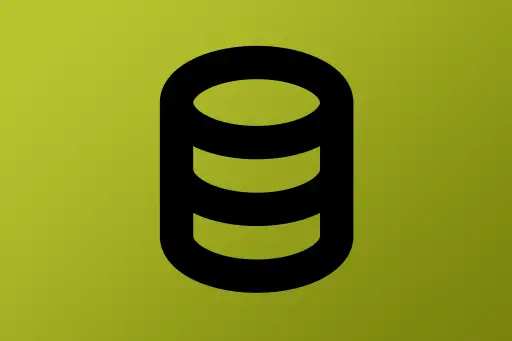
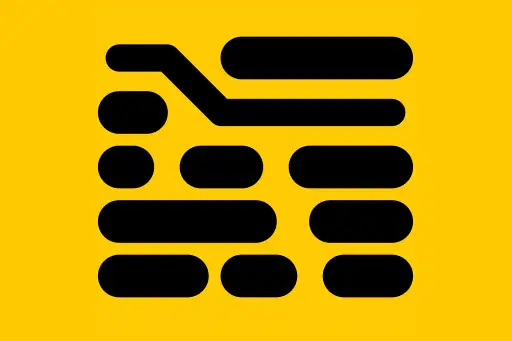
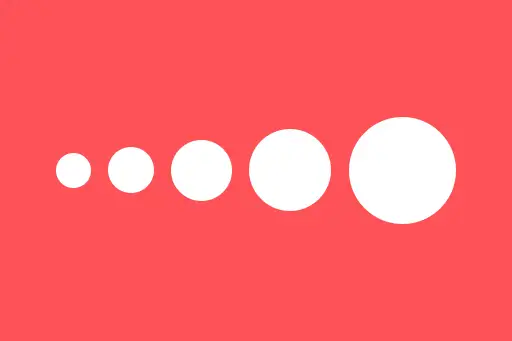
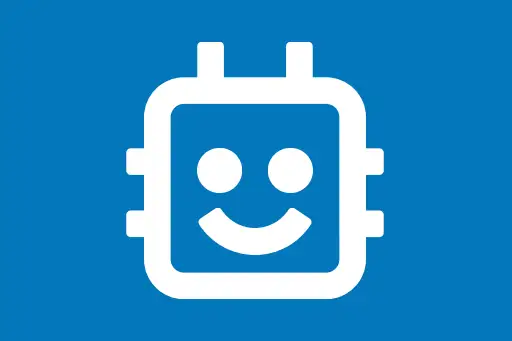
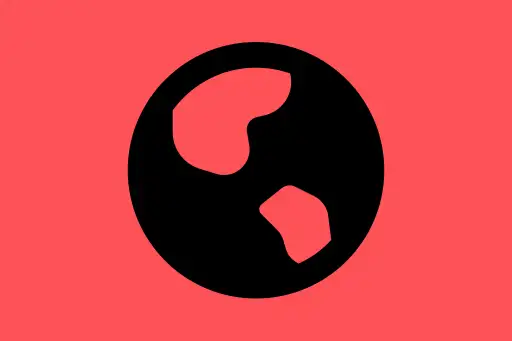
Limitations of RDDs
Limitations of RDDs
RDDs (Resilient Distributed Datasets) were the original data abstraction in Apache Spark, offering low-level control over data processing. However, as Spark evolved, RDDs began to show certain limitations — especially for large-scale, structured, and optimized data tasks.
1. Lack of Optimization
RDDs do not take advantage of Spark’s powerful query optimizer — the Catalyst optimizer — which means they often result in less efficient execution plans.
Example: Filtering a dataset
Let’s say we want to filter even numbers from a large dataset.
rdd = spark.sparkContext.parallelize(range(1, 10001))
even_numbers = rdd.filter(lambda x: x % 2 == 0)
print("Even count:", even_numbers.count())
Even count: 5000
This works, but Spark treats it as a black box — it doesn’t know what lambda x: x % 2 == 0
means internally. It cannot optimize or reorder this operation for better performance.
Question:
Why is optimization important in large-scale data?
Answer:
When working with huge datasets (terabytes or petabytes), even small inefficiencies can cause delays and increase costs. Optimizations can reduce time and memory usage drastically.
2. No Inbuilt Schema Support
RDDs do not understand the structure of your data. Every record is treated as a generic object (often tuples), making it hard to perform operations like filtering columns, joining datasets, or aggregating by column name.
Example: Tuple-based record in RDD
data = [("Alice", 28), ("Bob", 35), ("Carol", 22)]
rdd = spark.sparkContext.parallelize(data)
# Trying to filter by name length
filtered = rdd.filter(lambda x: len(x[0]) > 3)
print(filtered.collect())
[('Alice', 28), ('Carol', 22)]
We had to use x[0]
and x[1]
— not very readable. Compare this with DataFrames where we can use column names directly.
Question:
What’s the drawback of not having column names?
Answer:
It reduces code clarity and increases chances of errors, especially when dealing with complex or nested data structures.
3. Verbose Code for Simple Operations
Common data tasks like grouping, joining, or aggregating are more verbose with RDDs than with DataFrames.
Example: Counting word occurrences
words = ["apple", "banana", "apple", "orange", "banana", "apple"]
rdd = spark.sparkContext.parallelize(words)
word_counts = rdd.map(lambda word: (word, 1)) \
.reduceByKey(lambda a, b: a + b)
print(word_counts.collect())
[('orange', 1), ('banana', 2), ('apple', 3)]
While this works, doing the same with a DataFrame would be just one line with groupBy and count — cleaner and easier to read.
4. No Built-in Support for Structured Queries
RDDs cannot be queried using SQL. You can't write something like SELECT name FROM people WHERE age > 30
directly on an RDD.
This limits RDDs for users who are familiar with SQL or prefer declarative querying.
5. Not Suitable for Machine Learning Pipelines
Most machine learning libraries in Spark (like MLlib) expect structured input as DataFrames. RDDs are not compatible with newer APIs and pipelines.
Question:
Should you use RDDs for building ML pipelines in Spark?
Answer:
No. Use DataFrames or Datasets because they support schema, metadata, and Spark ML pipelines directly.
When Should You Use RDDs?
- When you need low-level control over data transformation
- When you are working with unstructured data and custom serialization
- For backward compatibility with older Spark applications
Summary
RDDs are powerful and flexible, but they come with limitations — lack of schema, optimization, and high verbosity. For most modern applications, Spark DataFrames or Datasets are preferred because they are faster, easier to use, and integrate well with Spark SQL and MLlib.
Comments
Loading comments...