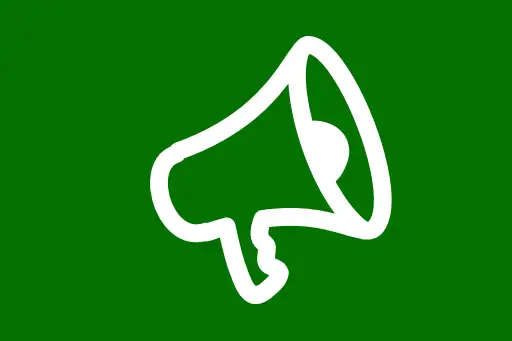
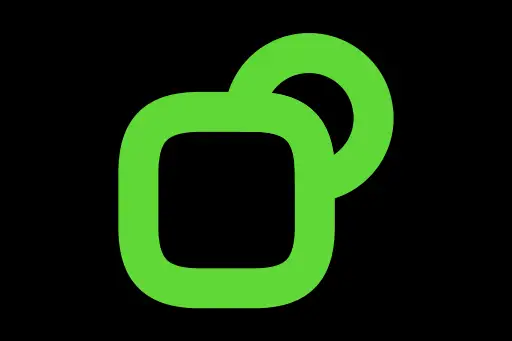



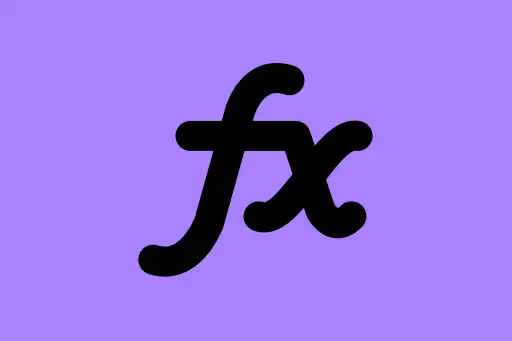


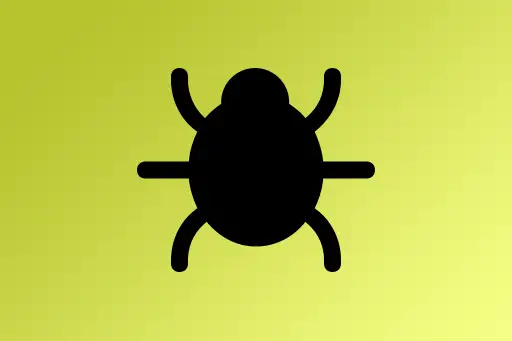


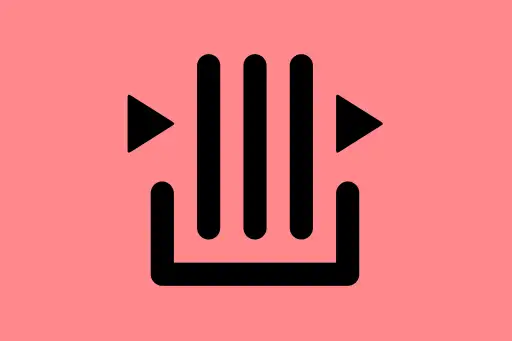

Working with Text and Binary Files
Introduction to File I/O
File I/O (Input/Output) is a fundamental concept in programming. It allows programs to interact with files stored on disk—either by reading data from them or writing data into them. Files are usually categorized as either text files or binary files.
Text vs Binary Files
- Text Files: Store data as readable characters. These files are human-readable and can be opened in text editors.
- Binary Files: Store data in raw bytes. These files are compact and efficient but not human-readable.
Working with Text Files
Let’s start with reading and writing text files. This is the most common form of file I/O for logs, reports, config files, etc.
Example 1: Writing to a Text File
// Pseudocode to write lines into a text file
OPEN file "report.txt" in write mode
WRITE "Report for Q1" to file
WRITE "Total Sales: $45000" to file
CLOSE file
report.txt ------------- Report for Q1 Total Sales: $45000
Question:
What happens if we open the file in write mode again?
Answer: The file will be overwritten. Any existing content will be erased.
Example 2: Reading from a Text File
// Pseudocode to read a text file line by line
OPEN file "report.txt" in read mode
WHILE not end of file
READ line from file
DISPLAY line
END WHILE
CLOSE file
Report for Q1 Total Sales: $45000
Working with Binary Files
Binary files store data in its raw format. They are often used for images, compiled code, audio, or structured data.
Example 3: Writing to a Binary File
// Pseudocode to write integers to a binary file
OPEN file "data.bin" in binary write mode
WRITE integer 1234 to file
WRITE integer 5678 to file
CLOSE file
Example 4: Reading from a Binary File
// Pseudocode to read integers from a binary file
OPEN file "data.bin" in binary read mode
READ integer from file and store in variable a
READ next integer and store in variable b
DISPLAY a, b
CLOSE file
1234 5678
Question:
Can we open a binary file in a text editor and still read it?
Answer: No. Binary files contain raw bytes which may not map to valid text characters. They can appear as gibberish or corrupt content in text editors.
Best Practices
- Always close files after reading or writing to avoid memory leaks.
- Use appropriate modes: text vs binary, read vs write vs append.
- Validate file existence and permissions before accessing.
- Handle exceptions during file operations to avoid crashes.
Conclusion
Mastering file I/O helps in building real-world applications that store and retrieve data persistently. Understanding the difference between text and binary formats enables better decision-making when dealing with file storage and processing.
Comments
Loading comments...