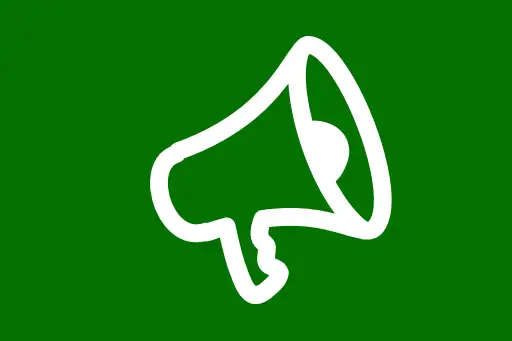
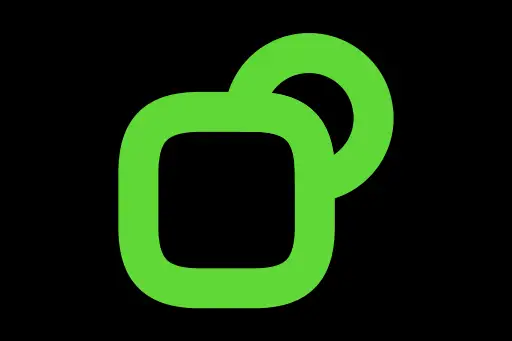



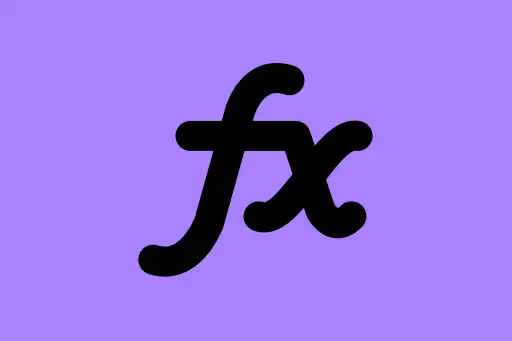


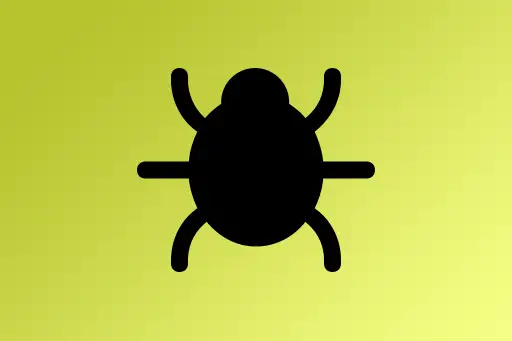


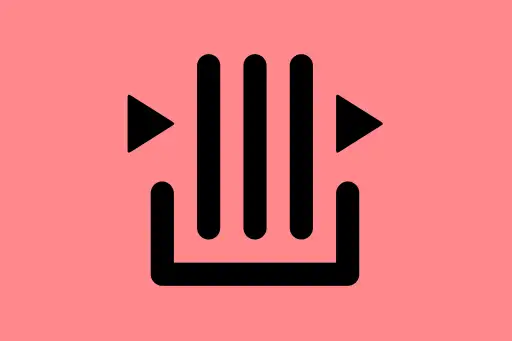

What Are Functions in Programming
Definition, Examples, and Purpose
What Are Functions?
In programming, a function is a named block of code that performs a specific task. It can be defined once and called (or reused) multiple times, making code modular, clean, and easier to maintain.
Why Use Functions?
- To avoid repeating code (DRY principle: Don’t Repeat Yourself)
- To break down complex problems into smaller, manageable parts
- To improve code readability and debugging
- To reuse logic across different parts of the program
Function Structure
At a basic level, a function includes:
- A name
- Optional parameters (inputs)
- A body with logic
- Usually a return value
function greetUser(name):
print "Hello, " + name + "!"
Here, greetUser
is the function name. It takes one parameter name
and prints a greeting message.
How to Call a Function
Once defined, a function can be called using its name followed by parentheses containing arguments:
greetUser("Alice")
Hello, Alice!
Example 1: Function Without Parameters
function showWelcomeMessage():
print "Welcome to the Programming Course!"
showWelcomeMessage()
Welcome to the Programming Course!
This function doesn’t take any input but simply prints a message when called.
Example 2: Function With Return Value
function add(a, b):
result = a + b
return result
sum = add(5, 3)
print sum
8
The add
function takes two inputs and returns their sum, which can be stored or printed.
Let’s Think:
Question: Can a function return nothing?
Answer: Yes. Some functions perform actions (like printing to screen or saving a file) and do not need to return a value.
Example 3: Function Returning Nothing
function logActivity(activity):
print "Logging activity: " + activity
logActivity("User logged in")
Logging activity: User logged in
Function Reusability
Once you define a function, you can use it as many times as needed:
logActivity("File uploaded")
logActivity("Email sent")
Logging activity: File uploaded Logging activity: Email sent
Intuition Builder:
Question: What if you want to repeat a calculation many times?
Answer: Wrap it in a function! That way, you don’t repeat logic — just call the function with different inputs.
Conclusion
Functions are fundamental building blocks in programming. They help you:
- Organize code logically
- Make programs more readable
- Enable reuse and modularity
Mastering functions is essential to writing clean, scalable programs!