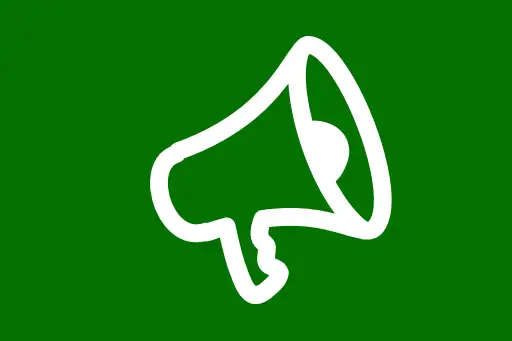
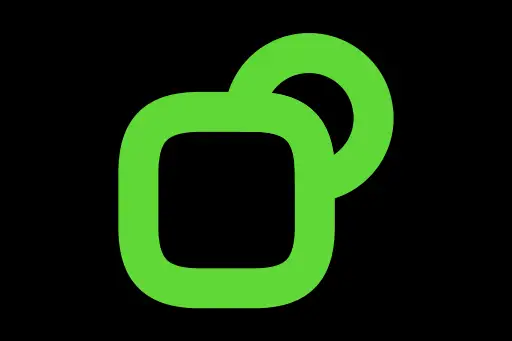



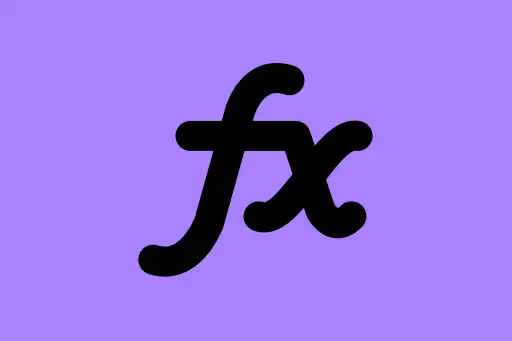


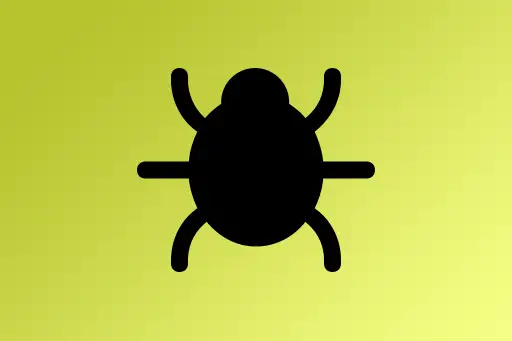


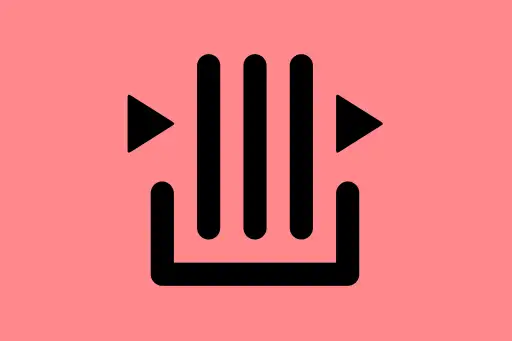

Understanding Variable Scope
Local, Global, and Nonlocal
What is Variable Scope?
Scope refers to the region of the code where a variable is defined and can be accessed. Understanding scope is essential to avoid bugs, naming conflicts, and unintended side effects in your code.
1. Local Scope
When a variable is declared inside a function, it is said to have local scope. It can only be accessed within that function and not outside of it.
function greet():
message = "Hello, world!" // Local variable
print(message)
greet()
print(message) // Error: message not defined outside function
Hello, world! Error: message is not defined
Why does this happen?
Because message
exists only inside the greet
function, it gets destroyed once the function finishes execution. Trying to access it outside leads to an error.
Question:
Can local variables from one function be used in another function?
Answer: No, unless passed explicitly as arguments. Each function has its own local scope.
2. Global Scope
A variable declared outside all functions or blocks is said to have global scope. It can be accessed from anywhere in the code.
status = "active" // Global variable
function showStatus():
print(status)
showStatus()
print(status)
active active
Modifying a Global Variable Inside a Function
To modify a global variable inside a function, you must explicitly declare it as global within that function.
count = 0 // Global variable
function increment():
global count
count = count + 1
increment()
print(count)
1
Question:
What happens if you try to change a global variable inside a function without declaring it as global?
Answer: It will create a new local variable with the same name, leaving the global variable unchanged.
3. Nonlocal Scope (Closure or Enclosing Scope)
A nonlocal variable comes into play when there is a nested function. A variable that is not local to the inner function but exists in an enclosing function’s scope is considered nonlocal.
function outer():
message = "Hi"
function inner():
nonlocal message
message = "Hello"
inner()
print(message)
outer()
Hello
Here, the inner function modified the message
defined in the outer
function using the nonlocal
keyword.
Question:
Is nonlocal
the same as global
?
Answer: No. nonlocal
affects variables in the nearest enclosing function, not in the global scope.
Summary Table
Scope Type | Where Defined | Accessible From | Can Be Modified Inside Function? |
---|---|---|---|
Local | Inside a function | Only within that function | Yes |
Global | Outside all functions | Anywhere in the code | Yes, with global keyword |
Nonlocal | In enclosing function | From nested inner functions | Yes, with nonlocal keyword |
Tips for Beginners
- Always be cautious when using global variables. They can make debugging harder.
- Use parameters and return values to pass data between functions instead of relying on global state.
- Nested functions can be powerful but require understanding of nonlocal scope.