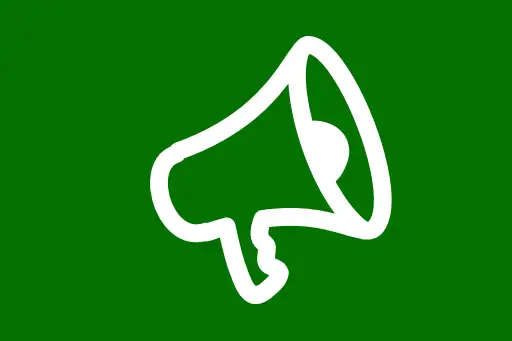
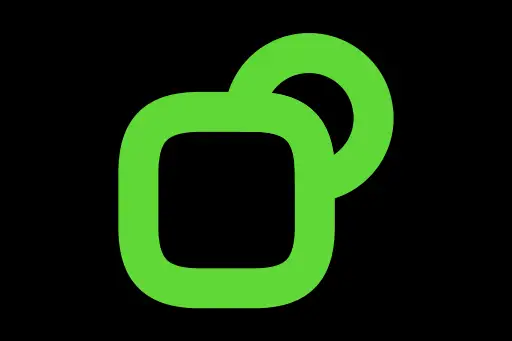



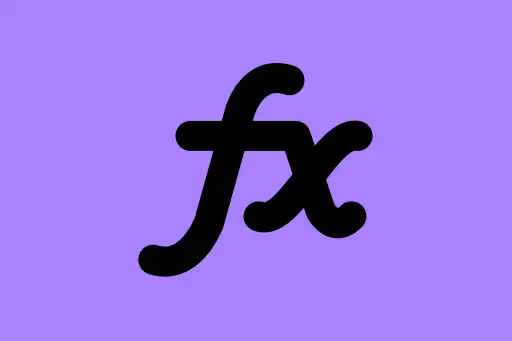


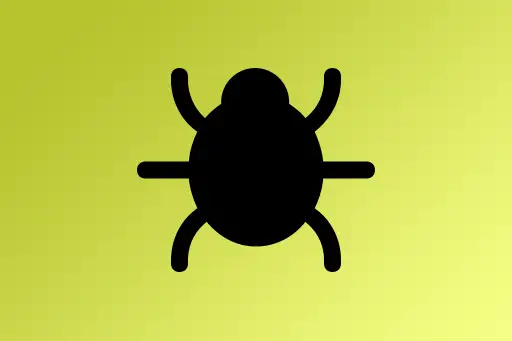


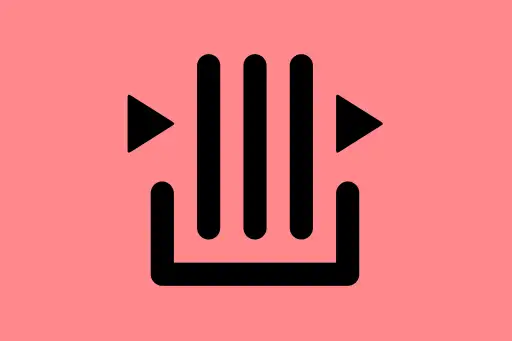

Understanding Try/Catch Blocks
Error Handling Explained
Next Topic ⮕Custom Exceptions
What are Try/Catch Blocks?
Try/Catch blocks are fundamental to error handling in programming. They allow you to gracefully handle errors that occur during the execution of your code, instead of letting your program crash unexpectedly.
The try
block contains code that might throw an error, and the catch
block defines how to handle that error if it occurs. This structure ensures your program remains in control even when something goes wrong.
Why Use Try/Catch?
- To avoid complete program termination due to unexpected issues.
- To provide user-friendly error messages.
- To handle specific types of errors differently.
Basic Try/Catch Structure
try:
// code that may cause an error
catch error:
// code to handle the error
Example 1: Handling Division by Zero
Let's consider a basic example where we divide two numbers, and we want to catch any division-by-zero errors.
try:
result = divide(10, 0)
print("Result is", result)
catch error:
print("An error occurred: Division by zero is not allowed")
An error occurred: Division by zero is not allowed
Explanation:
In the try
block, we attempt to divide 10 by 0. Since dividing by zero is invalid, an error occurs. Instead of crashing, the program jumps to the catch
block and prints a meaningful message.
Question:
What happens if no error occurs inside a try
block?
Answer:
If no error occurs, the catch
block is skipped entirely, and the program continues normally.
Example 2: Catching Errors with User Input
Imagine prompting a user for input that must be converted to a number. If the input is not valid, it would cause an error.
try:
input_value = get_input("Enter a number:")
number = convert_to_integer(input_value)
print("Double the number is:", number * 2)
catch error:
print("Invalid input. Please enter a valid number.")
Output (if user enters 'abc'):
Invalid input. Please enter a valid number.
Explanation:
If the user enters something like "abc"
, the conversion to integer fails and throws an error. The catch
block handles it and provides a helpful message instead of crashing the program.
Question:
Can I have multiple catch blocks for different error types?
Answer:
Yes, many programming languages allow multiple catch
blocks to handle specific error types separately. For example, one for division errors, another for file errors, etc.
Best Practices
- Only wrap the code that might fail inside the
try
block—not the entire program. - Always provide helpful messages in
catch
to guide users or developers. - Use specific error types (where supported) to handle different cases intelligently.
Example 3: Nested Try/Catch Blocks
Sometimes, you may need to use a try/catch inside another try/catch to handle errors at different levels.
try:
try:
value = get_input("Enter number:")
number = convert_to_integer(value)
result = divide(100, number)
print("Result:", result)
catch inner_error:
print("Inner error:", inner_error)
catch outer_error:
print("Something went very wrong:", outer_error)
Output (if user enters '0'):
Inner error: Division by zero
Summary
try/catch
blocks make your programs more robust and user-friendly by safely managing errors. Whether it’s a division error, a file that doesn’t exist, or bad input—handling it correctly ensures your program doesn’t crash and provides a smoother experience for the user.