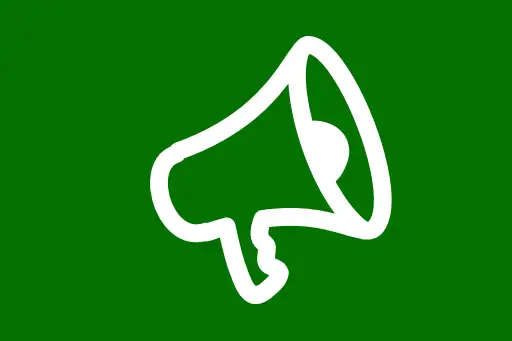
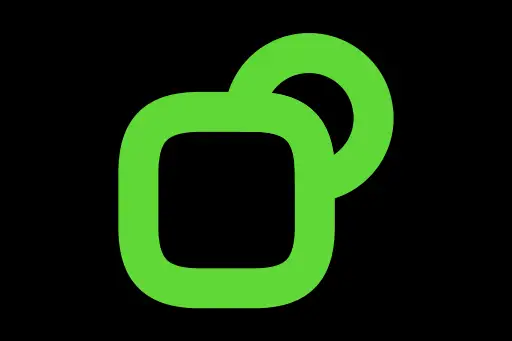



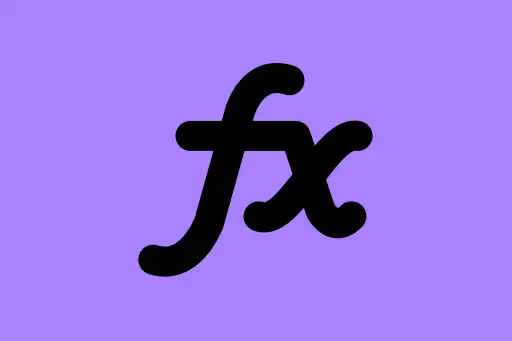


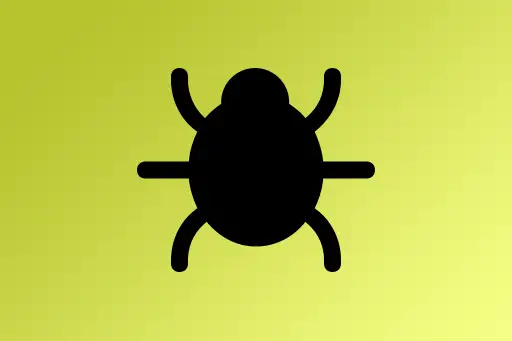


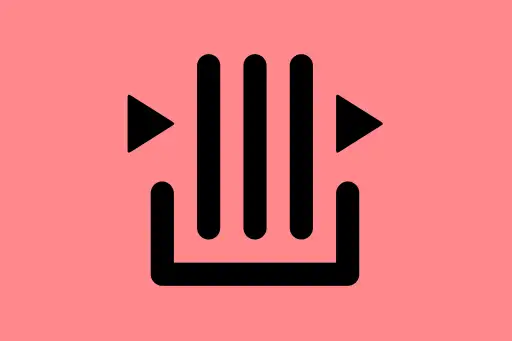

Testing and Code Reviews in Programming
Best Practices Explained
Why Testing and Code Reviews Matter
As your codebase grows, bugs become harder to spot just by reading the code. Testing and code reviews are essential practices to ensure reliability, correctness, and maintainability of your code.
Testing is the practice of verifying that your code behaves as expected. Code reviews involve another programmer looking over your code to identify mistakes, improve readability, and share knowledge.
Types of Testing
- Unit Testing: Tests small pieces of code (e.g., functions).
- Integration Testing: Tests how different parts of a system work together.
- System Testing: Tests the complete system from start to end.
- Regression Testing: Ensures new code doesn't break existing features.
Writing a Simple Unit Test
Let’s say you have a function that calculates the square of a number.
function square(x):
return x * x
To test this function, we write:
function test_square():
assert square(2) == 4
assert square(0) == 0
assert square(-3) == 9
All tests passed
Q: What happens if a test fails?
A: Your test framework should show which test failed and what was expected vs what was received. This gives you insight into where to debug.
Real-Life Debugging Example
Suppose we make a mistake in the function:
function square(x):
return x + x // Logical error
Running the same test now gives:
function test_square():
assert square(2) == 4 // Fails: returns 4, correct
assert square(-3) == 9 // Fails: returns -6, wrong!
Test Failed: square(-3) returned -6, expected 9
This guides us to fix the logic back to x * x
.
What is a Code Review?
A code review is the process where another developer inspects your code before it’s merged into the main codebase. The goal is to catch errors, enforce standards, and promote shared understanding of the system.
Best Practices During Code Review
- Keep changes small: Easier to review and test.
- Explain your changes: Use clear comments or a summary.
- Be respectful: Reviews are about the code, not the coder.
- Look for clarity: Is the code readable and maintainable?
- Check for side effects: Does the new code unintentionally affect other areas?
Code Review Example
Suppose a developer writes:
function getUserInfo(userId):
user = database.query("SELECT * FROM users")
return user
As a reviewer, you might ask:
- Shouldn't the query filter by
userId
? - What happens if the user doesn't exist?
- Is this query efficient?
How to Respond to Code Reviews
If you receive a review with suggestions:
- Take time to understand the feedback.
- Don’t take it personally—feedback is for your code, not you.
- Discuss if something is unclear or you disagree respectfully.
Q: Should I write tests before or after coding?
A: Ideally, write tests *before* coding (called Test-Driven Development), but even writing tests after is better than skipping them entirely.
Summary
Testing and code reviews aren't just for large companies—they're for anyone serious about writing good software. They reduce bugs, save time, and improve the quality of your codebase.
Practice Exercise
Try writing a function that checks if a number is even, and write unit tests for different inputs like 0, 2, 3, -4, etc.