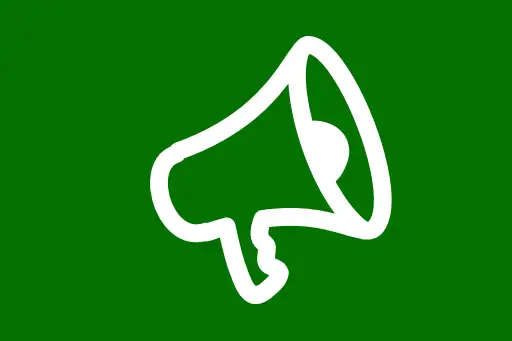
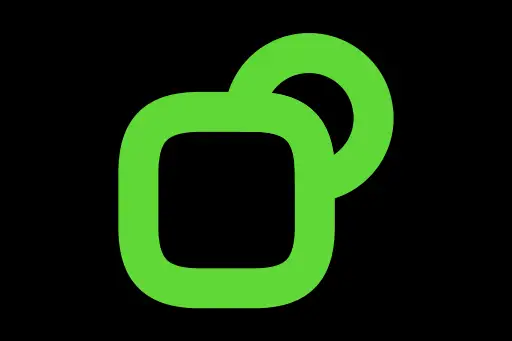



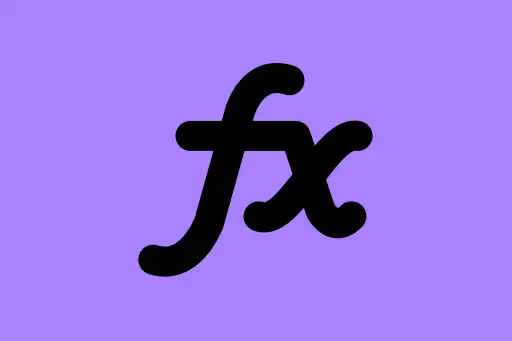


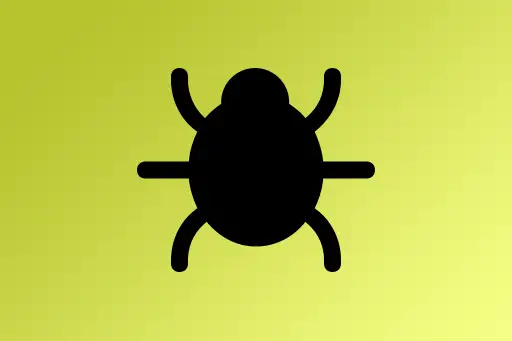


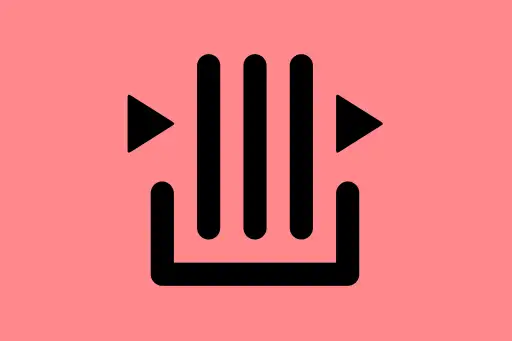

Synchronous vs Asynchronous Execution
Basics Explained with Examples
Next Topic ⮕Callbacks, Promises, and Futures in Programming
Understanding Execution Flow
In programming, how instructions are executed — whether they block the rest of the code or allow other tasks to run simultaneously — greatly affects program behavior and performance. This leads us to two essential concepts: synchronous and asynchronous execution.
What is Synchronous Execution?
In synchronous execution, tasks are performed one after another, in the exact order they are written. A task must complete before the next one begins. This makes the flow simple and predictable but can lead to delays if one task takes too long.
print("Start")
wait(3 seconds) // Simulating a blocking operation
print("End")
Start (wait 3 seconds...) End
Question:
What happens during the 3-second wait in synchronous code?
Answer: The program is paused and cannot do anything else until the wait finishes. This is called blocking.
What is Asynchronous Execution?
In asynchronous execution, tasks are initiated and allowed to complete at a later time, without blocking the rest of the code. This enables programs to remain responsive or perform multiple operations in parallel.
print("Start")
runAfter(3 seconds, function() {
print("Async Task Complete")
})
print("End")
Start End (wait 3 seconds...) Async Task Complete
Question:
Why does "End" print before "Async Task Complete"?
Answer: Because the asynchronous function does not block execution. It schedules the task and continues running the next lines immediately.
Comparing Both with a Realistic Analogy
Imagine you're cooking two dishes. In synchronous mode, you wait for the water to boil before chopping vegetables. In asynchronous mode, you start boiling the water, then chop vegetables while the water boils — making better use of your time.
Use Cases for Each
- Synchronous: Good for small, quick operations where order matters (e.g., math calculations, validation).
- Asynchronous: Best for time-consuming tasks like reading files, waiting for network responses, or loading large datasets.
Another Example: Reading a File
Synchronous Version
print("Reading file...")
data = readFile("data.txt") // blocks until done
print("File content:", data)
Reading file... (wait for file to load...) File content: [file content here]
Asynchronous Version
print("Reading file...")
readFileAsync("data.txt", function(data) {
print("File content:", data)
})
print("Continue with other tasks")
Reading file... Continue with other tasks (wait for file to load...) File content: [file content here]
Key Takeaways
- Synchronous: Blocks execution. Simple to write and debug.
- Asynchronous: Non-blocking. Efficient, especially for I/O and long-running operations.
Practice Thought
Imagine a program that processes 10,000 images one by one synchronously. What’s a better approach?
Answer: An asynchronous strategy that processes multiple images in parallel or in the background to save time and system resources.
Conclusion
Understanding the difference between synchronous and asynchronous execution is important for writing efficient, responsive, and scalable programs. While synchronous code is simpler to follow, asynchronous techniques are essential when dealing with real-world delays such as network latency or file system operations.