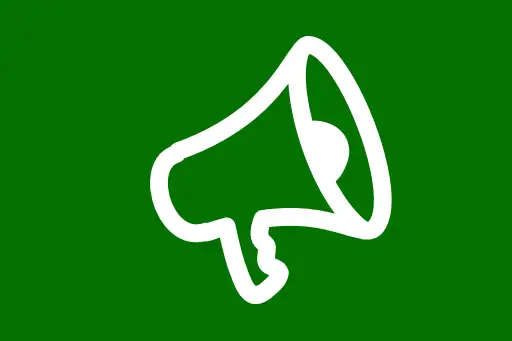
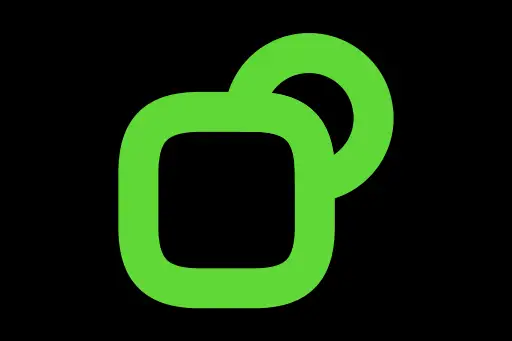



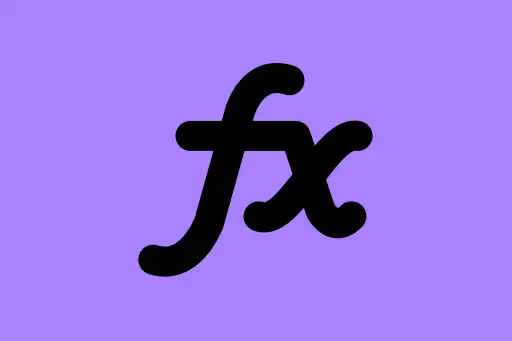


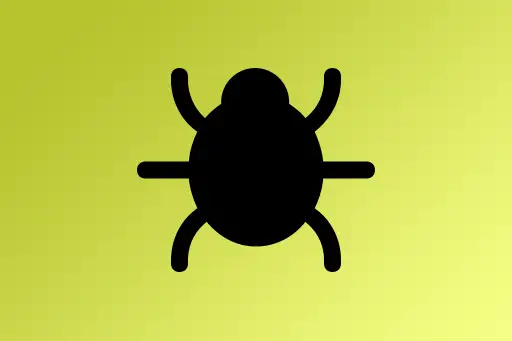


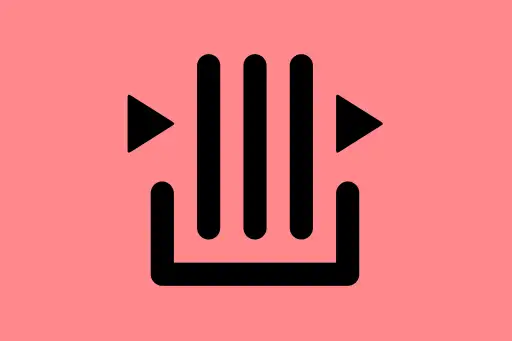

Modular Code and Project Organization
Best Practices in Programming
What is Modular Code?
Modular code refers to the practice of breaking down a program into independent, self-contained units or "modules." Each module handles a specific part of the program’s functionality and can be reused across different projects.
Why is Modularity Important?
- Improves readability and maintainability.
- Enables code reuse across projects or teams.
- Makes testing and debugging easier.
- Allows for team collaboration on larger projects.
Example 1: Without Modularity
Let’s say we are building a program to manage users and calculate taxes.
START
name = input("Enter user name")
income = input("Enter annual income")
if income > 50000 THEN
tax = income * 0.2
ELSE
tax = income * 0.1
ENDIF
print("User:", name)
print("Tax:", tax)
END
This works for a small program, but what if we need to calculate tax in multiple places or change tax rules?
Example 2: With Modularity
We separate logic into functions and group related tasks.
FUNCTION getUserInput
name = input("Enter user name")
income = input("Enter annual income")
RETURN (name, income)
END
FUNCTION calculateTax(income)
IF income > 50000 THEN
RETURN income * 0.2
ELSE
RETURN income * 0.1
ENDIF
END
FUNCTION printUserTax(name, tax)
print("User:", name)
print("Tax:", tax)
END
START
(name, income) = getUserInput()
tax = calculateTax(income)
printUserTax(name, tax)
END
Enter user name: Alice Enter annual income: 60000 User: Alice Tax: 12000.0
Question:
What if we had to change tax rates? How would modular code help?
Answer:
Instead of editing every tax calculation in the program, we only update the calculateTax
function—saving time and reducing error.
Organizing Project Files
As projects grow, organize code into folders and files:
project-root/
│
├── main.pseudo
├── user/
│ ├── input.pseudo
│ └── display.pseudo
├── tax/
│ └── calculator.pseudo
└── utils/
└── logger.pseudo
Benefits of Project Organization
- Separation of concerns: Keep business logic, user interaction, and utility code separate.
- Scalability: Add new features without cluttering the core logic.
- Team efficiency: Different team members can work on different modules independently.
Example 3: Calling from Organized Files
In your main file, import and use only what’s needed:
IMPORT getUserInput FROM user/input
IMPORT calculateTax FROM tax/calculator
IMPORT printUserTax FROM user/display
START
(name, income) = getUserInput()
tax = calculateTax(income)
printUserTax(name, tax)
END
Points to Remember
- Always group related logic into functions or files.
- Use folder structure to mirror code responsibility.
- Favor reusable modules instead of copy-pasting code.
- Each file/module should do one thing and do it well.
Quick Quiz
Q: Which of the following is a benefit of modular programming?
- A. Smaller codebase
- B. Easier to test and debug
- C. Better teamwork
- D. All of the above
Answer: D. All of the above