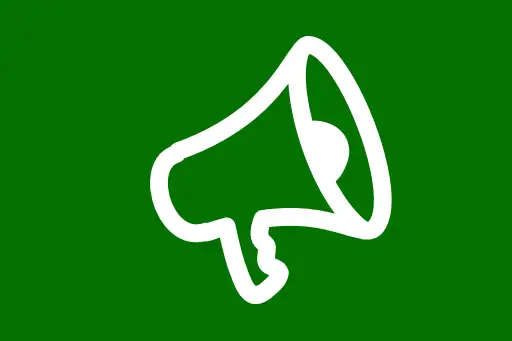
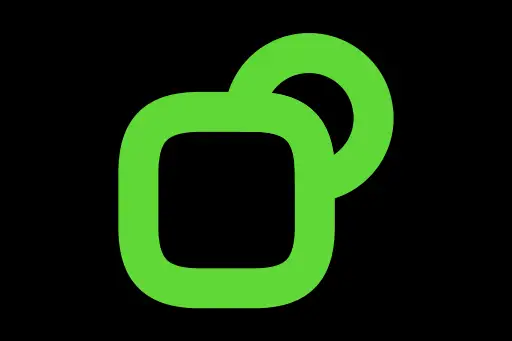



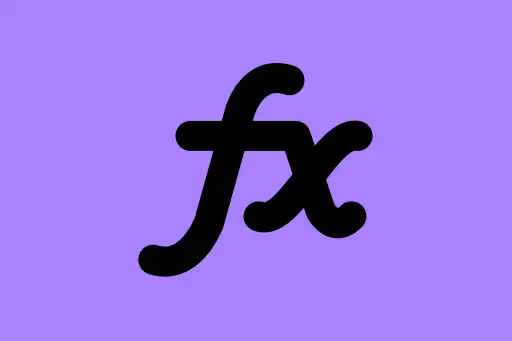


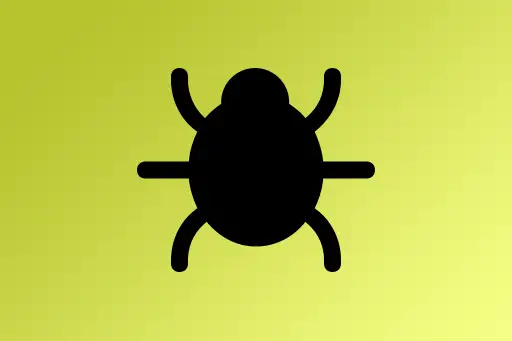


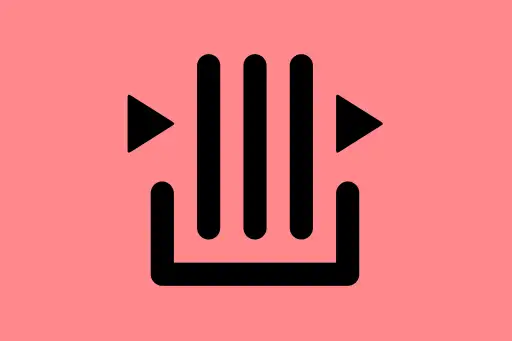

Instance vs Class Variables and Methods
OOPs Concepts Explained
Understanding Instance vs Class Variables and Methods
In object-oriented programming, variables and methods can be associated with either an instance (individual object) or a class (blueprint for objects). Knowing the difference is key to writing efficient, modular code.
Instance Variables and Methods
Instance variables are unique to each object created from a class. Each object gets its own copy, and changes made to one object’s variable do not affect others.
Instance methods operate on instance variables. They require an object to be called because they are designed to act on that object's data.
Class Variables and Methods
Class variables are shared across all objects of the class. There is only one copy, and changes to it reflect across all instances.
Class methods do not depend on instance data. They can access or modify class-level variables and are often used for utility or tracking purposes.
Pseudocode Example 1: Understanding Instance Variables
class Car:
method __init__(self, color):
self.color = color // instance variable
car1 = new Car("Red")
car2 = new Car("Blue")
print(car1.color)
print(car2.color)
Red Blue
Explanation: Here, each Car
object has its own color
value. Changing car1.color
won't affect car2.color
.
Question:
What will happen if we change car1.color = "Green"
after creating the objects?
Answer:
Only car1
will now have the color "Green". car2
will still be "Blue" since color
is an instance variable.
Pseudocode Example 2: Understanding Class Variables
class Car:
wheels = 4 // class variable shared by all cars
method __init__(self, color):
self.color = color
car1 = new Car("Red")
car2 = new Car("Blue")
print(car1.wheels)
print(car2.wheels)
Car.wheels = 6 // change class variable
print(car1.wheels)
print(car2.wheels)
4 4 6 6
Explanation: The variable wheels
is shared by all instances. Once we update it via the class name, the change reflects across all objects.
Question:
Can we access class variables through an instance like car1.wheels
?
Answer:
Yes, but it's recommended to access class variables using the class name (e.g., Car.wheels
) to make your code clearer.
Pseudocode Example 3: Instance vs Class Methods
class Counter:
count = 0 // class variable
method __init__(self):
Counter.count += 1
method get_instance_message(self):
print("I am an instance method.")
class method get_count():
print("Total instances:", Counter.count)
obj1 = new Counter()
obj2 = new Counter()
obj1.get_instance_message()
Counter.get_count()
I am an instance method. Total instances: 2
Explanation:
get_instance_message
is an instance method—it needs an object to be called and can access instance data.get_count
is a class method—it can be called without any object and is used to operate on class-level data.
When to Use What?
- Use instance variables when each object needs its own unique value.
- Use class variables when a value should be shared across all instances.
- Use instance methods when behavior depends on object-specific data.
- Use class methods when behavior relates to the class as a whole, like tracking or factory methods.
Quick Recap Table
Type | Scope | Usage |
---|---|---|
Instance Variable | Each Object | Holds object-specific data |
Class Variable | Shared by All Objects | Common property (e.g., counter) |
Instance Method | Called via Object | Accesses instance data |
Class Method | Called via Class | Accesses class data |
Practice Thought
If you were building a system where every user has a unique ID, but all users share the same access level initially, which variables would be instance and which would be class?
Answer:
User ID should be an instance variable since it’s unique for each user. Access level should be a class variable since it’s the same for everyone initially.