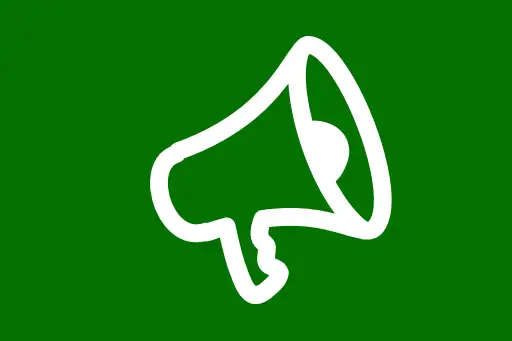
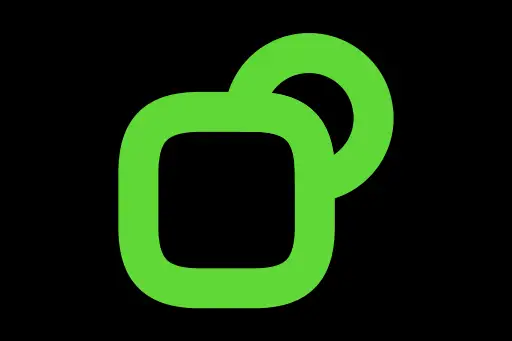



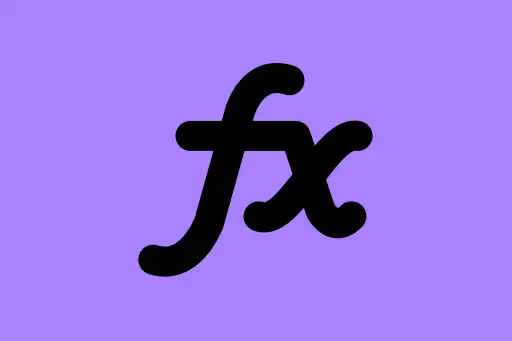


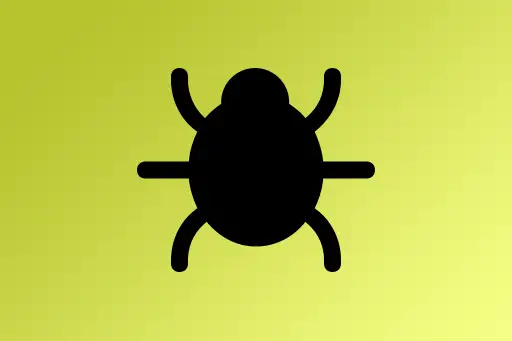


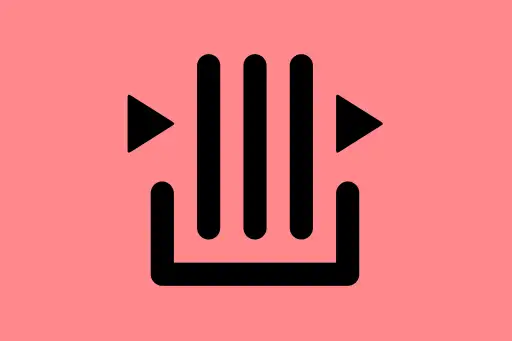

Inheritance in Programming
What is Inheritance?
Inheritance is one of the fundamental principles of Object-Oriented Programming (OOP). It allows a class (called the child class or subclass) to acquire the properties and behaviors (methods) of another class (called the parent class or superclass).
This means you can create new classes based on existing ones, avoiding code duplication and promoting reusability. Inheritance supports the idea of hierarchical classification — just like in the real world, where a "Dog" is a type of "Animal".
Why Use Inheritance?
- To promote code reuse.
- To establish a natural hierarchy.
- To enable polymorphism (explained in another topic).
- To enhance readability and maintainability of code.
Real-World Analogy
Imagine a blueprint for a general Vehicle that has properties like speed
and methods like move()
. Now suppose you want to define a Car. Instead of defining everything from scratch, you can let the Car
class inherit the properties and methods from Vehicle
, and add specific ones like openTrunk()
.
Basic Pseudocode Example
CLASS Vehicle
METHOD move()
PRINT "The vehicle is moving"
CLASS Car EXTENDS Vehicle
METHOD openTrunk()
PRINT "Trunk is now open"
// Create an object of Car
car1 = NEW Car()
car1.move() // Inherited from Vehicle
car1.openTrunk() // Specific to Car
The vehicle is moving Trunk is now open
How Does This Work?
The Car
class inherits the move()
method from Vehicle
. So when you create an object of Car
, it can use both the inherited method move()
and its own method openTrunk()
.
Question to Think About:
What happens if both parent and child define a method with the same name?
Answer: The method in the child class will override the method from the parent class. This is called method overriding, and it's useful when the child needs a different behavior.
Example with Overriding
CLASS Animal
METHOD speak()
PRINT "Animal makes a sound"
CLASS Dog EXTENDS Animal
METHOD speak()
PRINT "Dog barks"
// Create object
pet = NEW Dog()
pet.speak()
Dog barks
This demonstrates that the Dog
class has overridden the speak()
method of its parent Animal
.
Multilevel Inheritance Example
Inheritance can also be extended across multiple levels:
CLASS LivingBeing
METHOD breathe()
PRINT "Breathing..."
CLASS Animal EXTENDS LivingBeing
METHOD move()
PRINT "Animal moves"
CLASS Fish EXTENDS Animal
METHOD swim()
PRINT "Fish swims"
// Create object
fish1 = NEW Fish()
fish1.breathe()
fish1.move()
fish1.swim()
Breathing... Animal moves Fish swims
Points to Remember
- Inheritance allows a child class to use fields and methods of a parent class.
- It avoids code duplication and promotes reuse.
- Method overriding enables child classes to customize behavior.
- Inheritance can be single-level, multilevel, or hierarchical.
Quick Intuition Check
Can a child class access all properties of its parent?
Answer: Yes, except for private properties/methods which are not accessible directly. However, protected and public members are inherited.