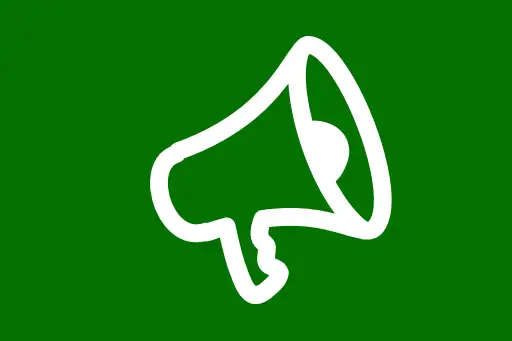
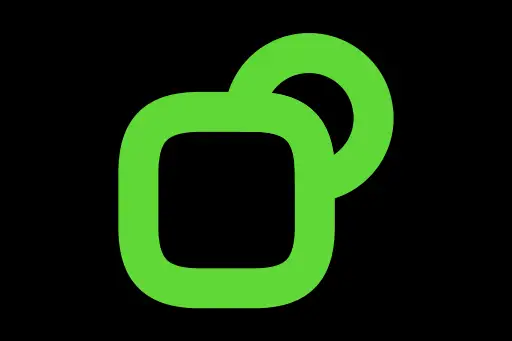



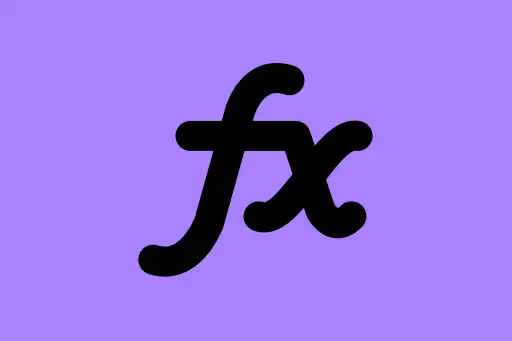


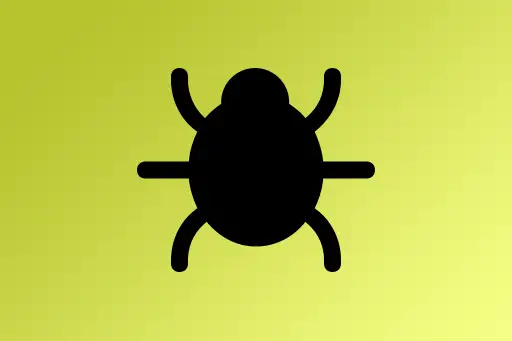


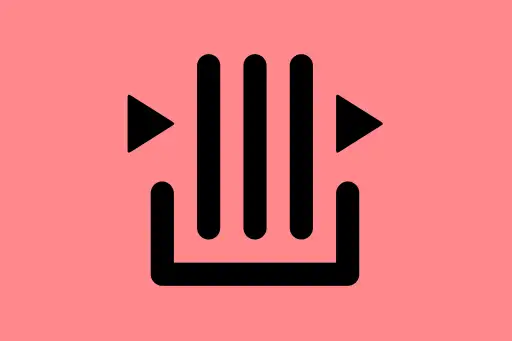

Infinite Loops and Exit Conditions in Programming
What is an Infinite Loop?
An infinite loop is a loop that never terminates unless an external condition forces it to stop. It continues executing endlessly because the loop's exit condition is never met or missing entirely.
Common Causes of Infinite Loops
- Missing or incorrect exit conditions
- Improper variable updates inside the loop
- Always-true conditions like
while (true)
Example 1: A Simple Infinite Loop
This loop will never end because the condition is always true and nothing changes inside the loop.
counter = 0
while counter < 5
print "Hello"
// Forgot to increase counter
Hello Hello Hello ... (infinite)
Fixing the Loop with an Exit Condition
To prevent infinite execution, we must modify the loop variable so that the condition eventually becomes false.
counter = 0
while counter < 5
print "Hello"
counter = counter + 1
Hello Hello Hello Hello Hello
Question:
Why did the previous version of the loop run forever?
Answer:
Because the variable counter
was never updated, so the condition counter < 5
always stayed true.
Example 2: Intentional Infinite Loop with Manual Exit
Sometimes infinite loops are used intentionally, such as in servers or menu-based programs. In these cases, an explicit break condition is added inside the loop.
loop forever
input = read_input()
if input == "exit"
break
print "You entered:", input
You entered: hello You entered: 123 You entered: goodbye ... (loop ends when input is "exit")
Question:
Is using loop forever
always a bad idea?
Answer:
No, it’s valid in cases where you want the loop to continue until an explicit instruction is given (like user input or system signal). But always include a safe exit inside the loop.
Best Practices for Exit Conditions
- Always ensure loop variables change in a way that moves toward stopping the loop
- Test edge cases, like zero or negative input
- If using an infinite loop on purpose, use a
break
orreturn
to exit - Add safety counters or timeouts if applicable
Example 3: Safety Counter for Emergency Exit
To avoid hanging systems, you can include a safety limit to break out of the loop after a certain number of steps.
attempts = 0
max_attempts = 10
loop forever
if condition_is_met()
break
attempts = attempts + 1
if attempts >= max_attempts
print "Breaking due to too many attempts"
break
Breaking due to too many attempts
Wrap-Up
Understanding infinite loops and how to control them is a foundational concept in programming. Whether you're preventing accidental endless loops or building intentional ones, knowing how and when to exit is critical for writing safe and efficient code.