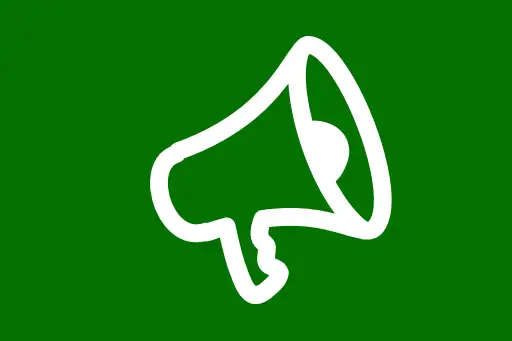
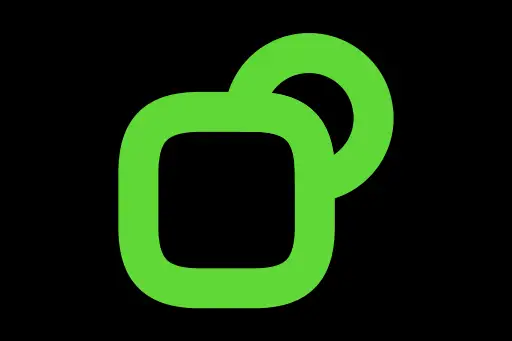



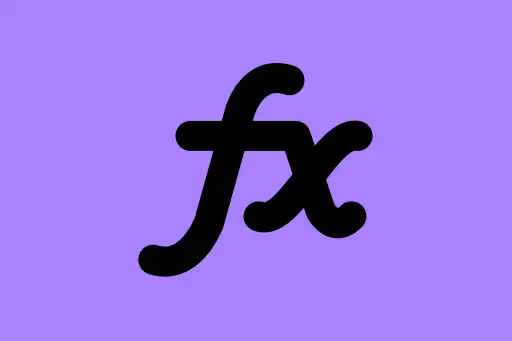


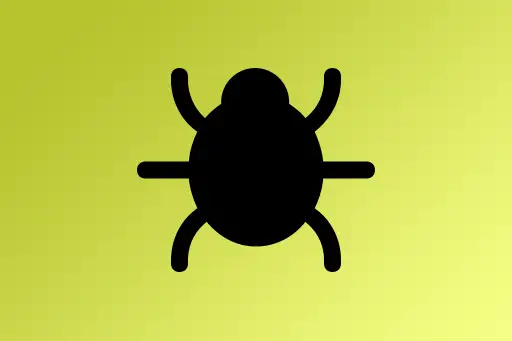


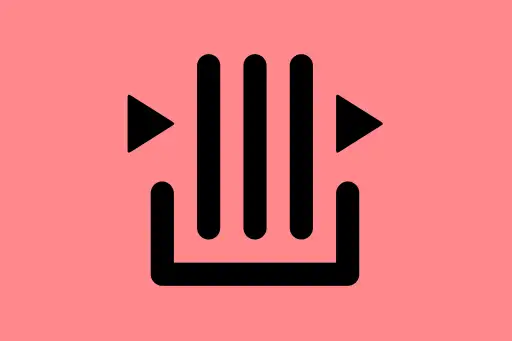

Immutability and Pure Functions
Functional Programming Fundamentals
What is Immutability?
Immutability means that once a value is created, it cannot be changed. Instead of modifying existing data, new data structures are created with the desired changes. This concept helps in making code more predictable, safer, and easier to debug.
Why is Immutability Important?
- It avoids unintended side-effects.
- Makes concurrent programming safer.
- Helps in writing reliable and testable code.
Example: Mutable vs Immutable Behavior
Let’s look at the difference between mutable and immutable behavior using pseudocode.
# Mutable example
list = [1, 2, 3]
append(list, 4)
print(list)
[1, 2, 3, 4]
This directly modifies the original list
. Any other function using list
will now see the changed version, which might cause unexpected behavior.
# Immutable example
list = [1, 2, 3]
newList = list + [4]
print(newList)
print(list)
[1, 2, 3, 4] [1, 2, 3]
Here, the original list
remains unchanged, and a new list newList
is created with the added element.
What are Pure Functions?
A pure function is a function that satisfies two main conditions:
- It always produces the same output for the same input.
- It has no side effects (doesn’t modify global variables, files, or input parameters).
Example: Pure vs Impure Functions
# Impure function
counter = 0
function increment():
counter = counter + 1
return counter
Each call to increment() returns a different value: 1, 2, 3...
This function is impure because it relies on and modifies a variable outside its scope.
# Pure function
function add(a, b):
return a + b
add(2, 3) => 5 add(2, 3) => 5 # always returns the same result
This function is pure. It depends only on its input and doesn’t affect anything outside itself.
Question: Why should we care about pure functions?
Answer: Pure functions make your code predictable and easy to test. They help you build robust systems by minimizing hidden dependencies and side effects.
Question: How does immutability support functional programming?
Answer: Functional programming emphasizes avoiding state changes. Immutability ensures data is not accidentally altered, which fits perfectly with this philosophy and helps create pure functions.
Best Practices
- Avoid changing input parameters inside a function.
- Return new values instead of modifying existing ones.
- Keep functions free of side-effects like logging, modifying files, or accessing global variables.
Conclusion
Immutability and pure functions are at the heart of clean and functional programming. Embracing these principles leads to code that is predictable, easy to test, and bug-resistant. In large systems or team environments, it greatly simplifies reasoning about program behavior.
Comments
Loading comments...