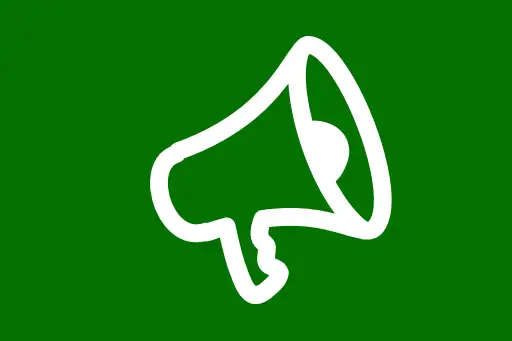
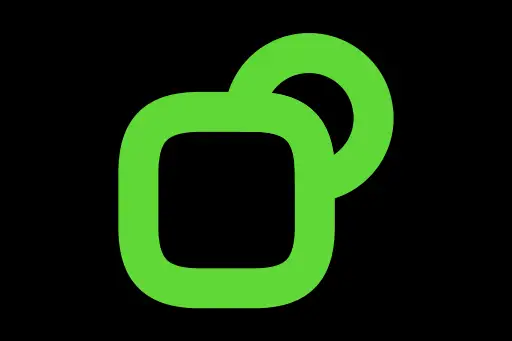



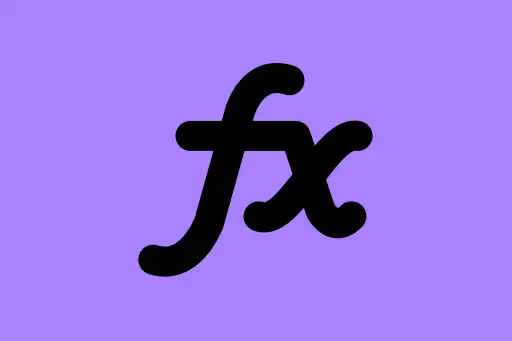


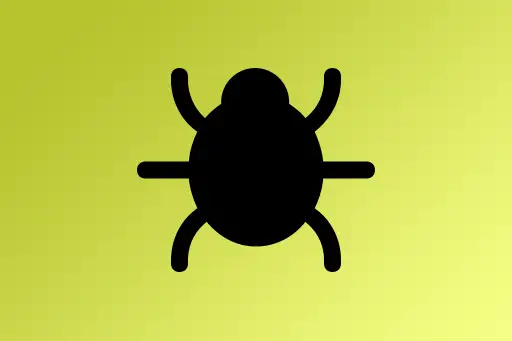


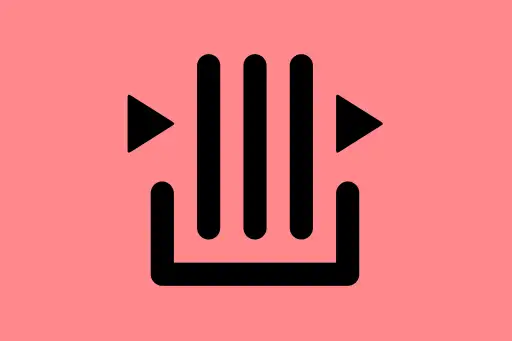

Understanding Dictionaries in Programming
Key-Value Data Structure Explained
What is a Dictionary?
A dictionary is a data structure that stores data in the form of key-value pairs. Each key in the dictionary is unique and is used to access its corresponding value. Think of a dictionary like a real-world glossary or contact list: you look up a word (key) and find its meaning (value).
Why Use Dictionaries?
- Fast lookups using keys.
- Efficient for storing associated data (e.g., ID → Name).
- Dynamic size – can grow as you add entries.
Example 1: Creating a Dictionary
# Create an empty dictionary and add key-value pairs
dictionary = {}
dictionary["name"] = "Alice"
dictionary["age"] = 30
dictionary["city"] = "Wonderland"
print(dictionary)
{"name": "Alice", "age": 30, "city": "Wonderland"}
How does a dictionary store its data?
A dictionary uses a special structure called a hash table internally. This allows it to locate values very quickly using the keys.
Question:
Can dictionary keys be repeated?
Answer: No, keys must be unique. If you assign a new value to an existing key, it will overwrite the previous value.
Example 2: Accessing and Modifying Values
# Access a value using its key
name = dictionary["name"]
print(name)
# Modify an existing value
dictionary["age"] = 31
print(dictionary["age"])
Alice 31
Example 3: Removing Keys
# Remove a key-value pair
remove dictionary["city"]
print(dictionary)
{"name": "Alice", "age": 31}
Question:
What happens if you try to access a key that doesn’t exist?
Answer: It will usually result in an error or a null/undefined value depending on the language or environment. Some implementations allow safe access with default values.
Example 4: Iterating Over a Dictionary
# Iterate over keys and values
for each key in dictionary:
value = dictionary[key]
print(key + ": " + toString(value))
name: Alice age: 31
Use-Cases of Dictionaries
- Storing configuration settings (e.g., {"theme": "dark", "language": "en"})
- Mapping IDs to user data
- Counting occurrences of elements
Question:
Are dictionaries ordered?
Answer: In many modern implementations, yes – items retain the order they were added. But in some older or lower-level systems, order is not guaranteed.
Summary
- Dictionaries store data as key-value pairs.
- They allow fast access and updates using keys.
- Keys must be unique and typically immutable (e.g., strings, numbers).
Practice Challenge
Create a dictionary that stores student names as keys and their test scores as values. Then:
- Update one of the scores
- Remove one student
- Print the final list