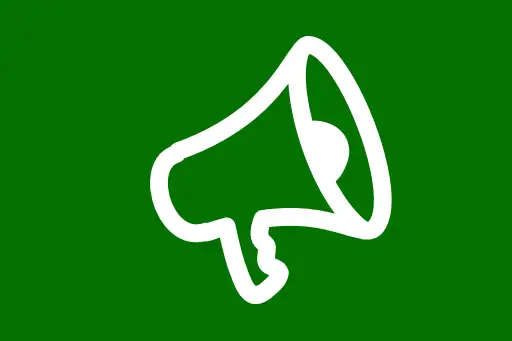
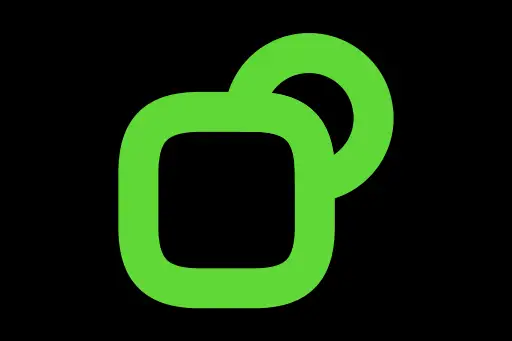



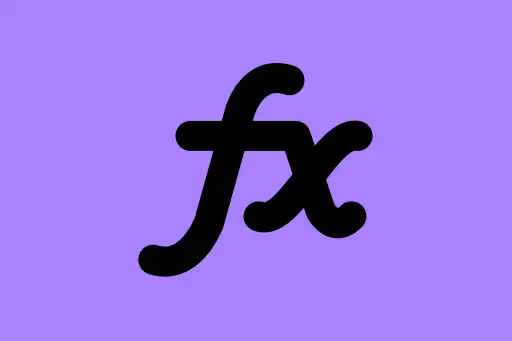


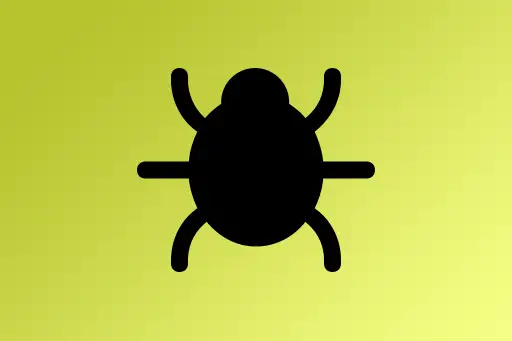


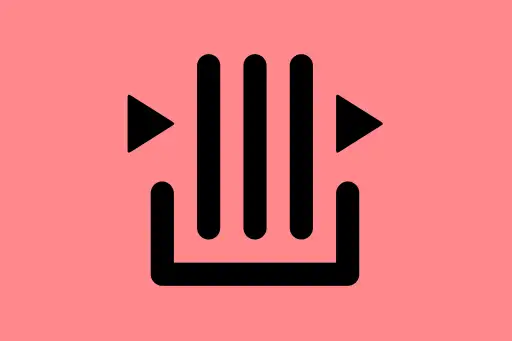

Learn to Debug Your Code
Identify and Fix Errors
Introduction to Debugging
Debugging is the process of identifying, analyzing, and removing bugs or errors in your program. A bug could be anything from a syntax error to an unexpected logical flaw that causes incorrect behavior or results.
Types of Bugs You Might Encounter
- Syntax Errors: Mistakes in code structure or grammar
- Runtime Errors: Errors that crash the program during execution
- Logical Errors: Program runs but returns wrong results
Step-by-Step Debugging Techniques
1. Use Print/Log Statements
One of the simplest ways to debug is by printing intermediate values or messages to track the program’s flow and data state.
function calculateTotal(price, taxRate)
total = price + (price * taxRate)
print("Price:", price)
print("Tax Rate:", taxRate)
print("Total:", total)
return total
end function
Price: 100 Tax Rate: 0.05 Total: 105.0
Question: What should you print when a program behaves unexpectedly?
Answer: Start by printing values of inputs, outputs, and intermediate variables that influence your logic path.
2. Trace the Execution Path
If your program has conditions and loops, follow the execution flow to understand which blocks are being executed and why.
function checkEligibility(age)
if age > 18
print("User is eligible")
else
print("User is not eligible")
end if
end function
Tip: Add a print before each decision point to confirm the path being taken.
3. Simplify the Problem
If your codebase is large, try to isolate the problematic part. Create a smaller version (minimal reproducible example) to test your assumptions.
# Full function is long and hard to debug. Let's isolate part of it.
function sumFirstN(n)
sum = 0
for i from 1 to n
sum = sum + i
end for
return sum
end function
Question: Why is isolating code helpful?
Answer: It reduces distractions and makes it easier to pinpoint errors in a focused section.
4. Use Assertions
Assertions help verify assumptions in your code. If the condition fails, the program stops and alerts you.
function divide(a, b)
assert(b != 0, "Divider must not be zero")
return a / b
end function
Assertion failed: Divider must not be zero
5. Walk Through the Code Manually
Read your code line by line and simulate it on paper or a whiteboard. Note down variable values at each step.
6. Rubber Duck Debugging
Explain your code to someone else—or to a rubber duck! Speaking your logic aloud often reveals overlooked errors.
Tips for Debugging
- Always read the error message carefully—it usually tells you where the problem is.
- Work from the top of the stack trace downward.
- Check for common issues: typos, off-by-one errors, missing return statements.
- Test your code incrementally after every small change.
Summary
Debugging is a skill that improves with practice. It’s not just about fixing errors, but about understanding your code better. Use a combination of techniques—logging, tracing, assertions, and isolation—to solve problems efficiently.
In the next lesson, we’ll explore Custom Exceptions and how to create error messages that make debugging even easier.