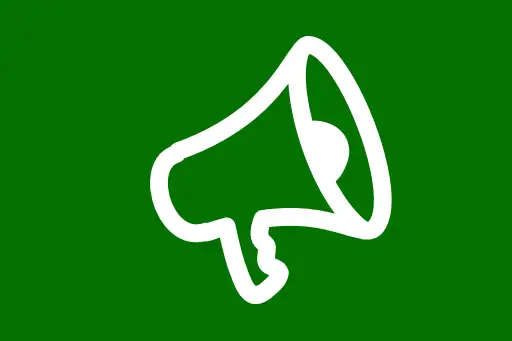
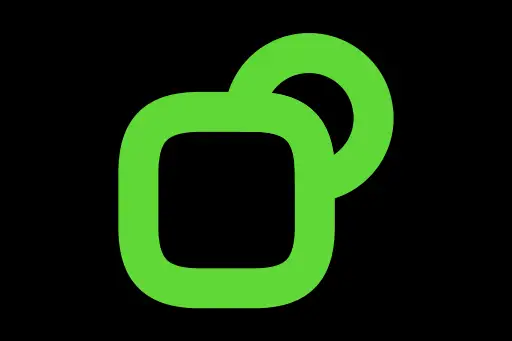



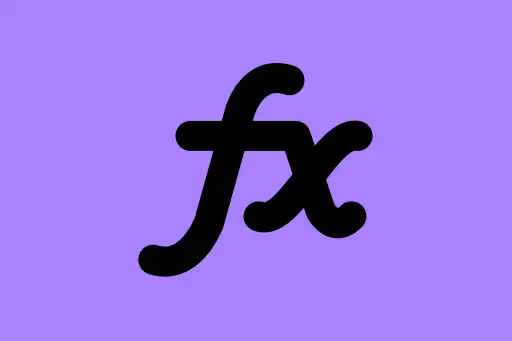


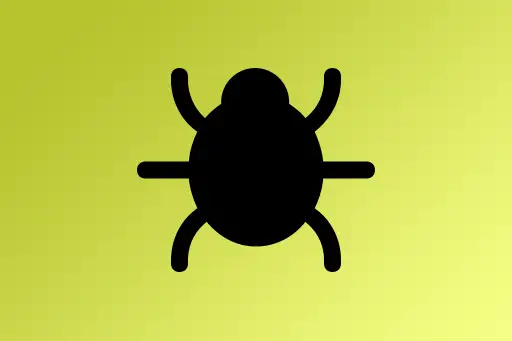


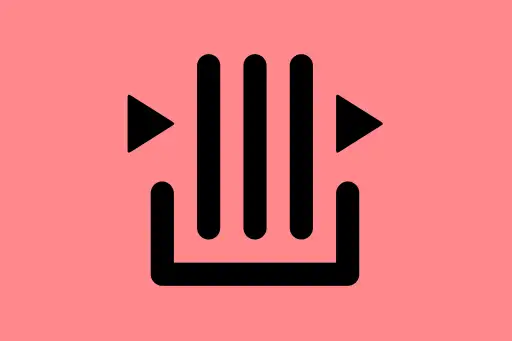

Understanding Complex Data Types in Programming
Arrays, Lists, Sets, Dictionaries
What are Complex Data Types?
Complex data types are used to store multiple values in a single variable. Unlike primitive data types (like integer, float, or boolean), which hold a single value, complex data types can hold collections of values and offer built-in ways to organize, access, and manipulate them efficiently.
Why Use Complex Data Types?
- To manage groups of data with a single identifier.
- To perform operations like sorting, searching, filtering, and aggregating on collections of items.
- To better structure data in real-world applications (like user profiles, orders, etc.).
Types of Complex Data Structures
1. Arrays / Lists
An array (or list) is a collection of elements stored in a sequence. Each item can be accessed by its index.
// Define a list of numbers
numbers = [10, 20, 30, 40]
// Access elements
print(numbers[0]) // First element
print(numbers[3]) // Last element
// Modify elements
numbers[1] = 25
// Add a new element at the end
append(numbers, 50)
// Loop through the list
for num in numbers:
print(num)
10 40 10 25 30 40 50
Question:
What happens if you try to access an index that doesn’t exist?
Answer:
You get an error or "out of bounds" exception depending on the programming environment. Always ensure the index is valid (between 0 and length - 1).
2. Sets
A set is a collection of unique, unordered elements. Useful for checking membership and removing duplicates.
// Define a set
unique_numbers = set(10, 20, 20, 30, 10)
// Add elements
add(unique_numbers, 40)
// Check membership
if 20 in unique_numbers:
print("20 is present")
// Loop through set
for item in unique_numbers:
print(item)
20 is present 10 20 30 40
Question:
Why might you use a set instead of a list?
Answer:
If you only care about unique items and fast membership checking, sets are more efficient than lists.
3. Dictionaries / Maps
Dictionaries (or maps) store data as key-value pairs. Each key is unique, and you use keys to access corresponding values.
// Create a dictionary to store student scores
scores = {
"Alice": 90,
"Bob": 85,
"Charlie": 92
}
// Access a value by key
print(scores["Alice"])
// Add a new key-value pair
scores["David"] = 88
// Modify a value
scores["Bob"] = 87
// Loop through dictionary
for key in scores:
print(key, scores[key])
90 Alice 90 Bob 87 Charlie 92 David 88
Question:
What is a good real-world use case for a dictionary?
Answer:
Storing student grades, user profiles (name, email, age), or configuration settings where you retrieve data using descriptive keys.
Comparing Complex Data Types
Type | Ordered? | Allows Duplicates? | Access Method | Common Use |
---|---|---|---|---|
List / Array | Yes | Yes | By index | Storing sequence of items |
Set | No | No | Membership test | Unique items, filtering |
Dictionary / Map | No | Keys: No, Values: Yes | By key | Key-value storage |
Summary
- Lists are good for ordered, index-based access.
- Sets help eliminate duplicates and check presence efficiently.
- Dictionaries are ideal when data is naturally paired (like name → value).
Quick Practice
// Practice Task:
// Create a dictionary with city names as keys and population as values.
// Print all cities with a population over 1 million.
cities = {
"Metropolis": 1500000,
"Smallville": 450000,
"Star City": 1200000
}
for city in cities:
if cities[city] > 1000000:
print(city)
Metropolis Star City