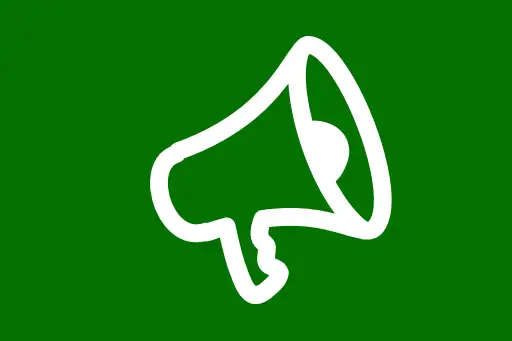
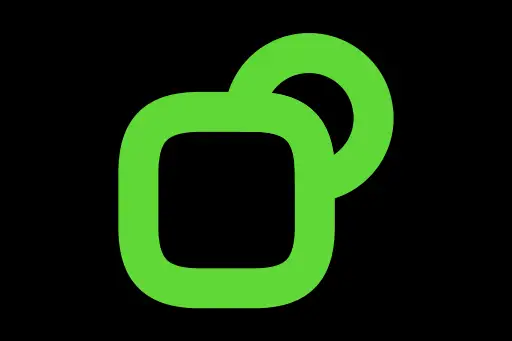



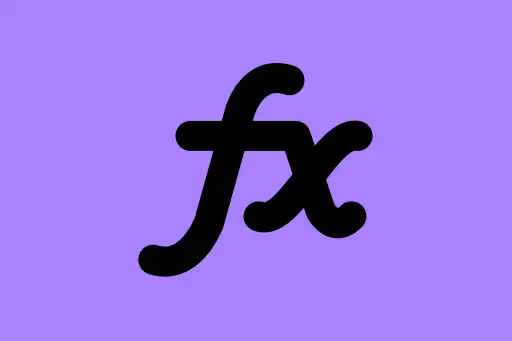


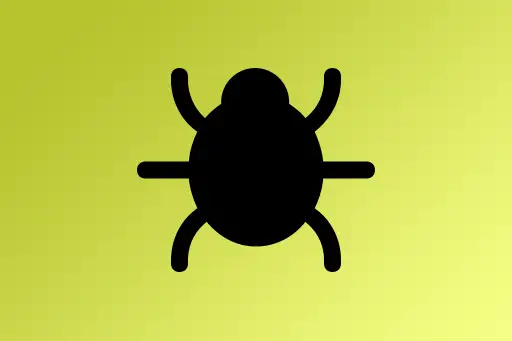


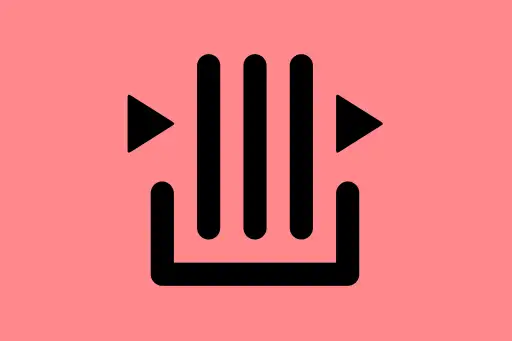

Understanding Comparison Operators in Programming
What Are Comparison Operators?
Comparison operators are used to compare two values or expressions. They always return a boolean value: either true
or false
. These operators are essential in decision-making logic like conditions and loops.
List of Common Comparison Operators
==
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
Example 1: Using ==
(Equal To)
SET a TO 5
SET b TO 5
IF a == b THEN
PRINT "a and b are equal"
ELSE
PRINT "a and b are not equal"
END IF
a and b are equal
Question: What happens if a
was 5 and b
was 10?
Answer: The result would be false
, so it would print "a and b are not equal"
.
Example 2: Using !=
(Not Equal To)
SET x TO 10
SET y TO 20
IF x != y THEN
PRINT "x and y are different"
ELSE
PRINT "x and y are the same"
END IF
x and y are different
Example 3: Using >
and <
SET age TO 18
IF age > 21 THEN
PRINT "You are allowed entry"
ELSE
PRINT "Entry denied"
END IF
Entry denied
Question: Why was entry denied even though age is 18?
Answer: Because the condition checks if age > 21
, and 18 is not greater than 21.
Example 4: Using >=
and <=
SET marks TO 75
IF marks >= 60 THEN
PRINT "You passed"
ELSE
PRINT "You failed"
END IF
You passed
Tip: Use >=
when you want to include the boundary value as valid. In this case, even 60 would result in "You passed".
When Are Comparison Operators Used?
They are primarily used in:
- Conditional statements like IF, ELSE IF
- Loops to repeat code until a condition is false
- Filtering or searching through data
Summary
- Comparison operators return either
true
orfalse
. - They are essential for decision-making in code.
- Always check whether the operator you use includes the boundary (like
>=
vs>
).
Understanding how these operators work is important before diving into more advanced topics like loops, functions, or algorithms.