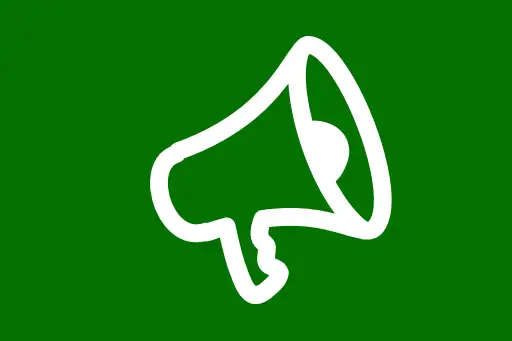
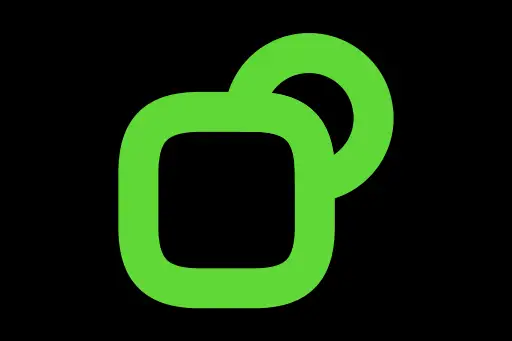



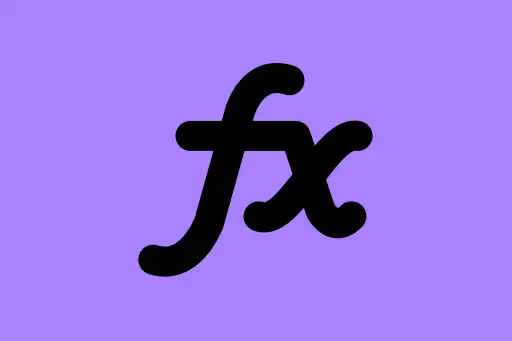


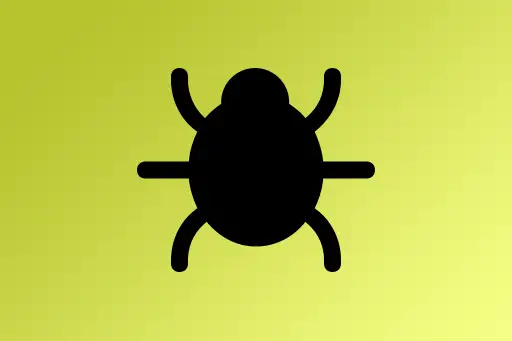


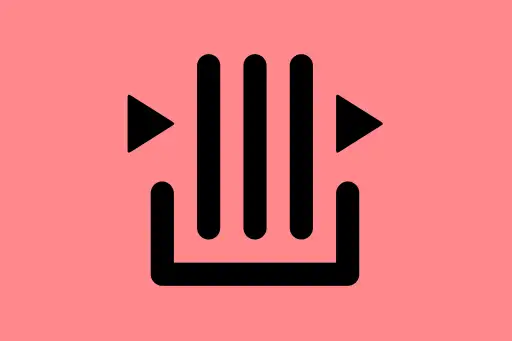

Understanding Classes and Objects in Programming
OOP Basics Explained
What are Classes and Objects?
In programming, a class is a blueprint or template that defines the structure and behavior (data and functions) of objects. An object is an actual instance of a class—just like a real-world object with properties and actions.
Imagine a class as a recipe and an object as the actual dish prepared from that recipe.
Real-World Analogy
Consider a "Car" as a class. All cars have certain properties like color, model, and engine type. They also have behaviors like drive, brake, and honk.
- The Car class defines these common properties and actions.
- Your individual car, say a red sedan, is an object (an instance) of that class.
Why Do We Use Classes and Objects?
- To model real-world entities in code.
- To group related data and functions together.
- To reuse code efficiently (you can create many objects from one class).
Basic Class and Object Structure
class Car:
attribute color
attribute model
method drive():
print "The car is driving."
method brake():
print "The car has stopped."
# Creating an object
myCar = new Car()
myCar.color = "Red"
myCar.model = "Sedan"
myCar.drive()
myCar.brake()
The car is driving. The car has stopped.
Explanation of the Code
class Car
defines a blueprint for cars.attribute
defines the properties every car has.method
represents actions the car can perform.myCar
is an instance (object) created from theCar
class.- We assign values to its attributes and call its methods.
Question: What happens if you create another car object?
Answer: You can create as many car objects as needed, each with its own unique state (color, model, etc.), but all sharing the same behavior (methods).
Another Example: Class for a Student
Let’s consider modeling a student:
class Student:
attribute name
attribute grade
method introduce():
print "Hi, my name is " + name + " and I am in grade " + grade
# Creating objects
student1 = new Student()
student1.name = "Alice"
student1.grade = "10"
student2 = new Student()
student2.name = "Bob"
student2.grade = "12"
student1.introduce()
student2.introduce()
Hi, my name is Alice and I am in grade 10 Hi, my name is Bob and I am in grade 12
Beginner Intuition Check
Q: Can two objects have different values for the same attribute?
A: Yes, even though both are instances of the same class, their properties can have different values.
Key Takeaways
- A class defines a template; an object is an instance of that template.
- Classes help organize code and model real-life entities with attributes and behaviors.
- Objects created from the same class can have different values but share the same structure.
Practice Questions
- What’s the difference between a class and an object?
- How can you create multiple objects from the same class?
- Can objects share methods but have different attribute values?
Next Up
We’ll learn about constructors, the special methods used to initialize objects with default values upon creation.