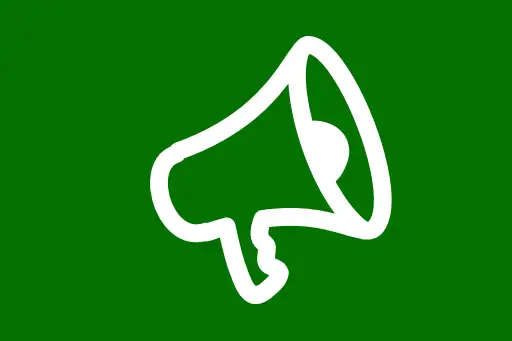
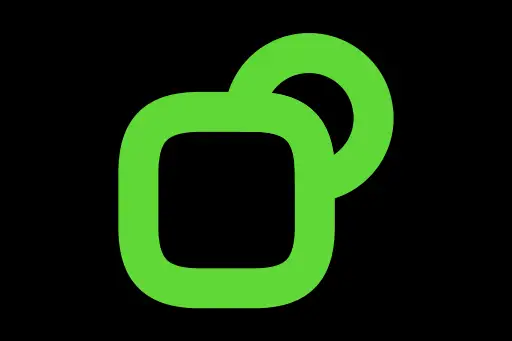



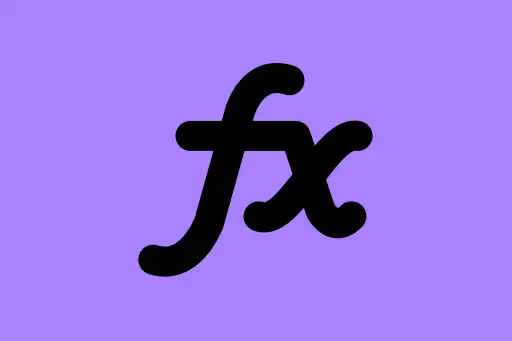


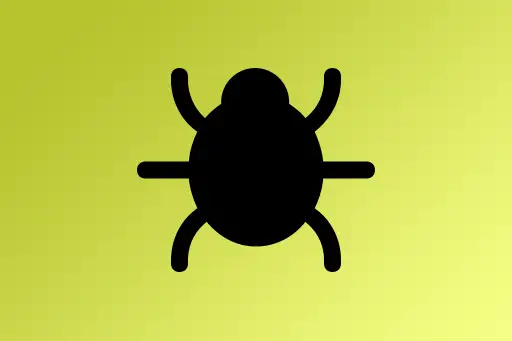


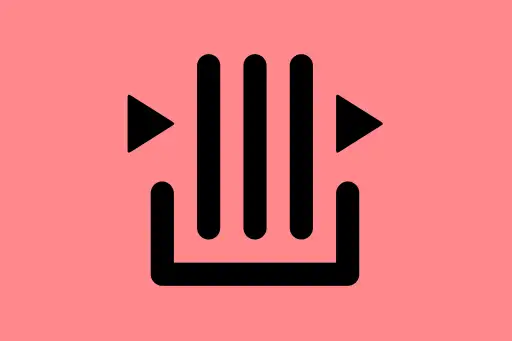

Understanding Arithmetic Operators in Programming
What are Arithmetic Operators?
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, and division. They form the building blocks of any numerical computation in programming.
List of Common Arithmetic Operators
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulo (Remainder)
Example 1: Basic Arithmetic Operations
Let’s take two numbers and apply all the arithmetic operators to understand their behavior.
START
SET a = 10
SET b = 3
PRINT "Addition: " + (a + b)
PRINT "Subtraction: " + (a - b)
PRINT "Multiplication: " + (a * b)
PRINT "Division: " + (a / b)
PRINT "Modulo: " + (a % b)
END
Addition: 13 Subtraction: 7 Multiplication: 30 Division: 3 Modulo: 1
Question: Why does division of 10 by 3 give 3 instead of 3.33?
Answer: In many programming environments, when both operands are whole numbers (integers), the division operation returns only the integer part (i.e., floor division). This is called integer division. If decimal accuracy is needed, you must explicitly convert operands to a floating-point type.
Example 2: Working with Negative Numbers
Let’s see how subtraction and modulo behave with negative numbers.
START
SET x = -15
SET y = 4
PRINT "Subtraction: " + (x - y)
PRINT "Modulo: " + (x % y)
END
Subtraction: -19 Modulo: -3
Question: Why is -15 % 4 equal to -3?
Answer: In some languages or environments, the modulo operation keeps the sign of the dividend (first number). Hence, -15 % 4 gives -3. Always check how your programming language implements modulo.
Example 3: Using Arithmetic Operators to Swap Values
Can you swap two numbers without using a temporary variable?
START
SET a = 5
SET b = 7
a = a + b // a becomes 12
b = a - b // b becomes 5
a = a - b // a becomes 7
PRINT a, b
END
a = 7, b = 5
Why does this trick work?
Answer: This works due to the reversible nature of addition and subtraction. By using these operators carefully, you can store and recover both original values without any extra space.
Example 4: Applying Arithmetic in Real Life
Let’s calculate the average of 3 scores:
START
SET math = 80
SET science = 90
SET english = 85
SET total = math + science + english
SET average = total / 3
PRINT "Total: " + total
PRINT "Average: " + average
END
Total: 255 Average: 85
Key Takeaways
- Arithmetic operators are fundamental to all mathematical computations.
- Division between integers may discard the decimal part.
- Modulo is useful for checking divisibility, wrapping around values (like in clocks), and more.
- Be aware of operator behavior with negative numbers—it varies by implementation.