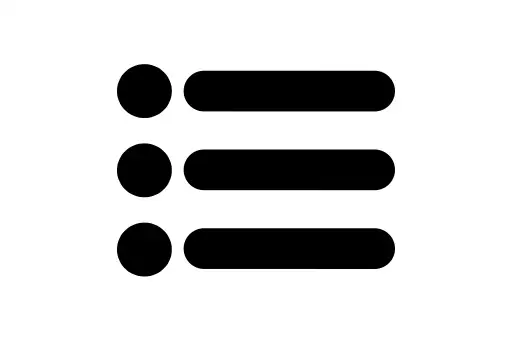
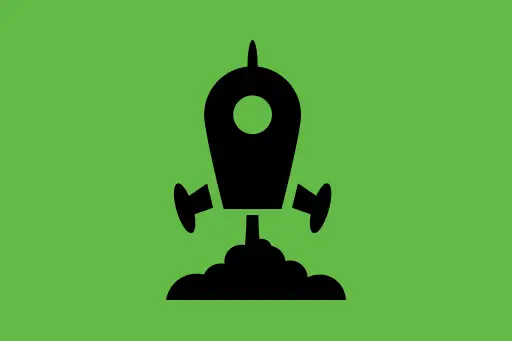
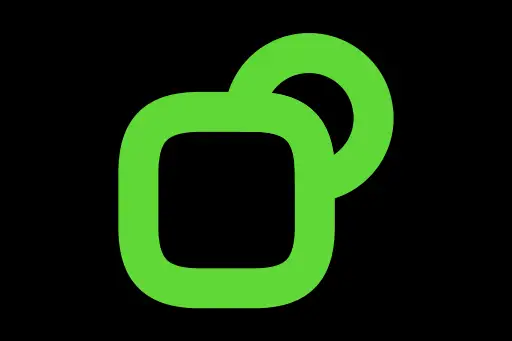
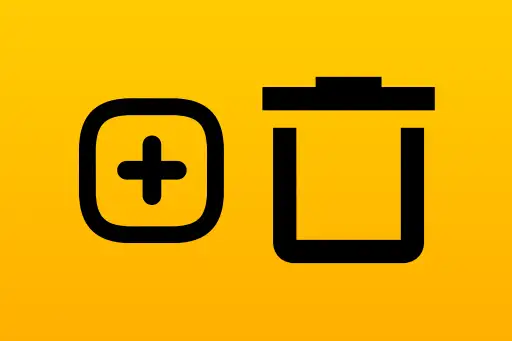
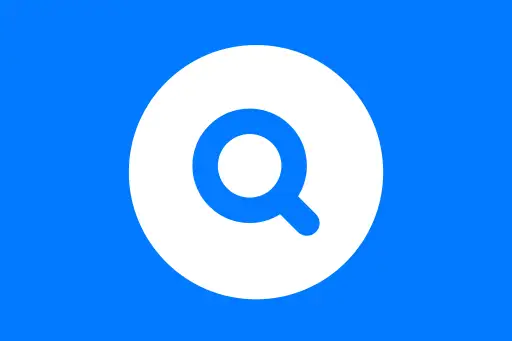
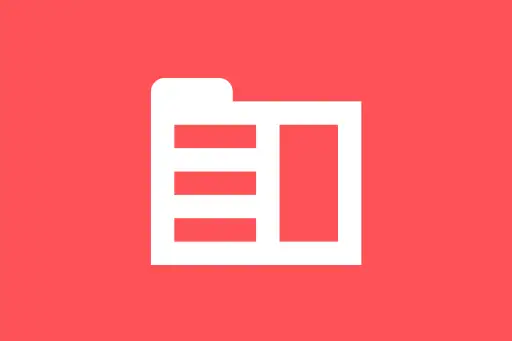
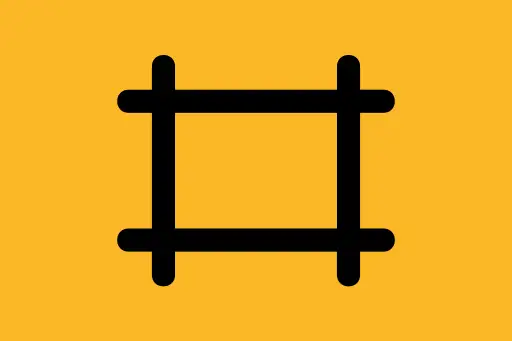
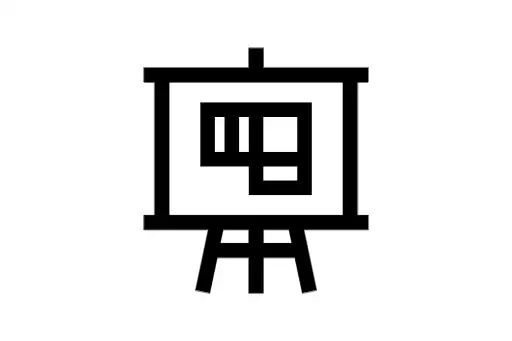
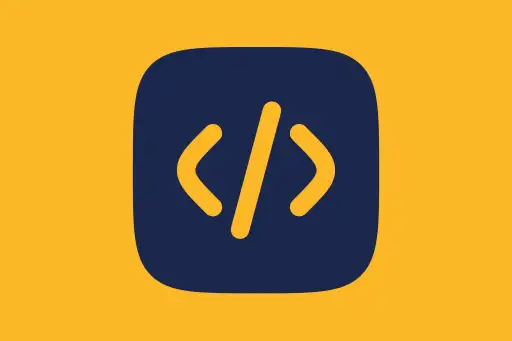
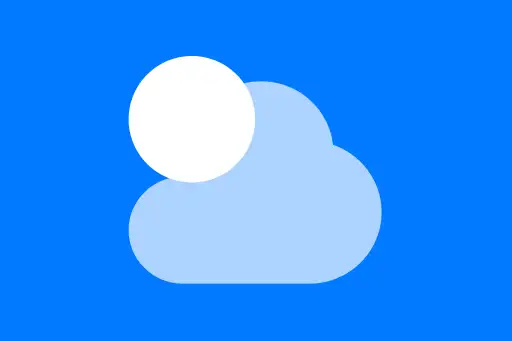
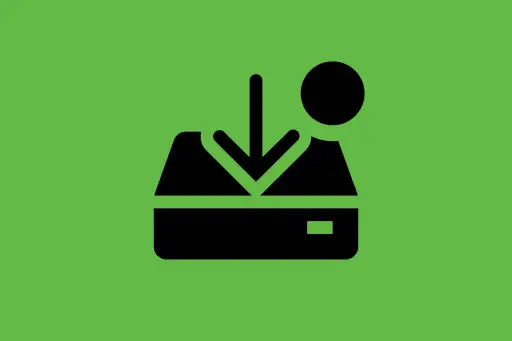
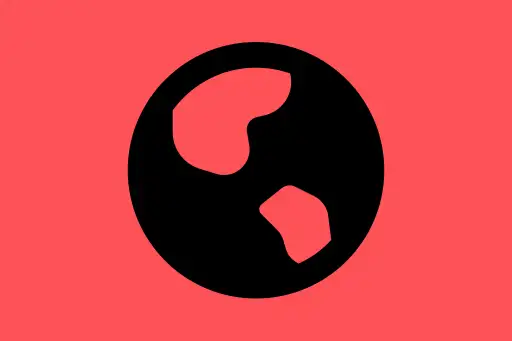
MongoDB Use-cases and Features Overview
MongoDB is a popular NoSQL database known for its flexibility, scalability, and ease of use. In this lesson, we'll explore where and why MongoDB is used in real-world scenarios and what features make it stand out for modern applications.
Use-case 1: Content Management Systems (CMS)
In a CMS, different types of content (articles, videos, events) may have different fields. Traditional SQL databases struggle with varying schemas, while MongoDB easily handles this with dynamic schemas.
db.contents.insertMany([
{ type: "article", title: "Learn MongoDB", author: "John", tags: ["mongodb", "nosql"] },
{ type: "video", title: "Intro to NoSQL", duration: "12min", url: "https://example.com" },
{ type: "event", title: "MongoDB Webinar", date: "2025-06-01", location: "Online" }
]);
{ acknowledged: true, insertedIds: [ObjectId("..."), ObjectId("..."), ObjectId("...")] }
Explanation: Each document has a different structure depending on the content type. MongoDB doesn’t enforce any predefined schema, making it ideal for such flexible use-cases.
Q: How would you store these different types of content in SQL?
A: You’d need separate tables or a wide table with many nullable fields. In MongoDB, this complexity is avoided.
Use-case 2: E-commerce Applications
MongoDB is great for storing product catalogs, customer data, and orders with embedded structures. Each product may have a different set of specifications, sizes, or colors.
db.products.insertOne({
name: "Smartphone",
price: 35000,
specs: {
brand: "TechBrand",
model: "X100",
battery: "4000mAh",
colors: ["black", "blue", "silver"]
}
});
{ acknowledged: true, insertedId: ObjectId("...") }
Explanation: The embedded specs
object holds structured data directly within the product document. No joins or separate tables are required.
Use-case 3: Real-time Analytics
MongoDB supports real-time analytics with its powerful aggregation framework. For example, counting user logins per day can be done with a simple pipeline.
db.logins.aggregate([
{ $match: { date: { $gte: ISODate("2025-04-01") } } },
{ $group: { _id: "$date", total_logins: { $sum: 1 } } },
{ $sort: { _id: 1 } }
]);
[ { _id: ISODate("2025-04-01"), total_logins: 120 }, { _id: ISODate("2025-04-02"), total_logins: 98 }, ... ]
Explanation: This example shows how MongoDB’s aggregation pipeline lets you group and count documents efficiently, making it suitable for dashboards and analytics systems.
Use-case 4: Mobile Applications
Mobile apps need to sync and store user data quickly. MongoDB's flexible schema and offline-first syncing (via MongoDB Realm) make it a natural choice.
Example: Saving a user's profile with varying optional fields like preferences, avatars, and activity logs.
db.users.insertOne({
username: "mob_user01",
preferences: {
theme: "dark",
notifications: true
},
activity_log: [
{ action: "login", timestamp: ISODate("2025-05-01T09:00:00Z") },
{ action: "purchase", item: "eBook", timestamp: ISODate("2025-05-01T10:15:00Z") }
]
});
{ acknowledged: true, insertedId: ObjectId("...") }
Explanation: The document combines nested objects and arrays, ideal for capturing complex mobile user data without joins.
Core Features of MongoDB
- Flexible Schema: Store varied documents without redefining structure.
- Scalable Architecture: Easily scale horizontally with sharding.
- High Availability: Built-in replication and failover with replica sets.
- Powerful Aggregation: Use pipelines to transform and analyze data.
- Indexing Support: Index any field to improve read performance.
- Geospatial Queries: Ideal for location-based services (e.g., maps, delivery apps).
Quick Question
Q: What happens if you try to insert a document with fields not present in other documents?
A: MongoDB allows it! That’s the benefit of having a dynamic schema. It won’t throw errors or require table alteration.
Summary
MongoDB is used in many real-world applications such as content platforms, e-commerce, analytics, mobile apps, and more. Its flexible document model, scalability, and advanced features like aggregation make it a go-to database for modern developers.
In the next lesson, we’ll set up MongoDB on your local machine so you can start creating your own documents and collections.