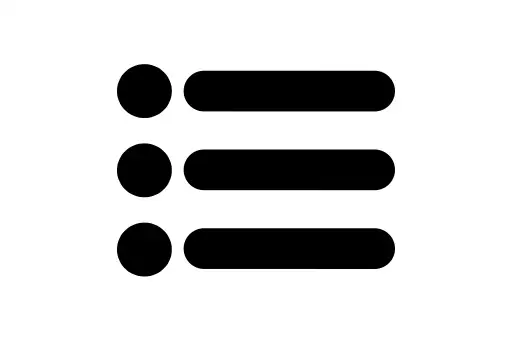
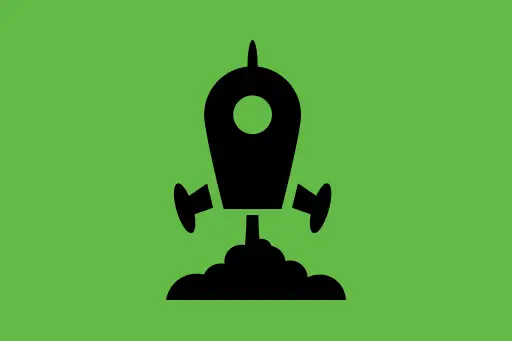
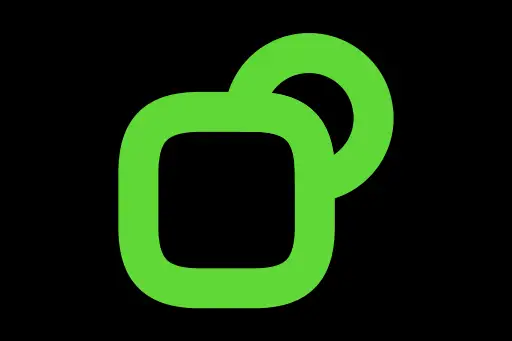
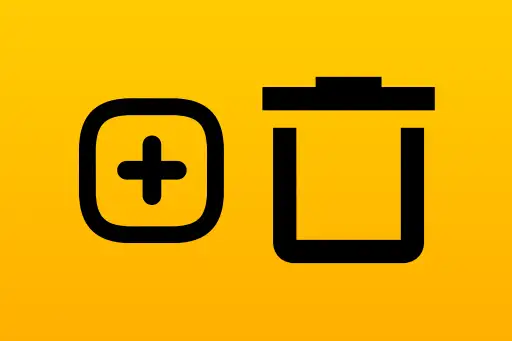
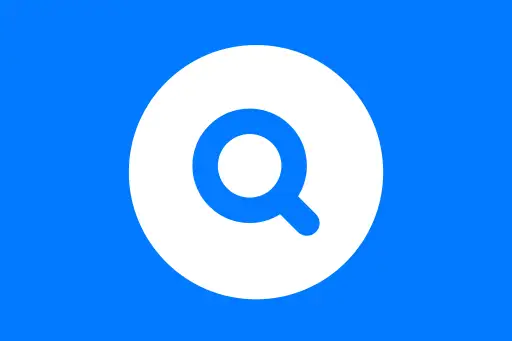
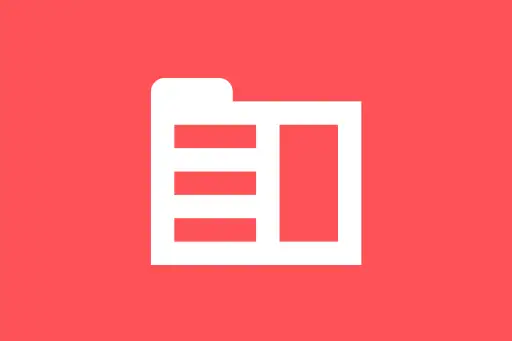
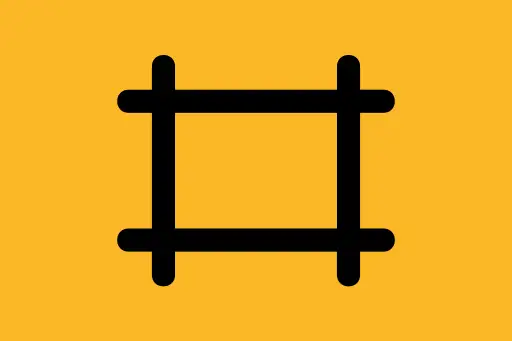
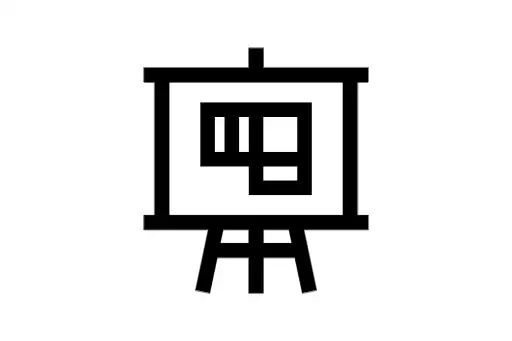
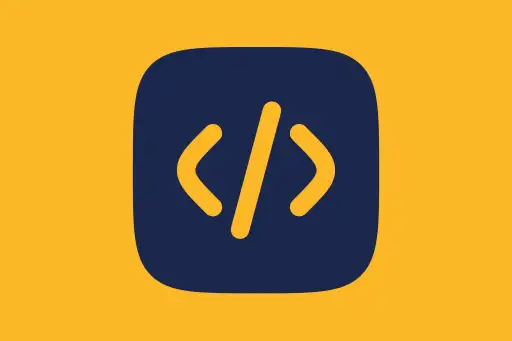
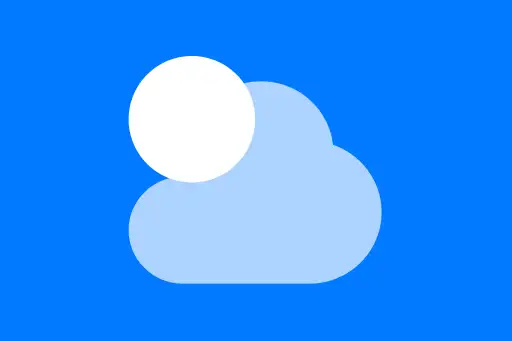
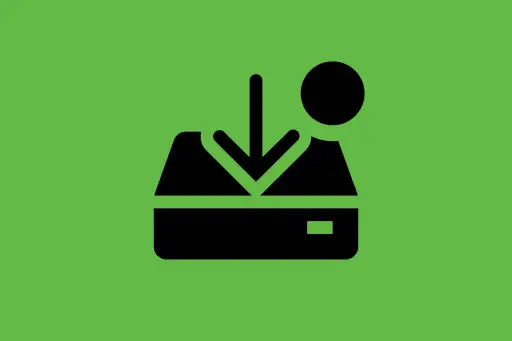
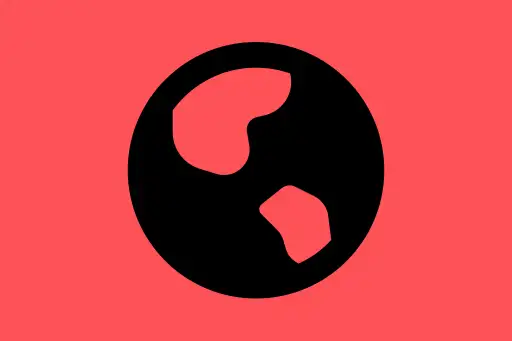
Setting Up a Free MongoDB Atlas Cluster
Next Topic ⮕Creating and Managing Databases in Atlas
Setting Up a Free MongoDB Atlas Cluster
MongoDB Atlas is the official cloud database service for MongoDB. It offers a free tier cluster that you can use to learn and develop applications without setting up MongoDB on your local system.
With Atlas, your database is hosted in the cloud, and you can connect to it from anywhere using a connection string.
Step 1: Create a MongoDB Atlas Account
Go to mongodb.com/cloud/atlas and sign up for a free account. You can use your email or sign in with Google or GitHub.
Question: Why use MongoDB Atlas instead of installing MongoDB locally?
Answer: Atlas lets you skip installation and manage your database from any machine. It also offers cloud backups, monitoring, and easy scaling—all for free in the basic tier.
Step 2: Create a New Project
- After logging in, click on “New Project”.
- Give your project a name like
MyFirstProject
. - Click “Next” → “Create Project”.
Step 3: Build a Cluster
- Click on “Build a Cluster” under your project.
- Choose the free tier: Shared Clusters →
M0
. - Select a cloud provider (AWS, Azure, GCP) and a region near you.
- Name your cluster (e.g.,
Cluster0
). - Click “Create Cluster”.
It may take 1–3 minutes to deploy your free cluster.
Step 4: Create a Database User
- Go to Database Access from the left sidebar.
- Click “Add New Database User”.
- Set a username and password (e.g.,
mongoUser
,mongoPass123
). - Choose Read and Write to Any Database.
- Click Add User.
Step 5: Whitelist Your IP Address
- Go to Network Access.
- Click “Add IP Address”.
- Choose “Allow access from anywhere” (adds
0.0.0.0/0
). - Click Confirm.
Note: You can restrict this later for better security.
Step 6: Connect to Your Cluster
- Click on Clusters → Connect.
- Choose “Connect Your Application”.
- Select driver:
Node.js
(or any language) - Copy the connection string (e.g.,
mongodb+srv://...
).
Example MongoDB connection string:
mongodb+srv://mongoUser:mongoPass123@cluster0.asdf.mongodb.net/test?retryWrites=true&w=majority
Question: What is the retryWrites=true&w=majority
part?
Answer: It's an option to enable retrying writes automatically and writing to the majority of nodes for consistency in the cluster.
Step 7: Connect with Mongo Shell
You can also connect using the command-line shell:
mongo "mongodb+srv://cluster0.asdf.mongodb.net/test" --username mongoUser
Enter password: ******** MongoDB shell version v5.x.x connecting to: mongodb+srv://cluster0.asdf.mongodb.net/test >
Now you're connected to your remote Atlas cluster using the Mongo shell!
Step 8: Insert a Sample Document
use testDatabase
db.students.insertOne({
name: "Arjun",
course: "MongoDB Basics",
enrolled: true
});
{ acknowledged: true, insertedId: ObjectId("...") }
This document is now saved in the cloud, in your MongoDB Atlas database.
Summary
- You created a free MongoDB Atlas cluster.
- Created a database user and whitelisted IPs.
- Connected using shell and inserted a sample document.
This setup is ideal for working on MongoDB projects from anywhere, without worrying about local configuration or server uptime.
Next Step
Let’s now explore how to connect your MongoDB Atlas database to code — using Node.js or Python to perform CRUD operations!