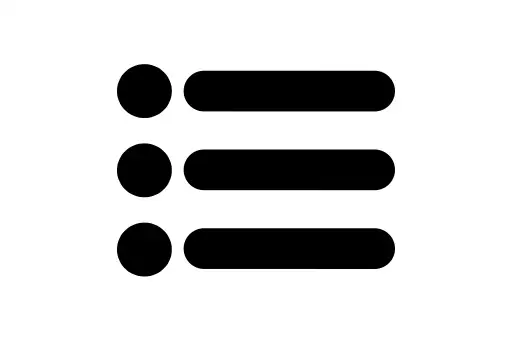
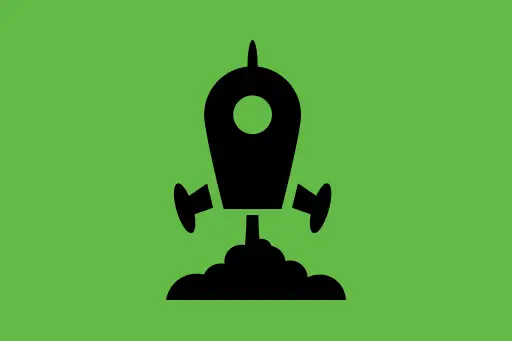
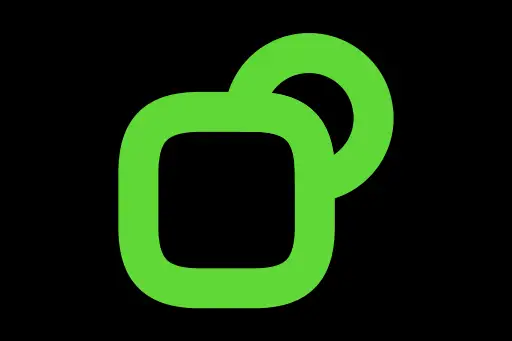
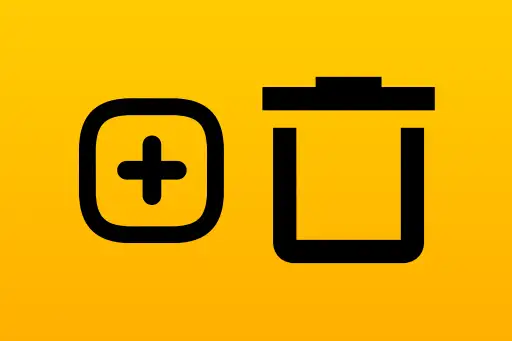
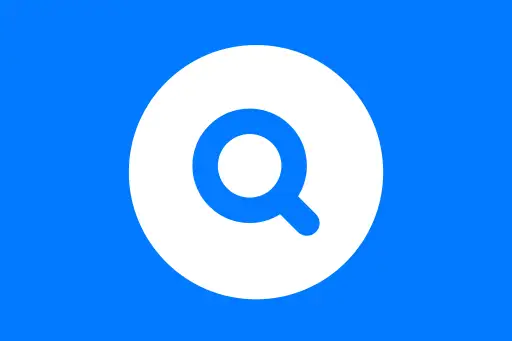
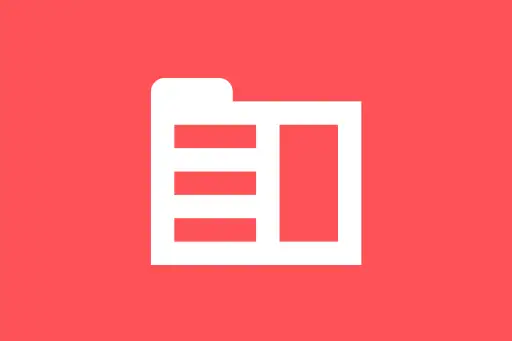
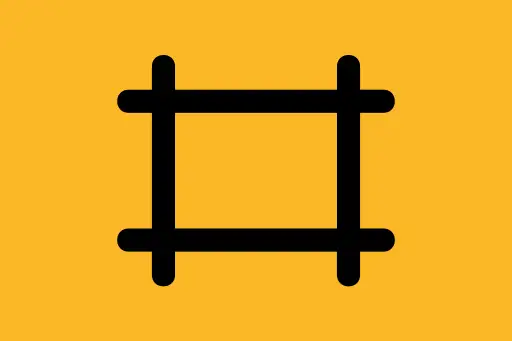
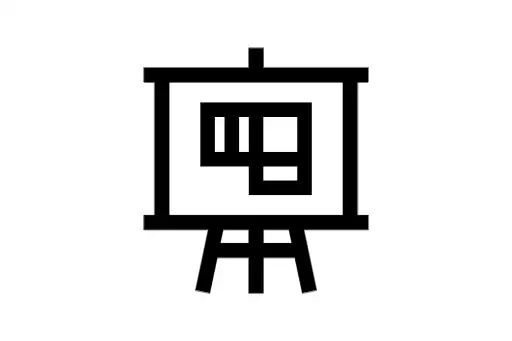
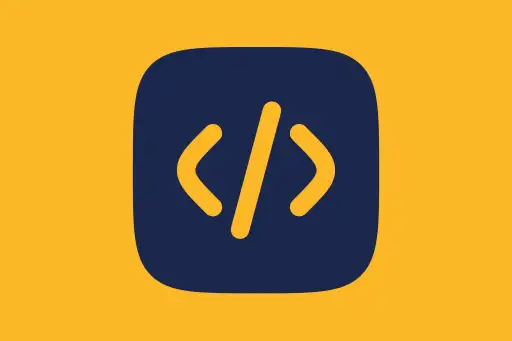
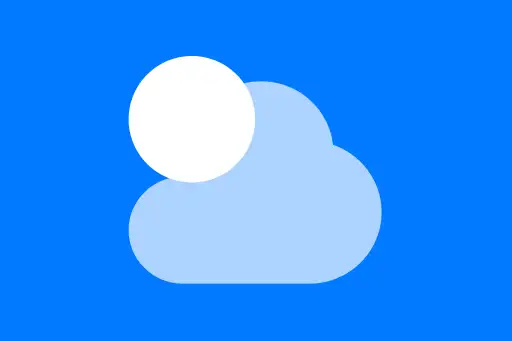
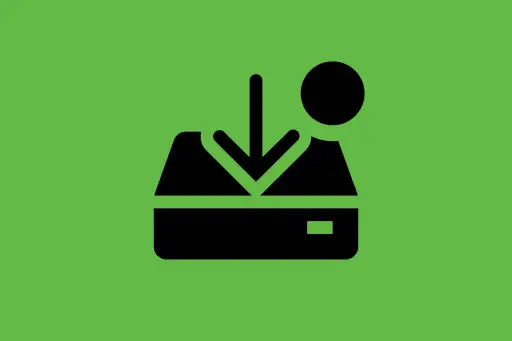
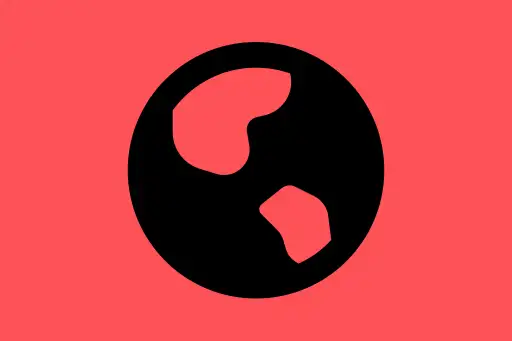
Basic MongoDB Security Best Practices
Next Topic ⮕Project 1: Task Tracker with Node.js + MongoDB
Basic MongoDB Security Best Practices
MongoDB is powerful, but with great power comes great responsibility. Out-of-the-box, MongoDB has minimal security enabled, especially in development environments. This tutorial covers essential practices to help you secure your MongoDB databases effectively.
Enable Authentication
By default, MongoDB does not enforce authentication, meaning anyone can access the database. This is risky, especially in production.
To enable authentication, modify the MongoDB configuration file mongod.conf
:
sudo nano /etc/mongod.conf
Locate or add the following lines:
security:
authorization: enabled
Restart MongoDB to apply the change:
sudo systemctl restart mongod
Create an Admin User
After enabling authentication, you need to create a user with administrative privileges to manage the database:
use admin
db.createUser({
user: "adminUser",
pwd: "StrongPassword123",
roles: [{ role: "userAdminAnyDatabase", db: "admin" }]
});
Successfully added user: { "user": "adminUser", "roles": [ { "role": "userAdminAnyDatabase", "db": "admin" } ] }
Q: What happens if you restart MongoDB after enabling auth but don’t create a user first?
A: You’ll lock yourself out! Always create the admin user before enabling authentication in production.
Use Role-Based Access Control (RBAC)
Never give all users admin privileges. MongoDB supports built-in roles like:
read
– read-only accessreadWrite
– read and writedbAdmin
– database-level adminuserAdmin
– user management
Create a user for a specific database:
use myapp
db.createUser({
user: "appUser",
pwd: "AppPassword456",
roles: [{ role: "readWrite", db: "myapp" }]
});
Successfully added user: { "user": "appUser", "roles": [ { "role": "readWrite", "db": "myapp" } ] }
Q: Why is it dangerous to use one admin user for both development and production?
A: It violates the principle of least privilege. If compromised, the attacker gets full access to everything.
Bind to Localhost or Specific IP
By default, MongoDB may bind to all IP addresses, making it open to external access. You can limit access using the bindIp
setting.
net:
bindIp: 127.0.0.1
Or allow a specific IP (e.g., your app server):
net:
bindIp: 127.0.0.1,192.168.1.100
Restart MongoDB to apply:
sudo systemctl restart mongod
Q: If MongoDB listens on 0.0.0.0, who can connect?
A: Anyone on the internet — a huge security risk unless behind a secure firewall.
Enable Firewall Rules
Use a firewall (like ufw
) to allow only your application or admin machine to connect:
sudo ufw allow from 192.168.1.100 to any port 27017
sudo ufw enable
This ensures no one else can connect to MongoDB except your trusted IP address.
Use TLS/SSL for Encrypted Connections
To encrypt communication between your app and MongoDB, use TLS/SSL certificates. This prevents packet sniffers from stealing credentials and sensitive data.
mongod --tlsMode requireTLS --tlsCertificateKeyFile /etc/ssl/mongo.pem
Q: Do you need SSL for local development?
A: Not always, but it’s mandatory in production and remote setups, especially when using MongoDB Atlas.
Avoid Using the Default Port in Production
MongoDB runs on port 27017
by default. Change it to something else in production environments to reduce the risk of automated attacks:
net:
port: 27333
Restart the service to reflect the new port.
Keep MongoDB Updated
Always use the latest stable version of MongoDB. Each version includes security patches and performance improvements. Use your system’s package manager to upgrade:
sudo apt update
sudo apt upgrade mongodb-org
Audit Logs (Optional but Recommended)
You can enable MongoDB audit logging to track all access and changes. This is especially useful in enterprise environments or for compliance needs.
Summary
- Enable authentication and create users with appropriate roles
- Restrict network access to trusted IPs
- Use firewalls and secure communication (TLS)
- Avoid running on default port in production
- Keep MongoDB up to date
These practices help ensure your MongoDB deployments are safe, secure, and production-ready.