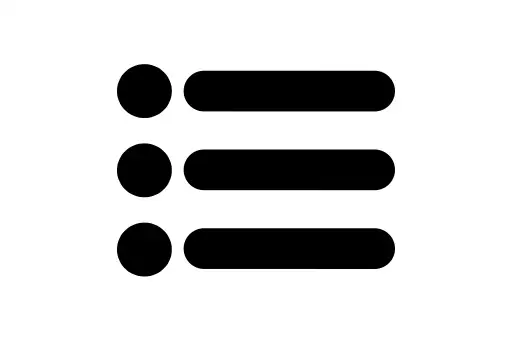
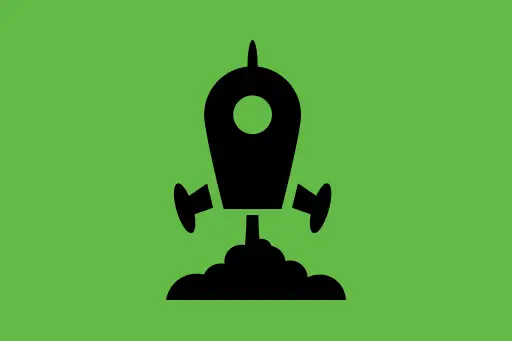
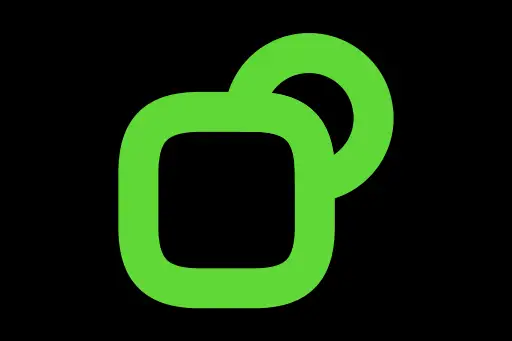
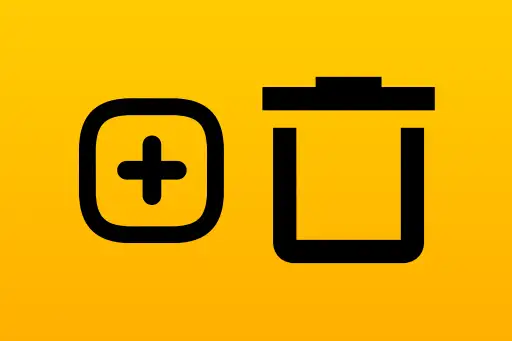
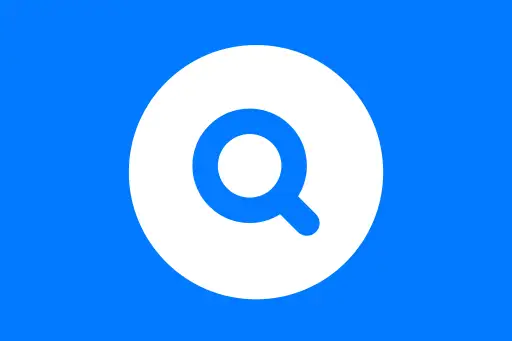
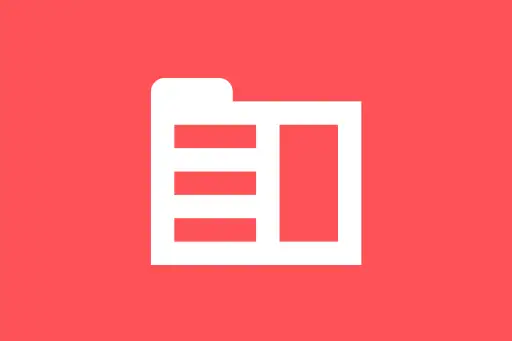
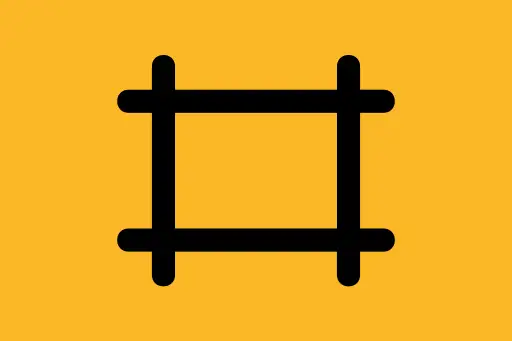
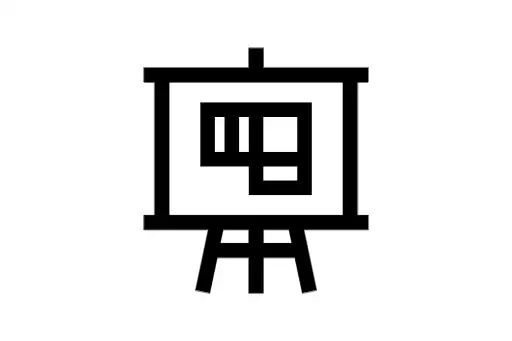
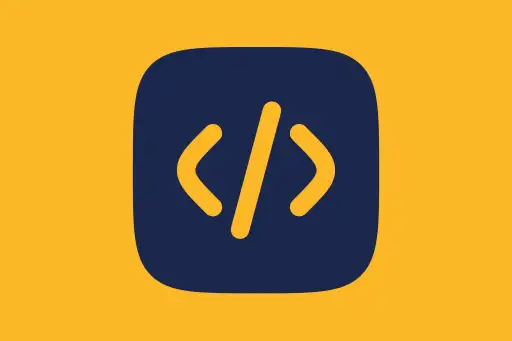
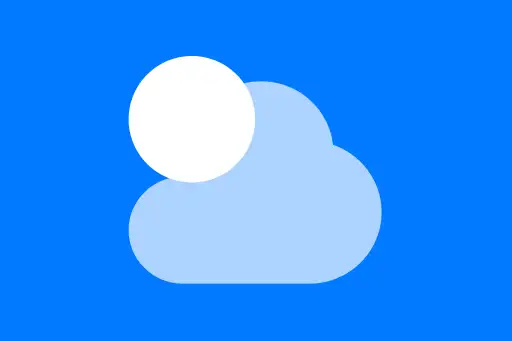
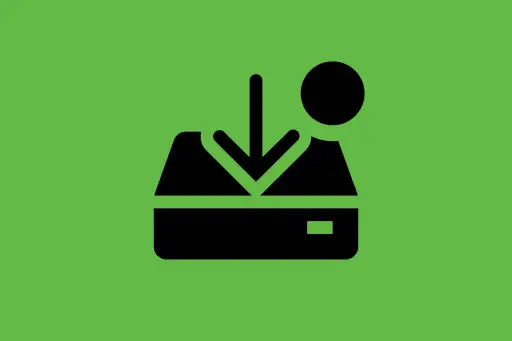
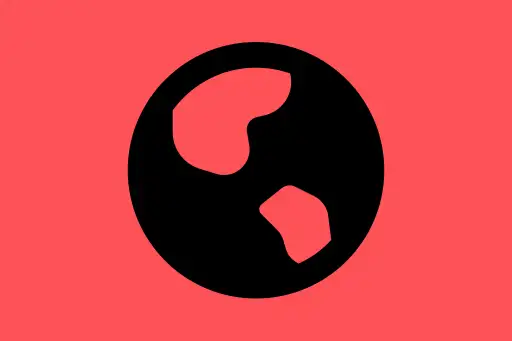
MongoDB Schema Validation with JSON Schema
Next Topic ⮕Defining Custom Validation Rules in MongoDB
MongoDB Schema Validation with JSON Schema
Unlike SQL databases, MongoDB is schema-less by default. This flexibility is great during early development, but as your application grows, having a consistent structure becomes important. MongoDB solves this with schema validation using JSON Schema.
Schema validation allows you to define rules for the documents inserted into a collection — such as required fields, data types, minimum/maximum values, string lengths, and more.
Why Use Schema Validation?
- To ensure all documents follow a consistent structure
- To prevent accidental insertion of malformed data
- To enforce data types and required fields
Q: If MongoDB is schema-less, why would we want validation?
A: Schema-less doesn't mean "anything goes forever." In production, clean and predictable data is important. Validation acts as a safety net while retaining MongoDB's flexibility.
Creating a Collection with JSON Schema Validation
Let’s create a users
collection where every document must:
- Have a
name
field (string, required) - Have an
age
field (integer, optional but must be between 18 and 99) - Optionally have an
email
field (string format)
Shell Command to Create Collection with Validator
db.createCollection("users", {
validator: {
$jsonSchema: {
bsonType: "object",
required: ["name"],
properties: {
name: {
bsonType: "string",
description: "must be a string and is required"
},
age: {
bsonType: "int",
minimum: 18,
maximum: 99,
description: "must be an integer in [18, 99]"
},
email: {
bsonType: "string",
pattern: "^.+@.+\..+$",
description: "must be a valid email format"
}
}
}
},
validationLevel: "strict",
validationAction: "error"
});
Explanation:
required: ["name"]
makesname
mandatorybsonType
ensures proper data typespattern
enforces regex for the email formatvalidationLevel: "strict"
means all inserts and updates are validatedvalidationAction: "error"
blocks invalid documents
Inserting a Valid Document
db.users.insertOne({
name: "Alice",
age: 30,
email: "alice@example.com"
});
{ acknowledged: true, insertedId: ObjectId("...") }
Inserting an Invalid Document
Let’s try inserting a user under 18:
db.users.insertOne({
name: "Bob",
age: 15
});
WriteError({ index: 0, code: 121, errmsg: 'Document failed validation', ... })
Explanation: The insert fails because age < 18 violates the minimum
rule in the schema.
Intuition Check
Q: What happens if you forget to include the name
field?
A: The insert will fail because name
is marked as required
in the schema.
Updating Documents and Validation
Even updates are validated under strict
mode.
db.users.updateOne(
{ name: "Alice" },
{ $set: { age: 120 } }
);
WriteError({ code: 121, errmsg: 'Document failed validation' })
Explanation: The update fails because age cannot exceed 99 as per the schema rule.
Modifying Schema Validation Rules
You can alter the validation rules on an existing collection using collMod
:
db.runCommand({
collMod: "users",
validator: {
$jsonSchema: {
bsonType: "object",
required: ["name", "email"],
properties: {
name: { bsonType: "string" },
email: {
bsonType: "string",
pattern: "^.+@.+\..+$"
}
}
}
}
});
{ ok: 1 }
Validation Levels and Actions
- validationLevel: "strict" (all operations), "moderate" (only new/modified fields)
- validationAction: "error" (reject invalid), "warn" (log a warning but allow)
Conclusion
MongoDB’s JSON Schema validation allows you to retain flexibility while enforcing structure where needed. You can specify required fields, types, ranges, regex patterns, and more — all helping ensure clean, consistent data without giving up NoSQL advantages.
Up next, you'll learn how to design schema relationships like one-to-many or many-to-many using embedded documents and references.