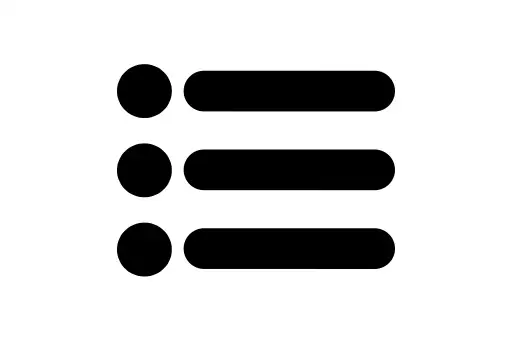
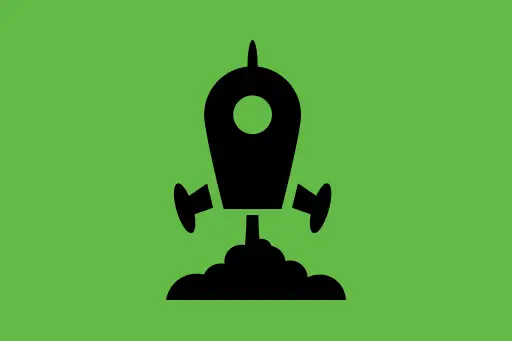
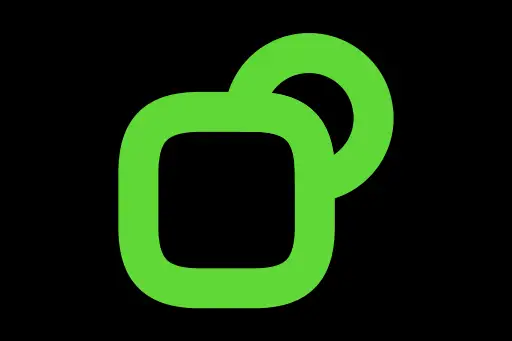
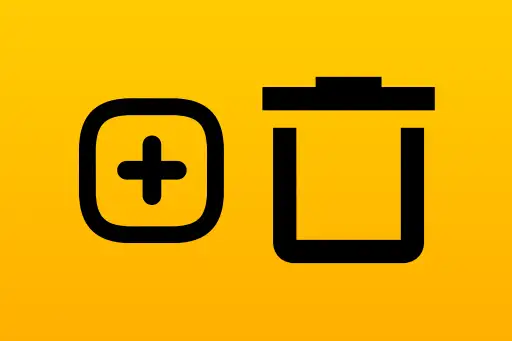
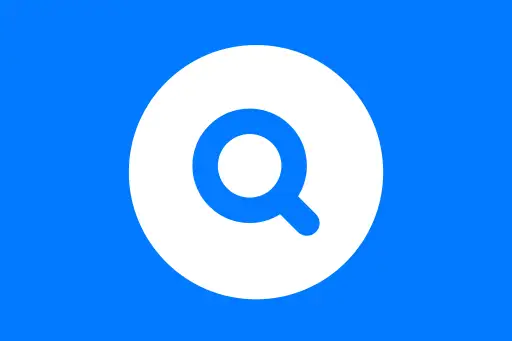
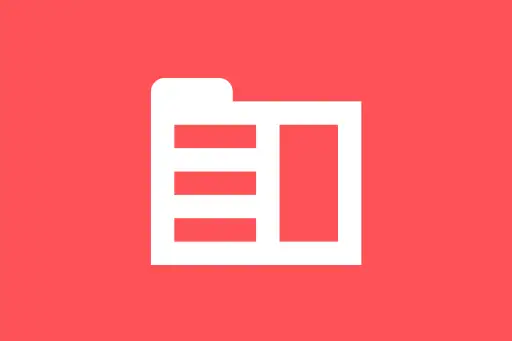
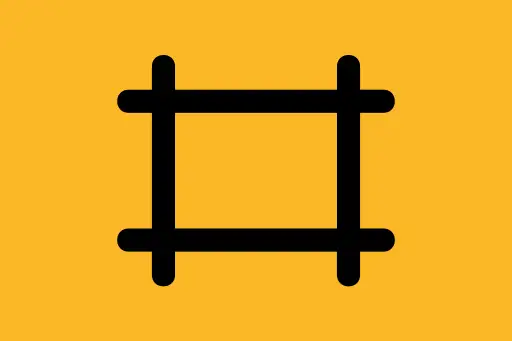
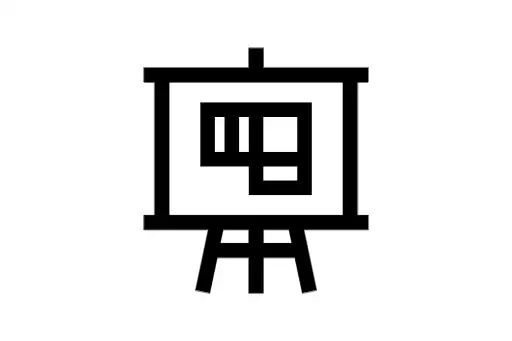
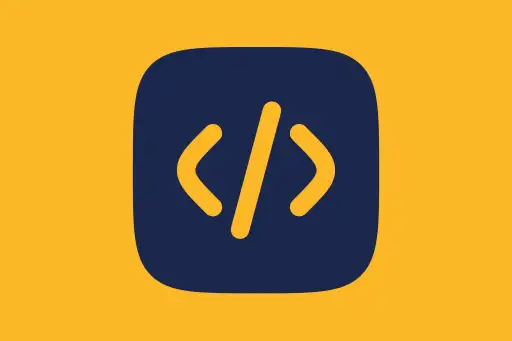
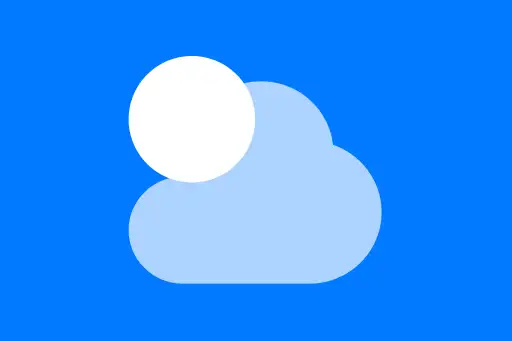
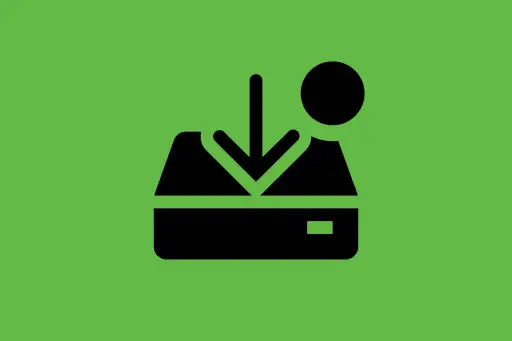
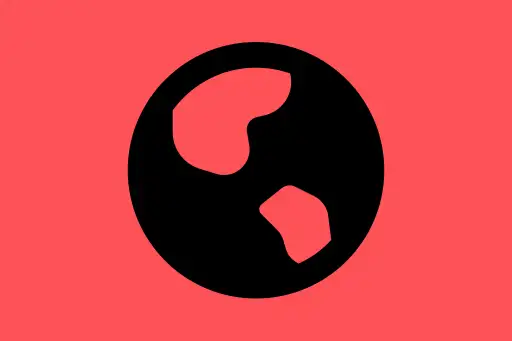
MongoDB with Python using PyMongo
Next Topic ⮕MongoDB with Node.js using Mongoose
MongoDB with Python using PyMongo
PyMongo is the official Python library for interacting with MongoDB. It allows Python developers to easily connect, read, write, and manipulate data in MongoDB using familiar Python syntax.
Installing PyMongo
First, make sure Python is installed. Then, install PyMongo using pip:
pip install pymongo
Connecting to MongoDB
Let’s start by connecting to a local MongoDB instance.
from pymongo import MongoClient
# Connect to the local MongoDB server
client = MongoClient("mongodb://localhost:27017/")
# Create or access a database
db = client["school"]
# Create or access a collection
students = db["students"]
Explanation: We create a connection using MongoClient
, then access a database and a collection. If they don't exist, MongoDB will create them on the fly when we insert data.
Inserting Documents
# Insert one document
students.insert_one({
"name": "Alice",
"age": 22,
"subjects": ["Math", "Science"]
})
# Insert multiple documents
students.insert_many([
{"name": "Bob", "age": 24},
{"name": "Charlie", "age": 21, "subjects": ["History"]}
])
Inserted IDs: [ObjectId(...), ObjectId(...)]
Explanation: insert_one()
adds a single document, while insert_many()
can insert multiple documents at once. You don’t need to define a schema beforehand.
Querying Documents
To read documents from the collection:
# Find one student
student = students.find_one({"name": "Alice"})
print(student)
# Find all students older than 21
for student in students.find({"age": {"$gt": 21}}):
print(student)
{'_id': ObjectId('...'), 'name': 'Alice', 'age': 22, 'subjects': ['Math', 'Science']} {'_id': ObjectId('...'), 'name': 'Bob', 'age': 24}
Q: Why do we use $gt
instead of Python's >
?
A: MongoDB uses its own set of operators like $gt
(greater than), $lt
(less than), etc., which PyMongo maps directly to its queries.
Updating Documents
You can update documents using update_one()
or update_many()
.
# Update one document
students.update_one(
{"name": "Bob"},
{"$set": {"age": 25}}
)
# Update many documents
students.update_many(
{"age": {"$gt": 21}},
{"$inc": {"age": 1}} # Increment age by 1
)
Modified count: 1 Modified count: 2
Explanation: The $set
operator sets a new value, while $inc
increases the current value. These MongoDB operators work the same way in Python with PyMongo.
Deleting Documents
# Delete one student
students.delete_one({"name": "Charlie"})
# Delete all students younger than 23
students.delete_many({"age": {"$lt": 23}})
Deleted count: 1 Deleted count: 2
Q: What happens if the filter doesn't match any document?
A: Nothing breaks. The operation runs safely and the deleted count will be 0.
Closing the Connection
client.close()
Best Practice: Always close the connection when done to free resources.
Summary
- PyMongo is the official driver to use MongoDB with Python
- You can connect to local or remote databases using
MongoClient
- Perform CRUD operations easily with methods like
insert_one()
,find()
,update_many()
, anddelete_one()
- MongoDB operators like
$gt
,$set
, and$inc
are used in PyMongo as they are
Next Steps
In the next lesson, we’ll explore how to use MongoDB with Node.js using Mongoose — a popular ODM library.