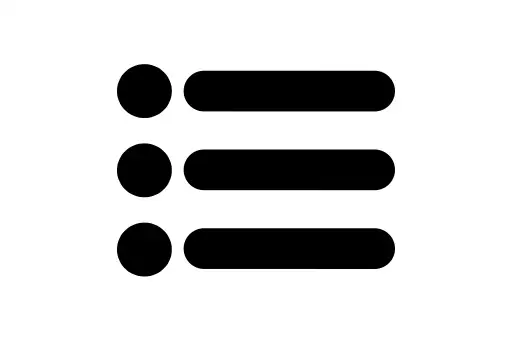
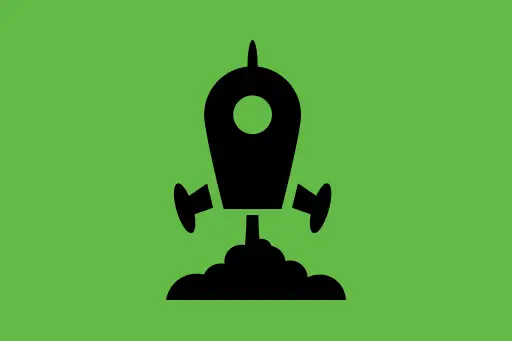
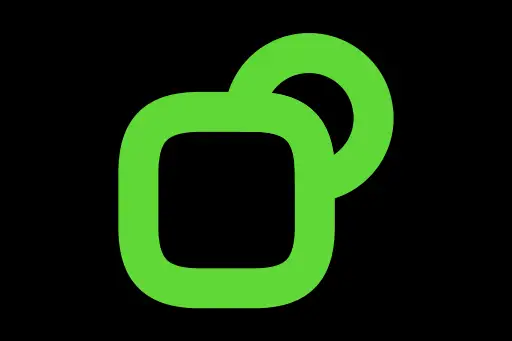
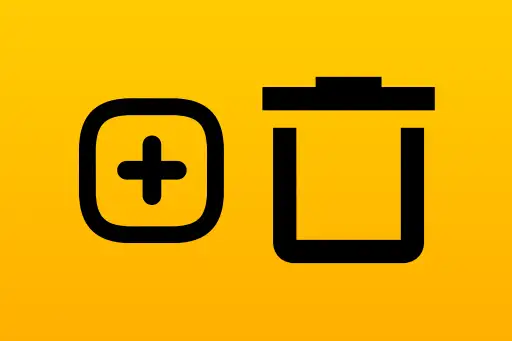
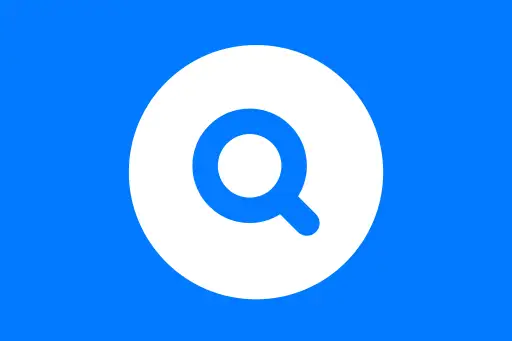
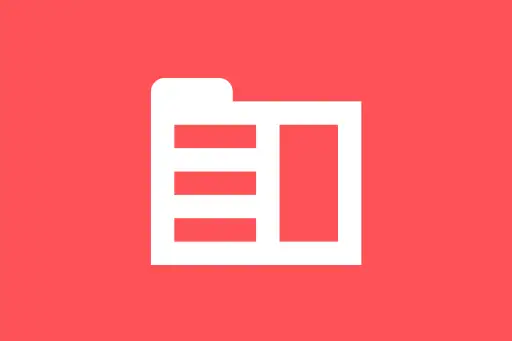
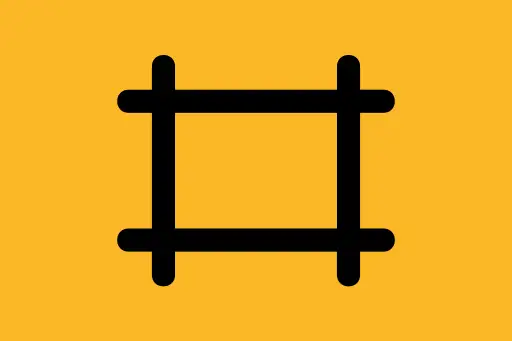
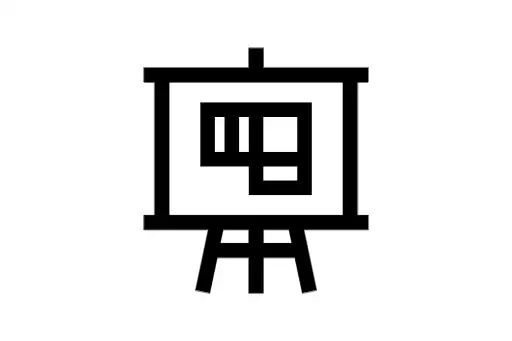
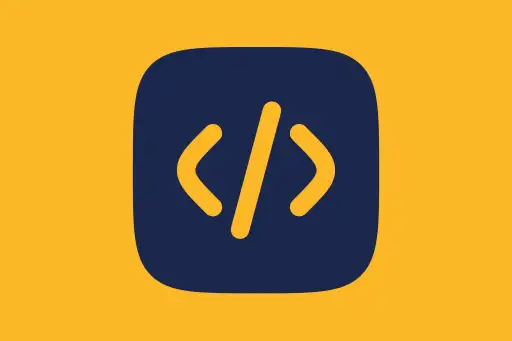
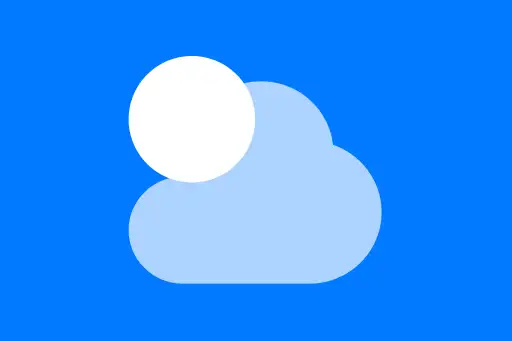
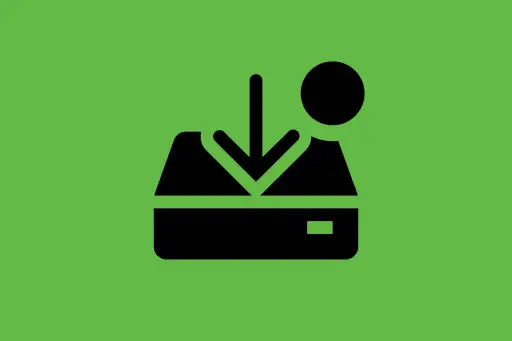
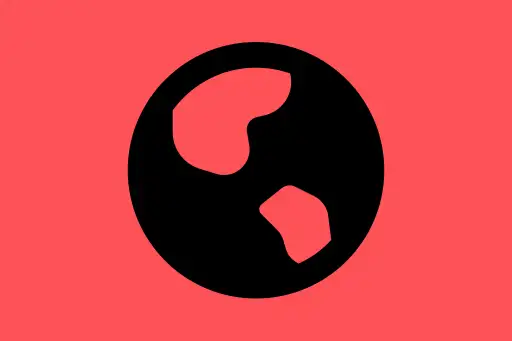
MongoDB with Node.js using Mongoose
Next Topic ⮕Setting Up a Free MongoDB Atlas Cluster
MongoDB with Node.js using Mongoose
MongoDB can be accessed in Node.js using a powerful Object Data Modeling (ODM) library called Mongoose. It allows you to define schemas, models, and provides a convenient API to interact with the database — making your code clean and manageable.
What is Mongoose?
Mongoose is an npm package that acts as a bridge between MongoDB and your Node.js application. It lets you define models with a schema and provides methods to perform CRUD operations.
Question: Why not use the native MongoDB driver?
Answer: While the native driver works fine, Mongoose offers schema validation, middleware, and a more developer-friendly API, especially for beginners.
Installing Mongoose
Before we begin, let’s set up a new Node.js project and install Mongoose.
mkdir mongoose-demo
cd mongoose-demo
npm init -y
npm install mongoose
Connecting to MongoDB
Create a file called app.js
and add the following connection code:
// app.js
const mongoose = require('mongoose');
mongoose.connect('mongodb://127.0.0.1:27017/mongoose_course')
.then(() => console.log("Connected to MongoDB"))
.catch(err => console.error("Connection failed", err));
Connected to MongoDB
Explanation: The URI points to a local MongoDB instance on port 27017. If you’re using MongoDB Atlas, replace the URI with your cluster's connection string.
Defining a Schema and Model
Let’s define a simple User model with fields like name, email, and age.
const userSchema = new mongoose.Schema({
name: String,
email: { type: String, required: true, unique: true },
age: Number
});
const User = mongoose.model('User', userSchema);
Explanation: The schema defines the structure of a document. User
becomes a model which represents the users
collection in MongoDB.
Inserting a Document
Add this code in app.js
after the model declaration to create a new user.
async function createUser() {
const user = new User({
name: "Alice",
email: "alice@example.com",
age: 28
});
const result = await user.save();
console.log(result);
}
createUser();
{ _id: ObjectId("..."), name: 'Alice', email: 'alice@example.com', age: 28, __v: 0 }
Question: What is __v
in the output?
Answer: Mongoose adds a version key __v
by default for internal versioning. It helps with concurrency control and can be ignored in most beginner cases.
Reading Documents
To find all users:
async function getUsers() {
const users = await User.find();
console.log(users);
}
getUsers();
[ { _id: ObjectId("..."), name: 'Alice', email: 'alice@example.com', age: 28, __v: 0 } ]
To filter users by age:
const youngUsers = await User.find({ age: { $lt: 30 } });
Updating a Document
Let’s update Alice’s age.
async function updateUser() {
const result = await User.updateOne({ name: "Alice" }, {
$set: { age: 29 }
});
console.log(result);
}
updateUser();
{ acknowledged: true, modifiedCount: 1, matchedCount: 1 }
Question: What does matchedCount
mean?
Answer: It shows how many documents matched the filter condition. modifiedCount
tells how many were actually updated.
Deleting a Document
To delete Alice from the database:
async function deleteUser() {
const result = await User.deleteOne({ name: "Alice" });
console.log(result);
}
deleteUser();
{ acknowledged: true, deletedCount: 1 }
Summary
In this lesson, you learned how to:
- Set up Mongoose in a Node.js project
- Define a schema and create a model
- Perform CRUD operations with ease
Mongoose abstracts away the complexity of dealing with raw MongoDB queries, making it easier to build full-stack applications. In the next topic, we'll use MongoDB Atlas to connect your application to a cloud-hosted MongoDB cluster.