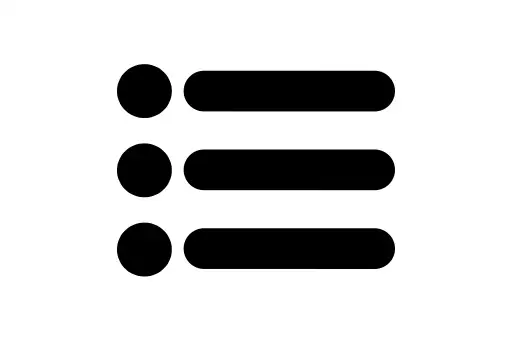
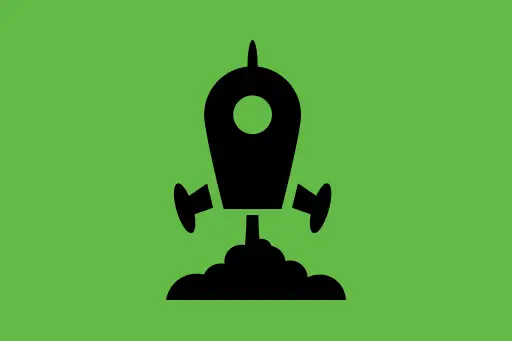
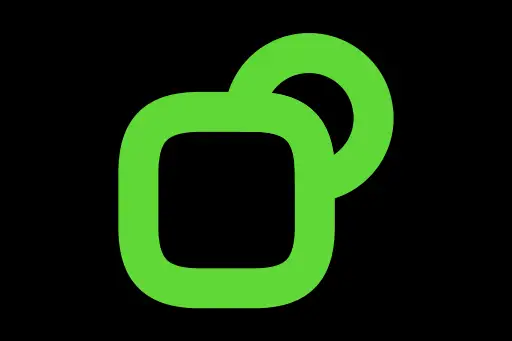
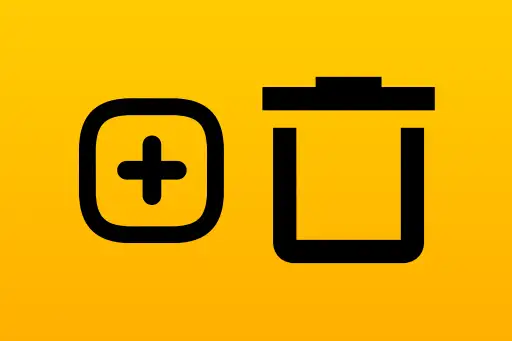
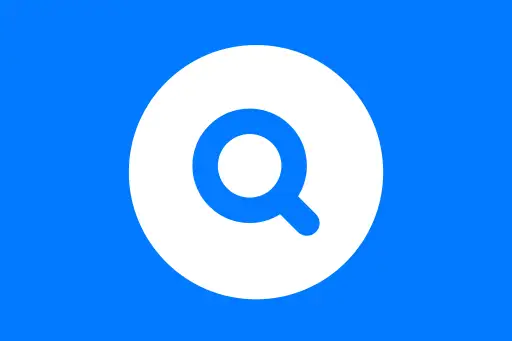
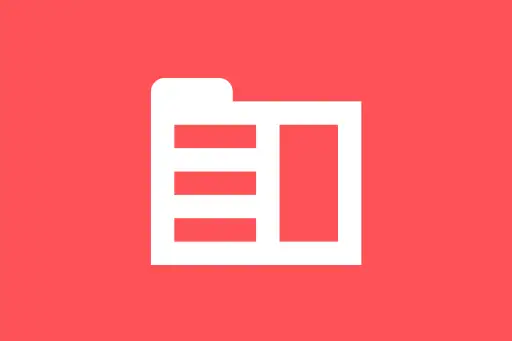
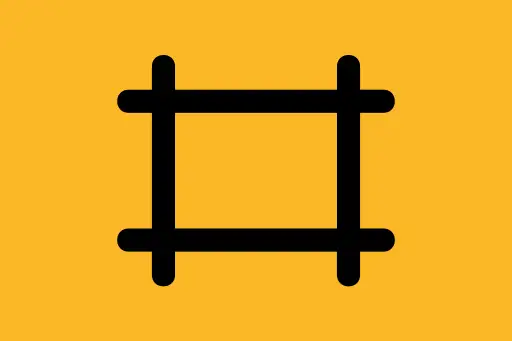
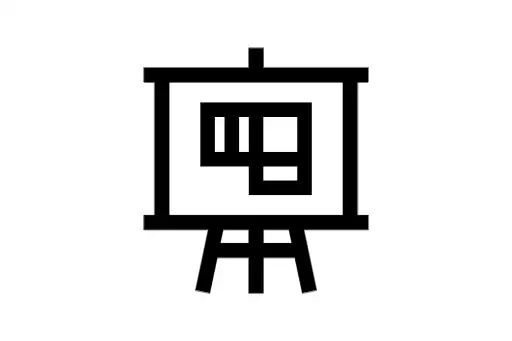
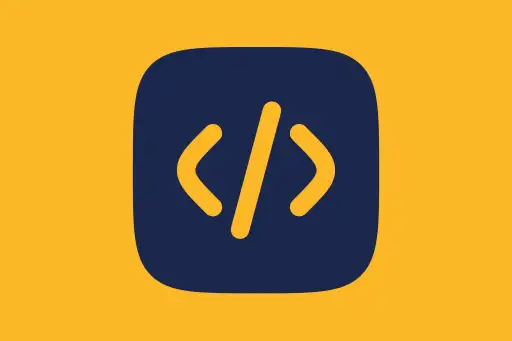
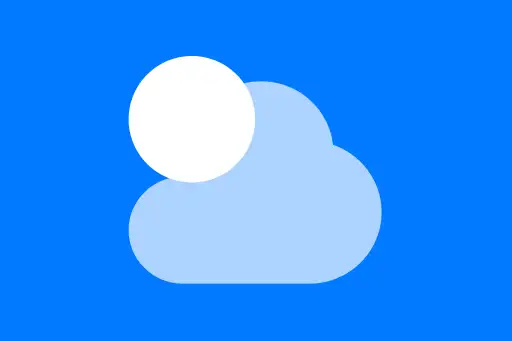
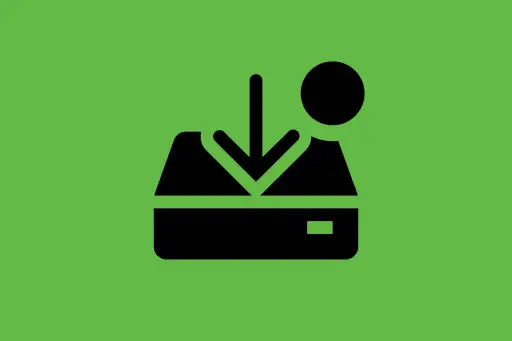
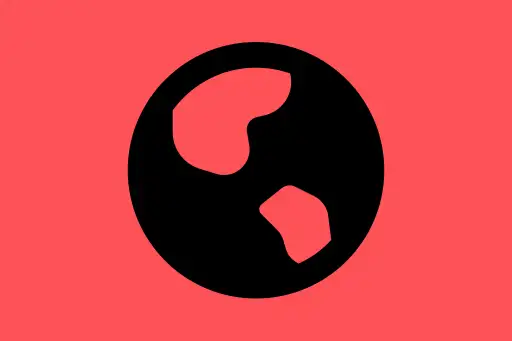
What are Indexes and Why Use Them
Next Topic ⮕Creating Single Field and Compound Indexes in MongoDB
What are Indexes and Why Use Them
Indexes in MongoDB are special data structures that store a portion of your data in a way that makes queries faster — similar to how an index in a book helps you quickly locate a topic instead of reading every page.
Why Do We Need Indexes?
Imagine you have a collection of 1 million user documents and you want to find a user by their email address. Without an index, MongoDB would have to scan every document in the collection — this is called a collection scan and it is slow.
With an index on the email
field, MongoDB can jump directly to the correct document — just like flipping to a specific word in a book’s index.
Default Index (_id)
Every document in MongoDB has a unique _id
field, and by default, MongoDB creates an index on this field automatically.
db.users.find({ _id: ObjectId("6631b20f4a2d9c2f1f5e6c33") });
This query is efficient because it uses the default _id
index.
How to Check Existing Indexes
You can see which indexes exist on a collection:
db.users.getIndexes();
[ { v: 2, key: { _id: 1 }, name: "_id_" } ]
Creating a Single Field Index
Suppose we frequently query users by email. We can create an index on the email
field to speed up those queries:
db.users.createIndex({ email: 1 });
{ "createdCollectionAutomatically": false, "numIndexesBefore": 1, "numIndexesAfter": 2, "ok": 1 }
Explanation: The 1
means ascending order. You can also use -1
for descending. This index will now improve all queries filtering by email
.
Example: Query Without vs With Index
Without Index:
db.users.find({ email: "alice@example.com" }).explain("executionStats");
"executionStats": { "totalDocsExamined": 1000000, "totalKeysExamined": 0, ... }
With Index:
db.users.createIndex({ email: 1 });
db.users.find({ email: "alice@example.com" }).explain("executionStats");
"executionStats": { "totalDocsExamined": 1, "totalKeysExamined": 1, ... }
Explanation: With the index in place, MongoDB directly finds the matching document without scanning the entire collection. This results in significant performance gains.
Intuition Check
Q: Can indexes make all types of queries faster?
A: No. Indexes help most when you're searching or sorting based on the indexed field. But they also consume space and slow down writes, so use them wisely.
Creating Compound Indexes
If you often query using multiple fields, like email
and status
, you can create a compound index:
db.users.createIndex({ email: 1, status: 1 });
Explanation: This index is useful for queries that filter on email
and optionally status
. Order matters in compound indexes — this one won’t optimize queries that filter only by status
.
Removing an Index
To remove an index you no longer need:
db.users.dropIndex("email_1");
Viewing Index Usage
To check how often an index is used:
db.users.aggregate([ { $indexStats: {} } ]);
Summary
- Indexes make read operations faster by avoiding full collection scans.
- MongoDB automatically creates an index on
_id
. - You can create single-field and compound indexes depending on your query patterns.
- Use
explain()
to verify if your query uses an index effectively.
Next Up
Now that you understand what indexes are and why they matter, we'll learn about different types of indexes like compound and multikey indexes — and how to optimize real-world queries using them.
Comments
Loading comments...