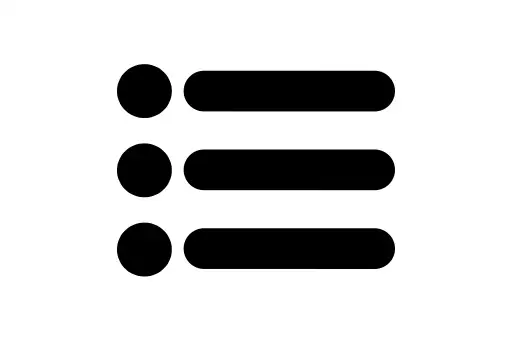
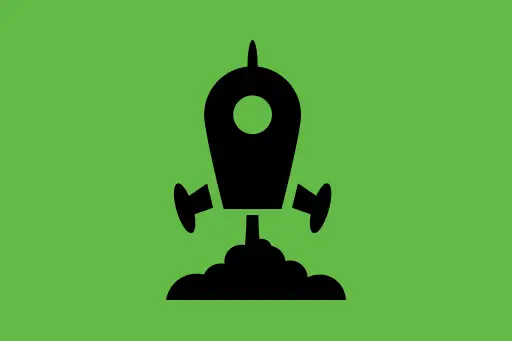
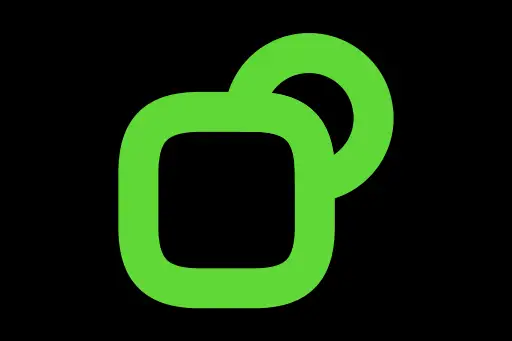
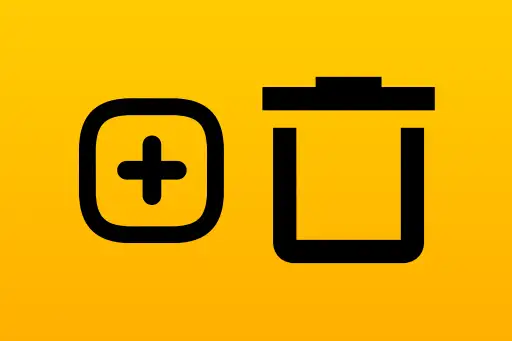
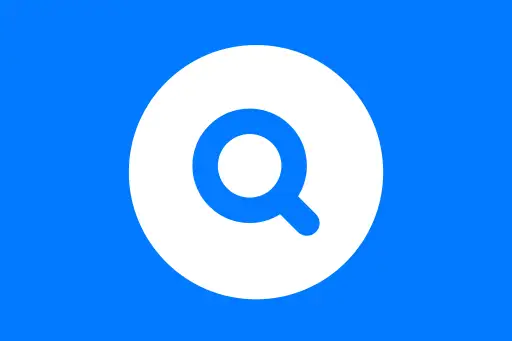
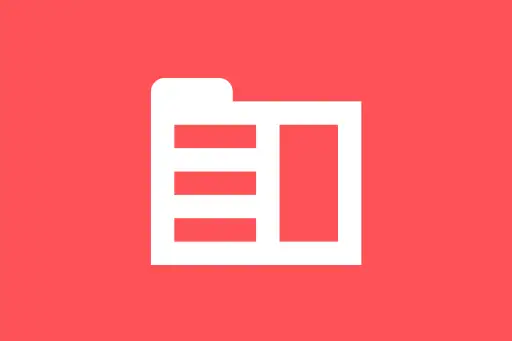
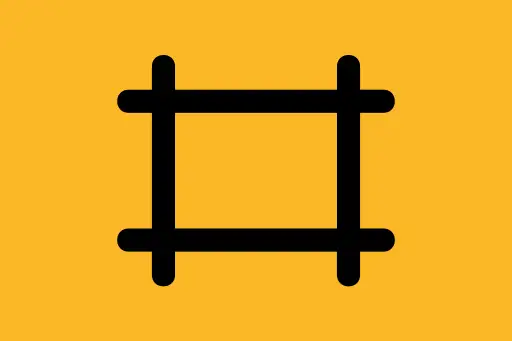
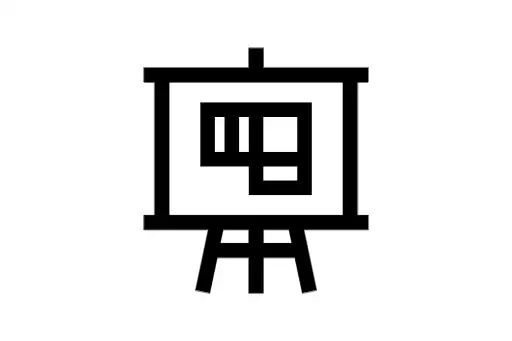
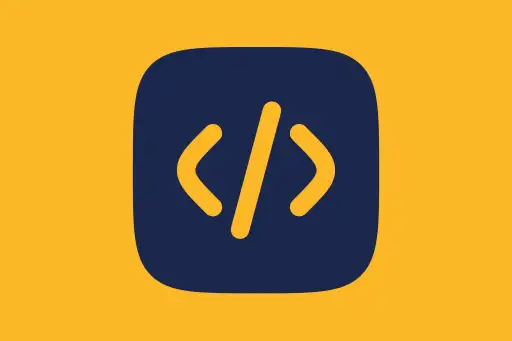
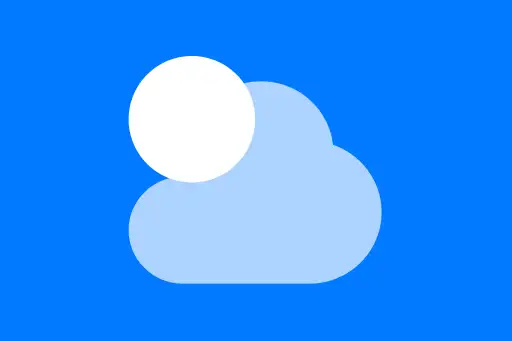
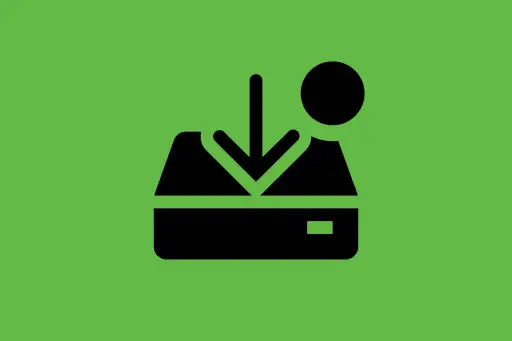
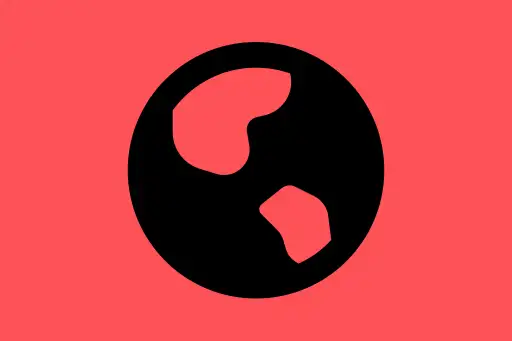
Delete Operations in MongoDB
Delete Operations in MongoDB
In MongoDB, delete operations are used to remove documents from a collection. You can choose to delete a single document, multiple documents, or even an entire collection depending on the requirement.
MongoDB provides the following methods to perform deletions:
deleteOne()
– deletes the first matching documentdeleteMany()
– deletes all documents that match the filterdrop()
– deletes the entire collection
deleteOne() – Delete a Single Document
This method deletes the first document that matches the filter criteria.
Example:
Let's assume we have a users
collection like this:
db.users.insertMany([
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 },
{ name: "Alice", age: 28 }
]);
Now we want to delete only the first user named "Alice".
db.users.deleteOne({ name: "Alice" });
{ acknowledged: true, deletedCount: 1 }
Explanation: Even though there are two documents with the name "Alice", only the first match is deleted by deleteOne()
. This is useful when you're sure that you want to remove only one occurrence.
Q: What happens if no document matches the filter?
A: Nothing is deleted, and deletedCount
will be 0
.
deleteMany() – Delete Multiple Documents
Use this when you want to delete all documents that match a condition.
Example:
We still have the remaining documents:
db.users.find();
{ _id: ..., name: "Bob", age: 30 } { _id: ..., name: "Alice", age: 28 }
Now delete all users named "Alice":
db.users.deleteMany({ name: "Alice" });
{ acknowledged: true, deletedCount: 1 }
Explanation: This removes all documents where the name is "Alice". It's useful when you want to clear multiple records at once.
Q: Can deleteMany()
be used without a filter?
A: Yes, but be cautious. Using an empty filter will delete all documents in the collection.
Dangerous Example – Delete All:
db.users.deleteMany({});
{ acknowledged: true, deletedCount: X }
Warning: This command will remove all documents in the collection. Always double-check filters before running delete operations.
drop() – Delete Entire Collection
Sometimes you may want to delete the entire collection, not just documents.
Example:
Let’s say we want to remove the users
collection completely:
db.users.drop();
true
Explanation: This deletes the entire users
collection including all documents and metadata. After this, running db.users.find()
will show an error since the collection no longer exists.
Command Line Equivalent
You can also use the Mongo shell via terminal or command prompt.
mongo
use test
db.users.deleteOne({ name: "Alice" })
Summary
deleteOne()
– deletes the first matching documentdeleteMany()
– deletes all matching documentsdrop()
– deletes the entire collection
Delete operations in MongoDB are straightforward but powerful. Make sure to test with filters before applying on large datasets to avoid accidental data loss.
Comments
Loading comments...