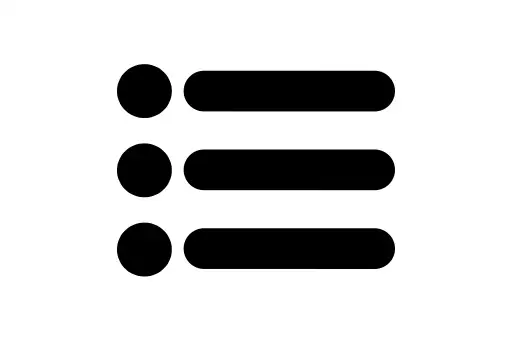
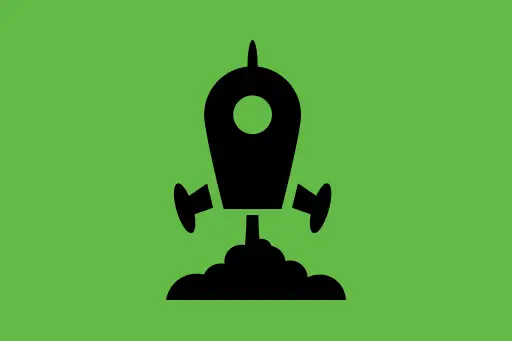
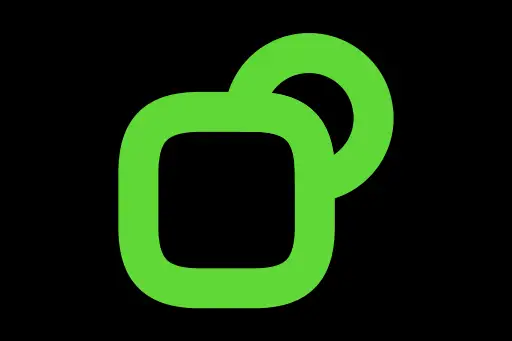
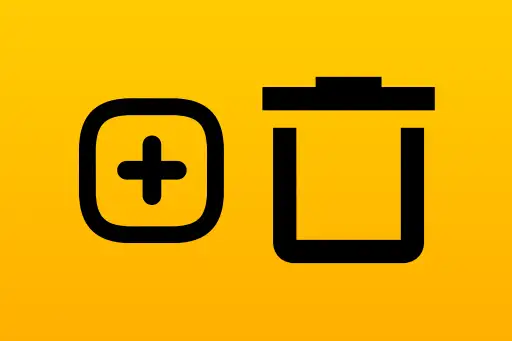
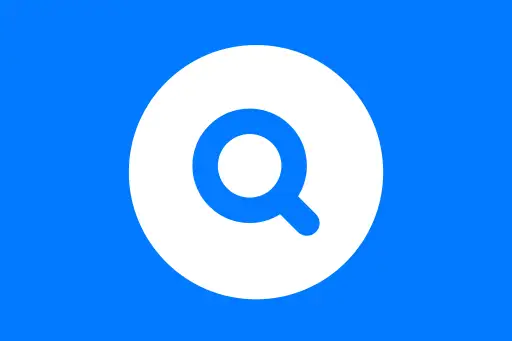
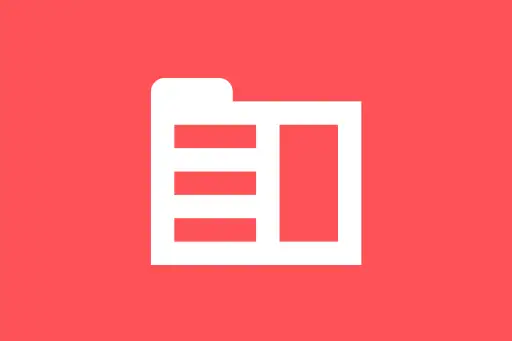
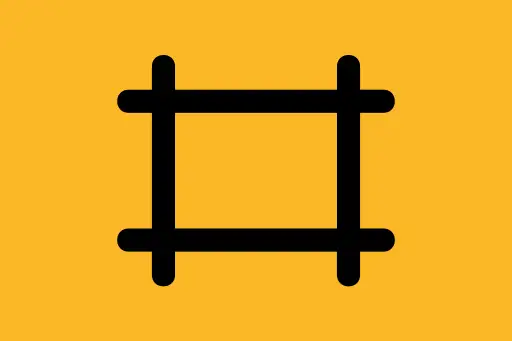
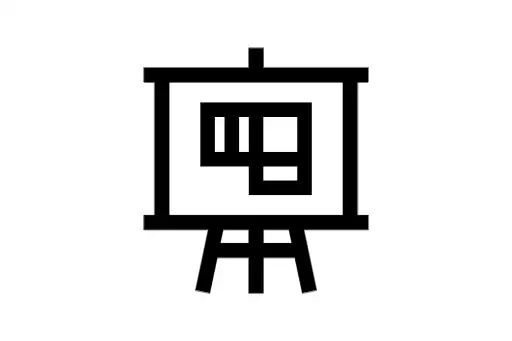
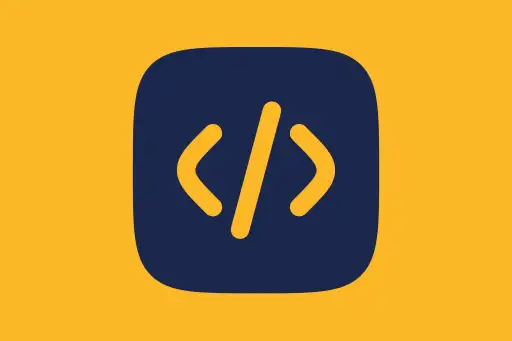
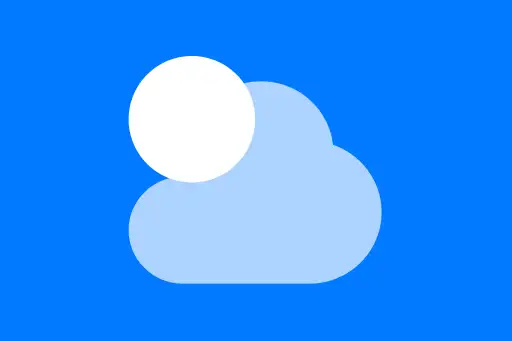
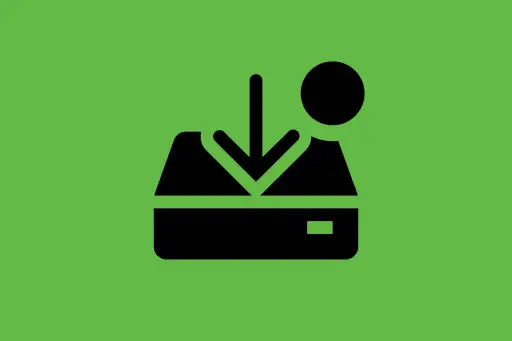
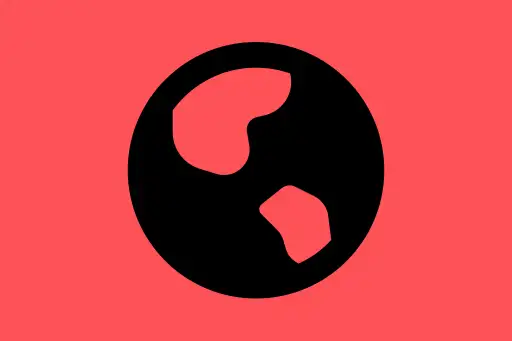
MongoDB Data Types
MongoDB Data Types
In MongoDB, documents are stored in a format called BSON (Binary JSON). BSON supports a rich set of data types that allow you to store and query your data efficiently.
Let’s explore the most commonly used MongoDB data types with examples.
String
Strings are used to store text data. They must be enclosed in double quotes.
db.users.insertOne({
name: "Alice"
});
{ acknowledged: true, insertedId: ObjectId("...") }
Q: Can we use single quotes for string values in MongoDB?
A: No. MongoDB strictly uses double quotes for keys and string values in BSON/JSON format.
Number (Int, Double)
MongoDB supports integers and floating-point numbers.
db.products.insertOne({
name: "Mouse",
price: 499, // integer
rating: 4.5 // floating point
});
{ acknowledged: true, insertedId: ObjectId("...") }
Note: MongoDB automatically identifies whether the number is an integer or double based on the value.
Boolean
Booleans store true/false values, commonly used for flags and status indicators.
db.settings.insertOne({
darkMode: true,
notifications: false
});
{ acknowledged: true, insertedId: ObjectId("...") }
Array
Arrays can hold multiple values, including mixed types or nested documents.
db.users.insertOne({
name: "Bob",
hobbies: ["reading", "gaming", "cycling"]
});
{ acknowledged: true, insertedId: ObjectId("...") }
Q: Can arrays in MongoDB store different data types?
A: Yes, arrays can include strings, numbers, booleans, even other arrays or objects.
Object / Embedded Document
MongoDB allows you to store objects (documents within documents), also known as embedded documents.
db.users.insertOne({
name: "Charlie",
address: {
city: "Mumbai",
zip: 400001
}
});
{ acknowledged: true, insertedId: ObjectId("...") }
Q: Why use embedded documents?
A: They allow related data to be stored together, improving query performance and reducing joins.
ObjectId
ObjectId
is the default unique identifier MongoDB assigns to each document. It is a 12-byte hexadecimal string.
db.items.insertOne({ name: "Chair" });
{ acknowledged: true, insertedId: ObjectId("66321faabc1234567890abcd") }
Q: Can I assign my own _id instead of ObjectId?
A: Yes. You can insert documents with custom _id
values if needed:
db.items.insertOne({ _id: "custom123", name: "Table" });
Date
Dates are stored using the ISODate()
function and support date/time operations.
db.logs.insertOne({
event: "User Login",
timestamp: new Date()
});
{ acknowledged: true, insertedId: ObjectId("...") }
Q: How can I query documents created today?
A: You can use $gte
with ISODate()
to filter by time range.
Null
Represents a missing or null value.
db.users.insertOne({
name: "David",
phone: null
});
{ acknowledged: true, insertedId: ObjectId("...") }
Summary Table of Core Types
Type | Example | Description |
---|---|---|
String | "hello" | Text value |
Number | 42, 3.14 | Integer or float |
Boolean | true | Logical value |
Array | [1, 2, 3] | List of values |
Object | { a: 1 } | Nested document |
ObjectId | ObjectId("...") | Unique ID |
Date | ISODate("...") | Timestamp |
Null | null | Missing or empty |
Conclusion
MongoDB provides a flexible set of data types that align naturally with most programming languages. From simple types like strings and numbers to advanced types like embedded objects and arrays, MongoDB makes it easy to model real-world data in a readable and scalable way.
In the next lesson, we’ll explore how to create databases and collections, and how these data types come together in real CRUD operations.