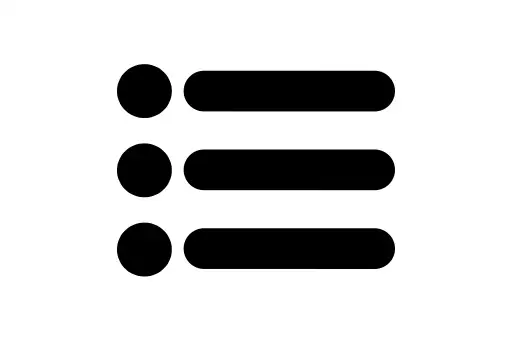
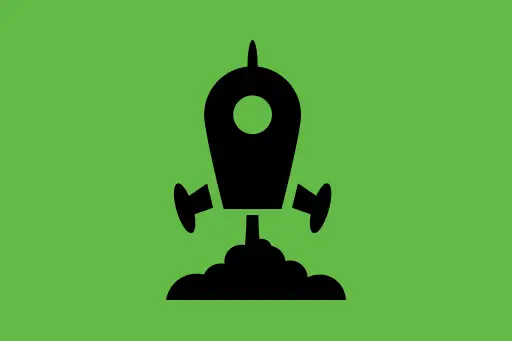
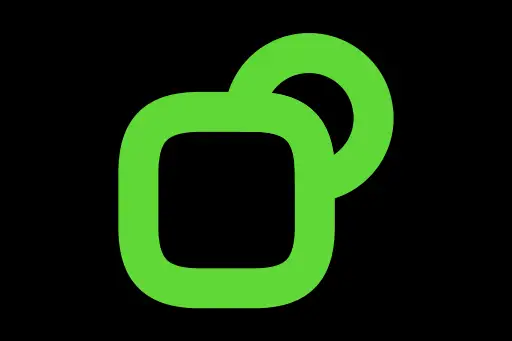
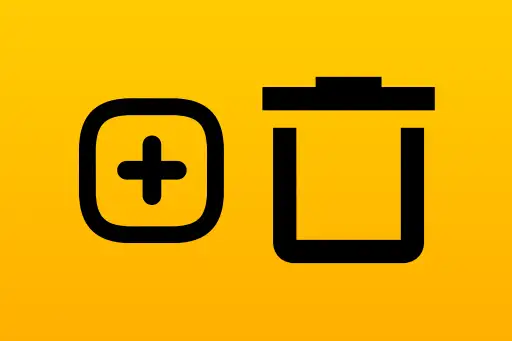
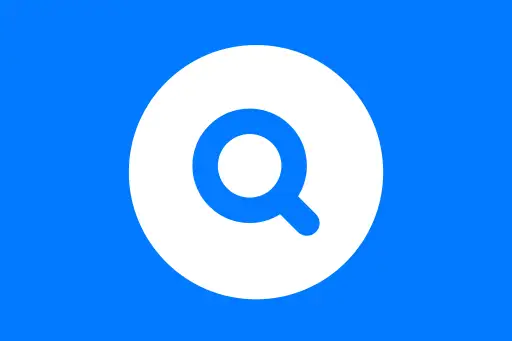
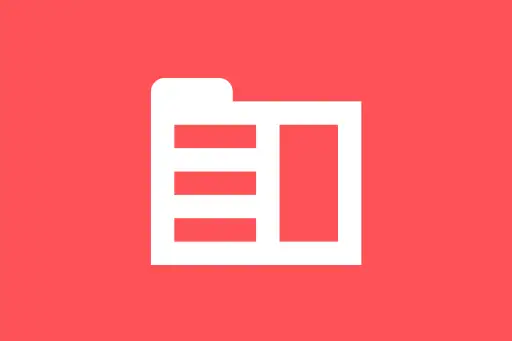
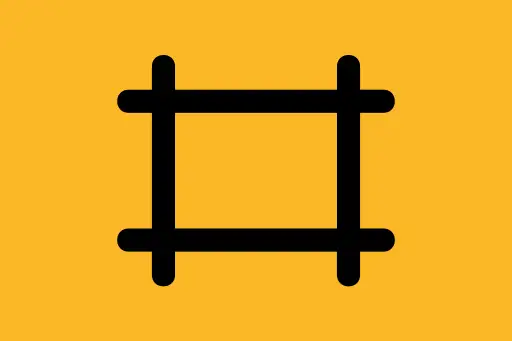
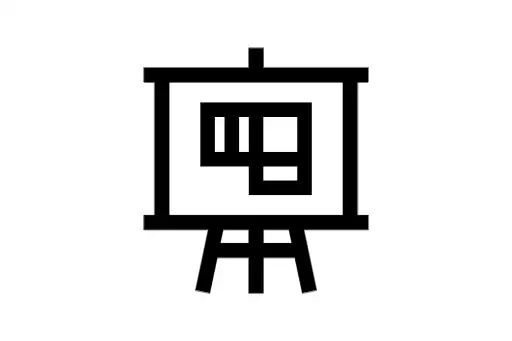
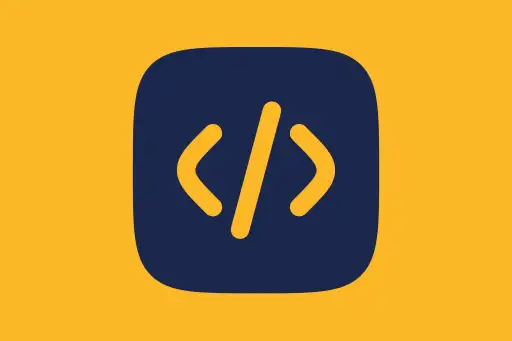
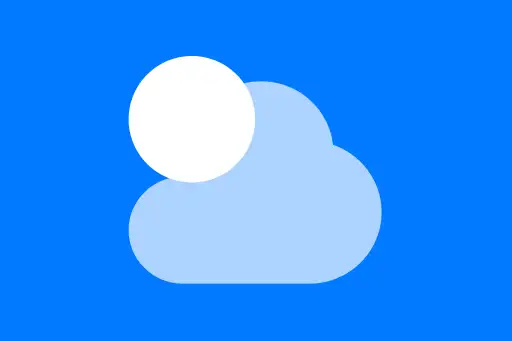
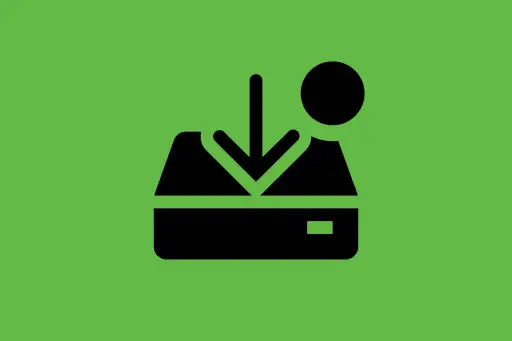
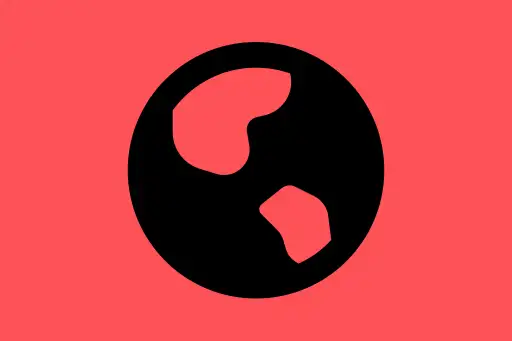
Creating and Managing Databases in Atlas
Next Topic ⮕Connecting MongoDB Atlas to Local Applications
Creating and Managing Databases in Atlas
MongoDB Atlas is the official cloud service provided by MongoDB Inc. It allows developers to run, manage, and scale MongoDB clusters in the cloud — with no need to manually install MongoDB on a server.
In this tutorial, you will learn how to create a MongoDB database in Atlas, access it using MongoDB Compass, and manage your collections and documents from the cloud.
Step 1: Sign Up for MongoDB Atlas
Visit mongodb.com/cloud/atlas and create a free account. Choose the Free Shared Cluster (M0) tier during setup — it’s enough for learning and small projects.
Step 2: Create a Cluster
Once signed in:
- Click on “Build a Cluster”
- Choose a cloud provider (AWS/GCP/Azure) and a region close to you
- Name your cluster (e.g.,
Cluster0
) - Click “Create Cluster”
This takes a couple of minutes to provision.
Step 3: Create a Database and Collection
Once your cluster is ready, click on “Browse Collections” and then:
- Click “Add My Own Data”
- Enter
myFirstDatabase
as the database name - Enter
users
as the collection name - Click “Insert Document” to add your first record
Let’s add this document:
{
"name": "Alice",
"email": "alice@example.com",
"age": 28
}
{ _id: ObjectId("..."), name: "Alice", email: "alice@example.com", age: 28 }
Explanation: You’ve just created a database, a collection, and inserted your first document — all from the browser interface of MongoDB Atlas.
Step 4: Add Database User and IP Whitelist
To connect from your code or Compass, you must:
- Go to “Database Access”
- Create a new user with username and password (e.g.,
mongoUser
) - Go to “Network Access”
- Add your IP address (or use
0.0.0.0/0
to allow access from anywhere)
Step 5: Connect Using Compass or Code
Click “Connect” and choose “Connect using MongoDB Compass”. Copy the connection string and paste it in Compass.
mongodb+srv://mongoUser:<password>@cluster0.xxxxx.mongodb.net/myFirstDatabase?retryWrites=true&w=majority
Replace <password>
with your database password and click “Connect”. You should now see your database and the users
collection with the inserted document.
Connecting Using Code (Node.js)
Install MongoDB driver in your Node.js project:
npm install mongodb
Then use the following code:
const { MongoClient } = require('mongodb');
const uri = 'mongodb+srv://mongoUser:<password>@cluster0.xxxxx.mongodb.net/myFirstDatabase?retryWrites=true&w=majority';
async function run() {
const client = new MongoClient(uri);
try {
await client.connect();
const database = client.db("myFirstDatabase");
const collection = database.collection("users");
const user = { name: "Bob", email: "bob@example.com", age: 32 };
const result = await collection.insertOne(user);
console.log("Inserted:", result.insertedId);
} finally {
await client.close();
}
}
run().catch(console.dir);
Inserted: 651e72f5e60adf003abc1234
Explanation: This Node.js script connects to your MongoDB Atlas cluster and inserts a document into the users
collection.
Question
Q: What if I forget to whitelist my IP address?
A: You’ll get a network timeout error when trying to connect. Always make sure your current IP is whitelisted in the “Network Access” settings in Atlas.
Managing Collections
From the Atlas UI, you can:
- Add new collections or documents
- Edit existing documents directly
- Run filter queries and aggregations from the web UI
Summary
MongoDB Atlas makes it incredibly easy to create and manage databases in the cloud. You can insert documents, connect from applications, and manage everything via a secure and user-friendly dashboard.
In the next topic, you’ll learn how to connect your Atlas database to real-world applications using Python and Node.js drivers.