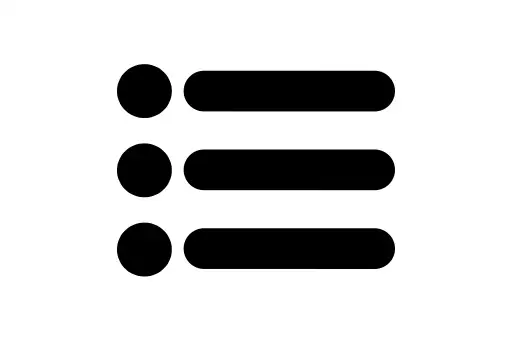
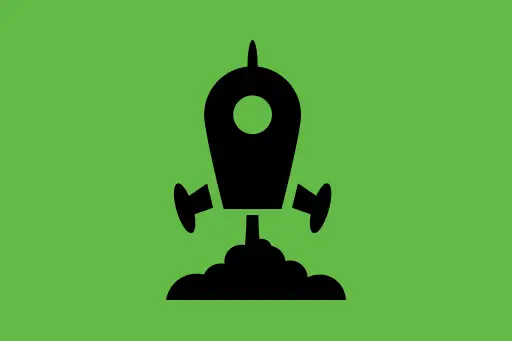
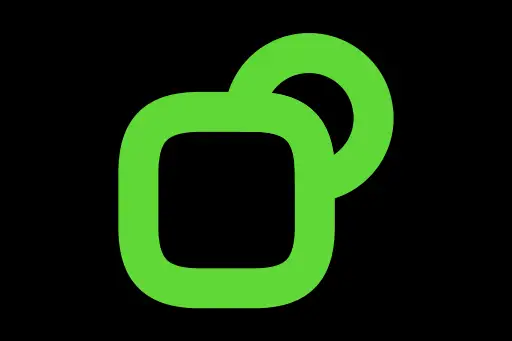
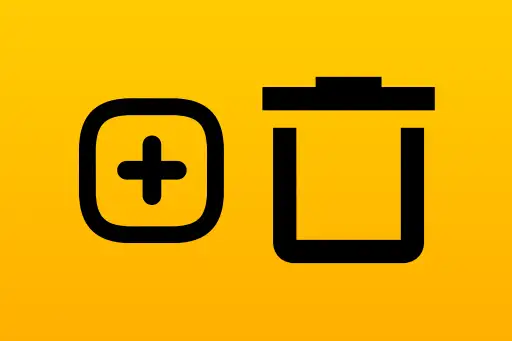
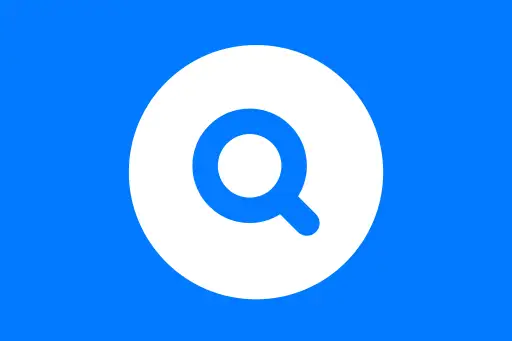
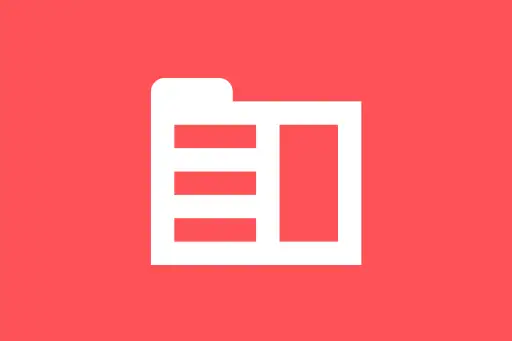
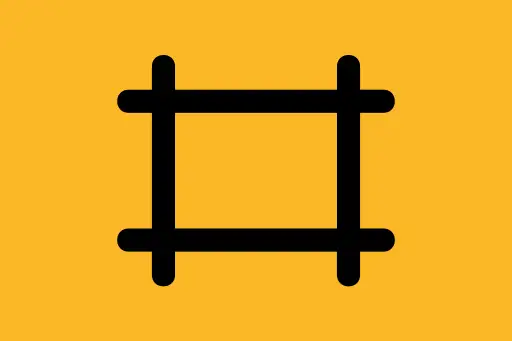
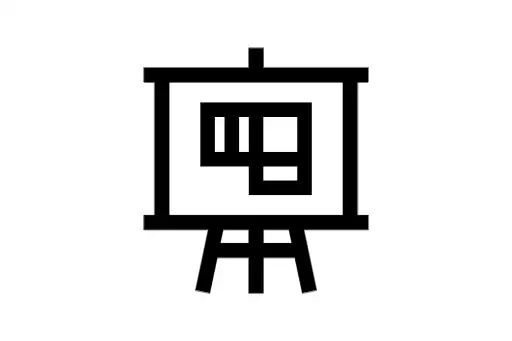
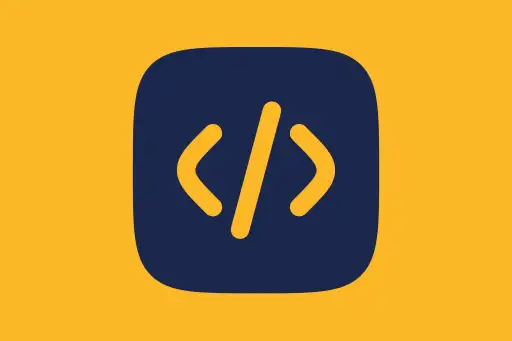
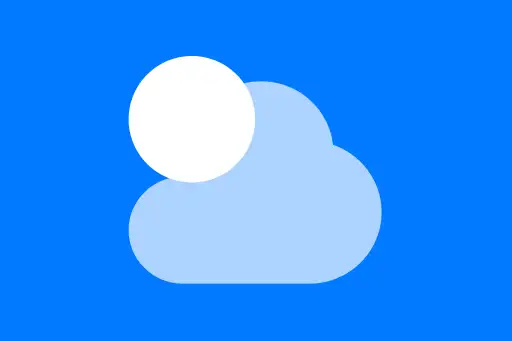
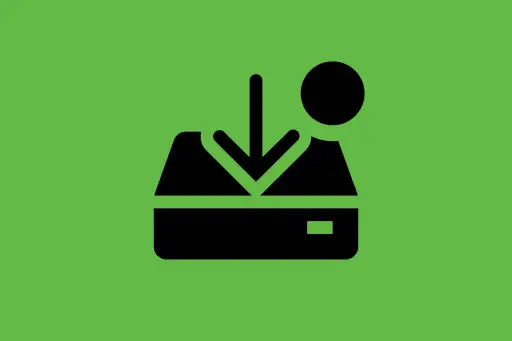
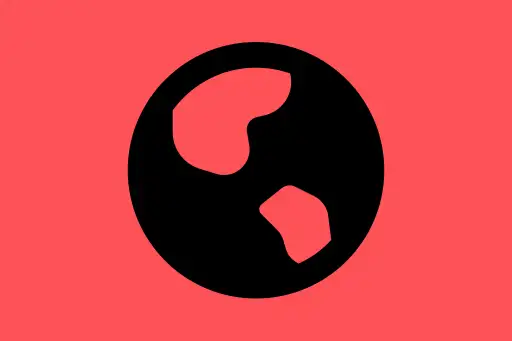
Creating a Database and Collection in MongoDB
Creating a Database and Collection in MongoDB
Before you can store documents in MongoDB, you need a database and a collection. Think of a database as a folder, and a collection as a file inside that folder that holds your JSON-like documents.
Using the MongoDB Shell to Create a Database
To start the Mongo shell, run:
mongo
Now, to create or switch to a database:
use myFirstDB
switched to db myFirstDB
Note: The database is not created immediately. It only gets created when you add a collection and insert data into it.
Creating a Collection
There are two ways to create a collection:
- Implicitly by inserting a document into it
- Explicitly using
db.createCollection()
Method 1: Implicit Creation (Most Common)
db.users.insertOne({
name: "Alice",
age: 25
});
{ acknowledged: true, insertedId: ObjectId("...") }
This command automatically creates the users
collection and inserts the document.
Method 2: Explicit Creation
db.createCollection("products")
{ ok: 1 }
You can now insert documents into the products
collection.
Inserting into the Explicitly Created Collection
db.products.insertOne({
name: "Laptop",
price: 75000,
inStock: true
});
{ acknowledged: true, insertedId: ObjectId("...") }
Intuition Check
Q: Why doesn't MongoDB create the database immediately when you type use myFirstDB
?
A: Because MongoDB uses lazy creation. A database is only created when data is actually inserted. This saves space and keeps the system efficient.
Listing All Databases
show dbs
admin 0.000GB config 0.000GB local 0.000GB myFirstDB 0.000GB (only appears if a document was inserted)
Listing All Collections in the Current Database
show collections
products users
Intuition Check
Q: Can you insert data into a collection before creating the database?
A: Yes! MongoDB automatically creates the database and the collection for you as soon as you insert data.
Summary
use dbName
switches or creates a databasedb.collection.insertOne()
creates the collection (and database) implicitlydb.createCollection("name")
explicitly creates a collectionshow dbs
andshow collections
help you view the structure
In the next lesson, we will learn how to insert multiple documents and understand how data is stored in MongoDB collections.