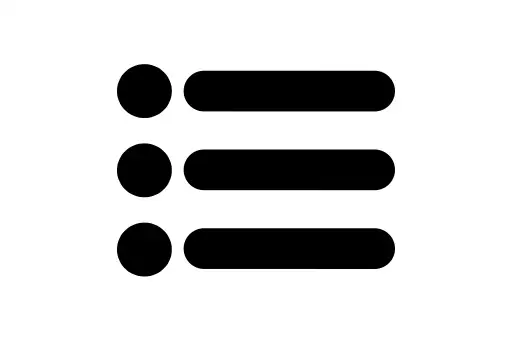
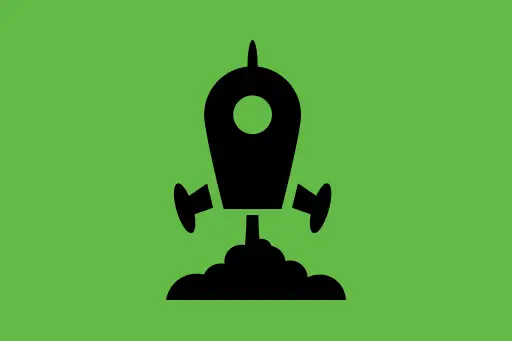
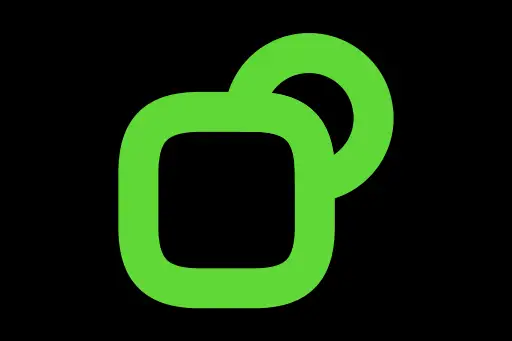
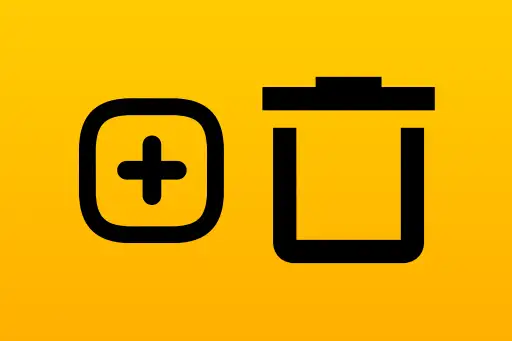
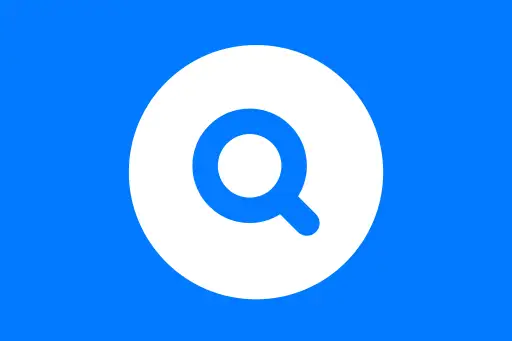
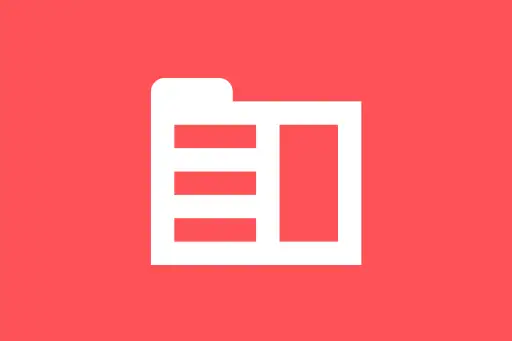
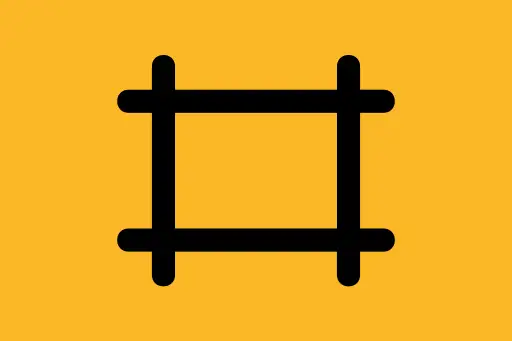
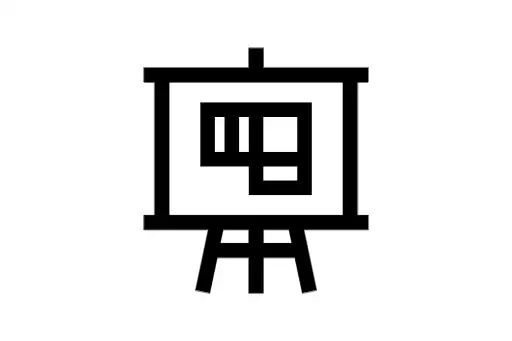
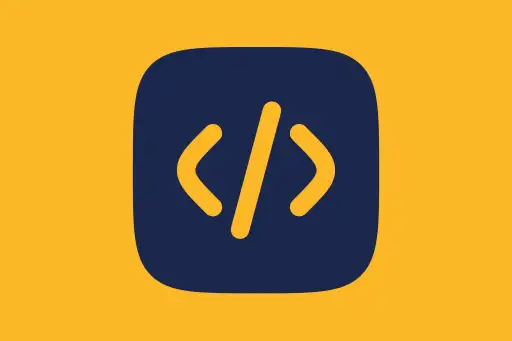
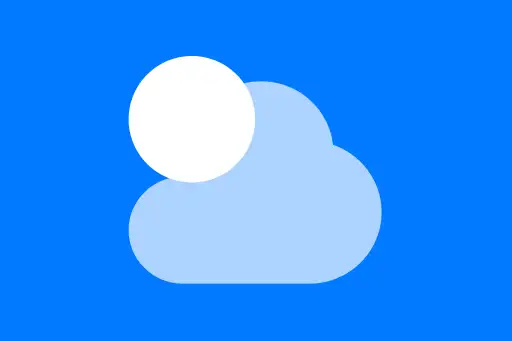
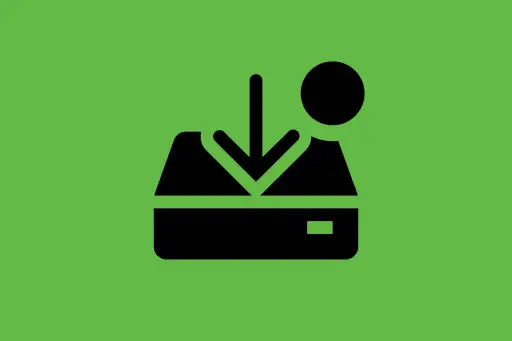
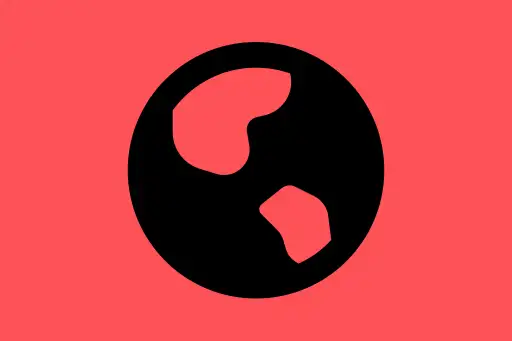
Connecting MongoDB Atlas to Local Applications
Next Topic ⮕Backing Up MongoDB using mongodump
Connecting MongoDB Atlas to Local Applications
MongoDB Atlas is a cloud-based database service that allows you to host MongoDB clusters with scalability, monitoring, and security built-in. To use it in your local development environment, you need to connect your application using the connection string provided by Atlas.
Step 1: Create a MongoDB Atlas Cluster
- Go to https://cloud.mongodb.com
- Create a free account or log in
- Click "Build a Cluster" → Choose "Shared" (Free tier)
- Choose a region (preferably close to your location)
- Name your cluster (or use default) and click "Create Cluster"
Step 2: Create a Database User
Once the cluster is created, go to "Database Access" → "Add New Database User".
- Set a username and password
- Give the user role:
Read and write to any database
- Click "Add User"
Step 3: Whitelist Your IP Address
Under "Network Access", click "Add IP Address" → Add your current IP (or use 0.0.0.0
to allow all for testing purposes).
Step 4: Get the Connection String
Go to "Database" → "Connect" → "Connect your application".
- Copy the connection string (looks like
mongodb+srv://<user>:<password>@cluster0.mongodb.net/?retryWrites=true&w=majority
)
Replace <user>
and <password>
with your actual credentials.
Example 1: Connect using Node.js and Mongoose
Install Mongoose:
npm install mongoose
Sample code:
// file: connect.js
const mongoose = require('mongoose');
const uri = "mongodb+srv://yourUsername:yourPassword@cluster0.mongodb.net/test?retryWrites=true&w=majority";
mongoose.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => {
console.log("MongoDB connected successfully!");
})
.catch((err) => {
console.error("MongoDB connection error:", err);
});
MongoDB connected successfully!
Explanation: You use Mongoose to connect to the Atlas cluster using the provided URI. The useNewUrlParser
and useUnifiedTopology
options are recommended for compatibility and stability.
Example 2: Connect using Python and PyMongo
Install PyMongo:
pip install pymongo
Sample code:
# file: connect.py
from pymongo import MongoClient
uri = "mongodb+srv://yourUsername:yourPassword@cluster0.mongodb.net/?retryWrites=true&w=majority"
client = MongoClient(uri)
# Access a test database
db = client.test
print("Collections in test DB:", db.list_collection_names())
Collections in test DB: []
Explanation: We use MongoClient
from PyMongo to connect. The db.list_collection_names()
lists existing collections in the test
database.
Intuition Check
Q: Why do we need to whitelist IP addresses on MongoDB Atlas?
A: For security. MongoDB Atlas restricts access only to known IP addresses to prevent unauthorized connections.
Q: Can I use this URI in production?
A: Yes, but you should store credentials in environment variables or use secrets management tools — never hard-code sensitive credentials in production apps.
Best Practices
- Use environment variables to store connection URIs
- Regularly rotate database credentials
- Limit user privileges — avoid admin roles unless necessary
Summary
Connecting your MongoDB Atlas cluster to local applications is straightforward once you configure users, whitelist IPs, and use the provided URI. You can use Node.js (Mongoose) or Python (PyMongo) depending on your stack.
In the next topic, we’ll learn how to build and run real-world applications using this connection.