





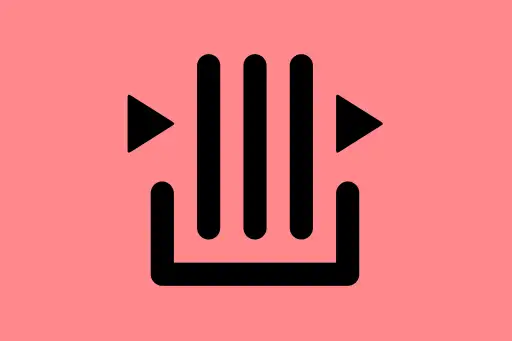
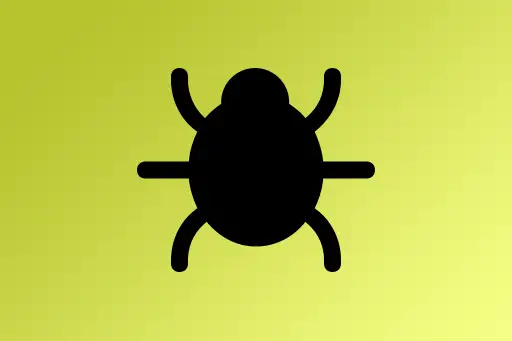
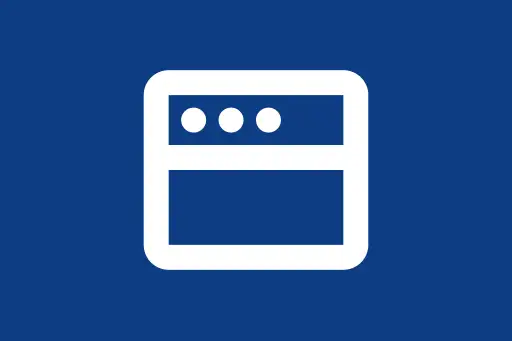
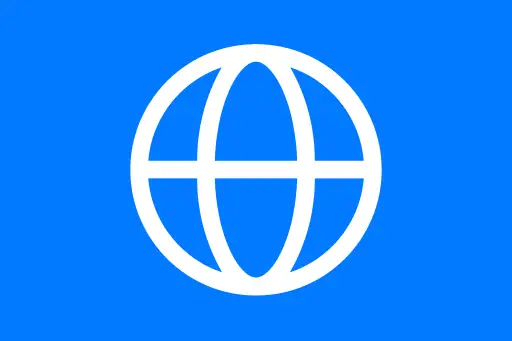
Understanding JavaScript Timers
Next Topic ⮕JavaScript Form Validation - Validate User Input with Pure JS
What are Timers in JavaScript?
JavaScript provides built-in timer functions that allow you to execute code after a delay or repeatedly at fixed intervals. These are especially useful when you want to delay actions, run animations, or execute repeated tasks.
setTimeout(function, delay)
– Runs a function once after the delay (in milliseconds).setInterval(function, interval)
– Runs a function repeatedly, every interval (in milliseconds).clearTimeout(id)
andclearInterval(id)
– Stop the timers created by the above functions.
Using setTimeout
Let’s begin with a simple example where we print a message after 2 seconds:
console.log("Timer started");
setTimeout(function () {
console.log("2 seconds passed!");
}, 2000);
Timer started 2 seconds passed!
How does setTimeout
work?
setTimeout
schedules the function to run after the delay but does not block the rest of the code. It's non-blocking, meaning the program continues to run.
Question:
What happens if you use setTimeout
with 0 milliseconds delay?
Answer:
Even if the delay is 0, the function goes to the end of the task queue and runs after the current execution stack is complete.
Using clearTimeout
to Cancel a Timeout
Sometimes you may want to cancel the scheduled timeout before it executes. Here's how:
let timerId = setTimeout(() => {
console.log("This will not run");
}, 3000);
clearTimeout(timerId);
console.log("Timer cancelled");
Timer cancelled
Using setInterval
setInterval
is used to run a function repeatedly at a given interval. Example:
let count = 0;
let intervalId = setInterval(() => {
count++;
console.log("Interval count:", count);
if (count === 3) {
clearInterval(intervalId);
console.log("Interval cleared");
}
}, 1000);
Interval count: 1 Interval count: 2 Interval count: 3 Interval cleared
Common Mistakes and Best Practices
- Avoid nesting too many timers as it makes code harder to debug.
- Always clear intervals if no longer needed to prevent memory leaks.
- Use named functions instead of anonymous for better readability and reuse.
When Should You Use Timers?
- Delaying UI updates (e.g., loading animations).
- Polling a server at intervals.
- Retrying a failed operation after some delay.
Conclusion
Timers in JavaScript are powerful tools for handling asynchronous actions. Whether you want to delay execution or run something repeatedly, setTimeout
and setInterval
give you the control you need.
Comments
Loading comments...