





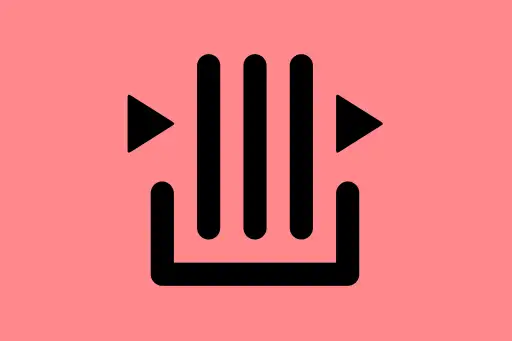
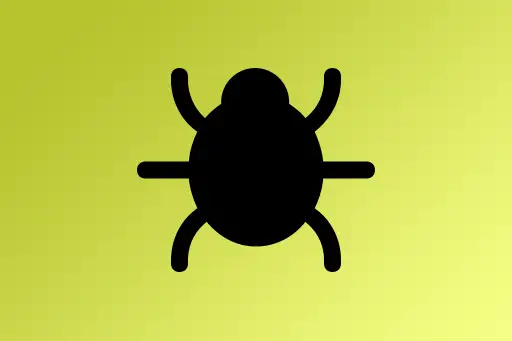
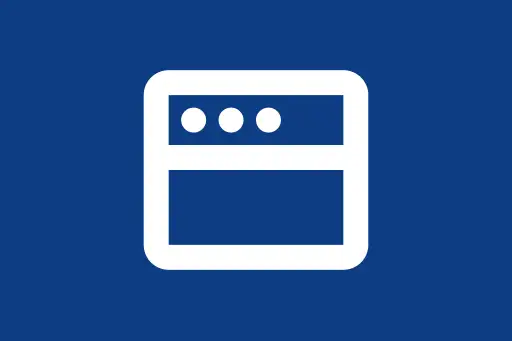
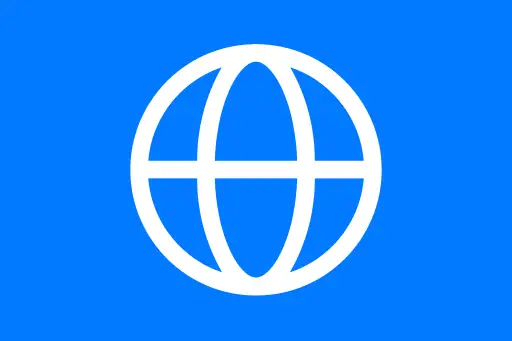
Project 3:
Simple Quiz Application
Next Topic ⮕JavaScript Budget Tracker Project - Expense Manager with Pure JavaScript
Overview
This project demonstrates how to build a simple quiz application using pure JavaScript. It runs entirely in the browser console or Node.js environment and does not depend on HTML or browser DOM. It's perfect for beginners learning how to use arrays, functions, conditionals, loops, and basic input/output operations.
Objective
Create a JavaScript-based quiz program that:
- Asks multiple-choice questions from a predefined list
- Accepts user input via prompt (for browser) or readline (for Node.js)
- Calculates and shows the final score
- Provides feedback on correct and incorrect answers
Step-by-Step Development
Step 1: Define Quiz Questions
Start with an array of objects to represent each quiz question.
const questions = [
{
question: "Which language runs in a web browser?",
options: ["Java", "C", "Python", "JavaScript"],
answer: "JavaScript"
},
{
question: "What does CSS stand for?",
options: ["Central Style Sheets", "Cascading Style Sheets", "Cascading Simple Sheets", "Cars SUVs Sailboats"],
answer: "Cascading Style Sheets"
},
{
question: "What year was JavaScript created?",
options: ["1996", "1995", "1994", "none of the above"],
answer: "1995"
}
];
Why use objects to store questions?
Objects let us bundle related data (question, options, answer) together. This makes it easy to loop over them and access the needed information cleanly.
Step 2: Ask Questions and Get User Input
In Node.js, use readline
to read input from the terminal.
const readline = require('readline').createInterface({
input: process.stdin,
output: process.stdout
});
let score = 0;
let current = 0;
function askQuestion() {
const q = questions[current];
console.log("\n" + q.question);
q.options.forEach((opt, idx) => {
console.log(`${idx + 1}. ${opt}`);
});
readline.question("Your answer (1-4): ", (userInput) => {
const chosen = q.options[parseInt(userInput) - 1];
if (chosen === q.answer) {
console.log("Correct!");
score++;
} else {
console.log(`❌ Wrong! The correct answer is: ${q.answer}`);
}
current++;
if (current < questions.length) {
askQuestion();
} else {
console.log(`\n🎉 Quiz Finished! Your Score: ${score}/${questions.length}\n`);
readline.close();
}
});
}
askQuestion();
Which language runs in a web browser? 1. Java 2. C 3. Python 4. JavaScript Your answer (1-4): 4 Correct! ... 🎉 Quiz Finished! Your Score: 2/3
How does the input handling work?
The readline
module listens for input in the terminal. Each time the user answers a question, we compare their choice to the correct answer and update the score accordingly.
Step 3: Enhancements (Optional)
- Randomize the order of questions
- Add a timer for each question
- Track incorrect questions for review
- Support replaying the quiz
Final Thoughts
This project teaches important JavaScript concepts in a real-world context. By using arrays, loops, input/output handling, and basic conditional logic, you can build a complete functional application—all in JavaScript.
Questions to Reinforce Learning
Q1: Why do we use parseInt()
on user input?
A: Because input from readline
is always a string. We convert it to a number so we can use it to index the options
array.
Q2: What would happen if the user enters a number out of range (e.g., 5)?
A: The chosen
variable would be undefined
, and the answer comparison would fail. We can add a validation to handle this gracefully.
Q3: Can this code run in a browser?
A: No. The readline
module is Node.js specific. For browser-based quizzes, you'd use prompt()
or create UI with HTML and handle events with JavaScript.
Key Takeaways
- Practice using objects and arrays to structure data
- Learn conditional checks and comparison
- Understand the basics of user interaction in terminal environments
Comments
Loading comments...