





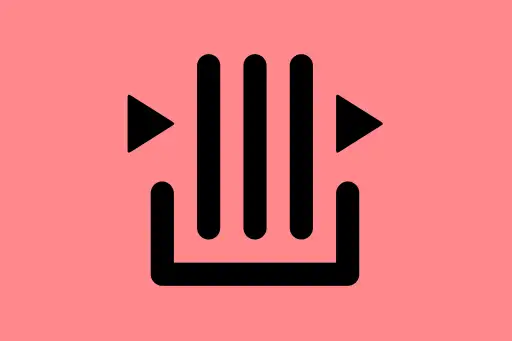
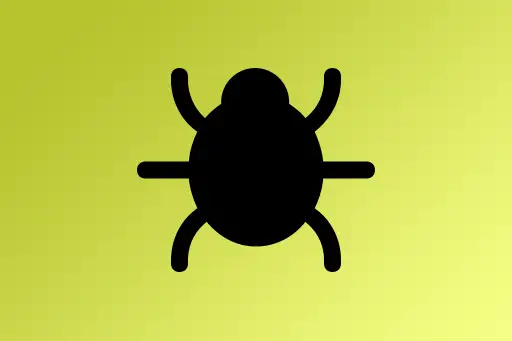
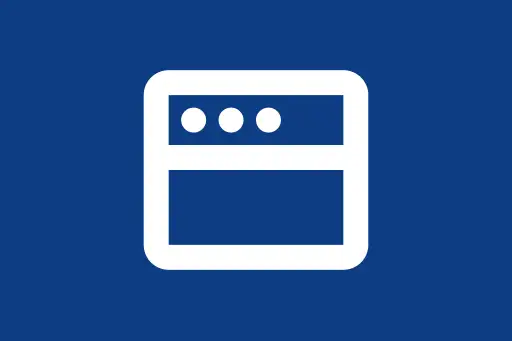
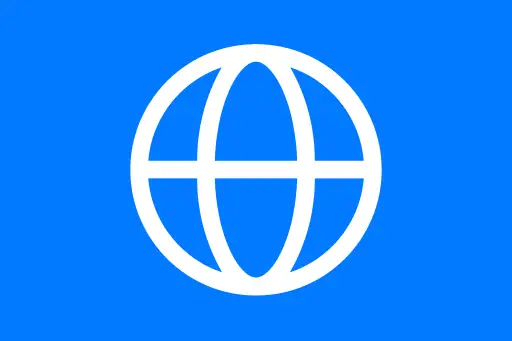
Project 2:
Weather App using API
Next Topic ⮕Simple Quiz Application in JavaScript
Overview
This project will help you build a Weather App using pure JavaScript and an external API. We will use the OpenWeatherMap API to fetch real-time weather data based on the user's city input.
What You'll Learn
- How to make HTTP requests using
fetch()
- How to parse and handle JSON data
- How to build a CLI or console-based tool using JavaScript
- How to use environment variables to store API keys (Node.js only)
Step 1: Get API Access
Sign up at OpenWeatherMap and get your free API key.
Step 2: Understand the API Request
The API URL to get weather for a city looks like this:
https://api.openweathermap.org/data/2.5/weather?q=London&appid=YOUR_API_KEY&units=metric
Question:
What are the parameters in the API URL?
Answer: q
is the city name, appid
is your API key, and units=metric
ensures temperature is in Celsius.
Step 3: Write JavaScript Code (Browser Console)
This version runs directly in the browser console. Replace YOUR_API_KEY
with your actual key.
const city = "Delhi";
const apiKey = "YOUR_API_KEY"; // Replace with your real API key
fetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`)
.then(response => {
if (!response.ok) {
throw new Error("City not found");
}
return response.json();
})
.then(data => {
console.log(`Weather in ${data.name}:`);
console.log(`Temperature: ${data.main.temp}°C`);
console.log(`Weather: ${data.weather[0].description}`);
console.log(`Humidity: ${data.main.humidity}%`);
console.log(`Wind Speed: ${data.wind.speed} m/s`);
})
.catch(error => {
console.error("Error fetching weather data:", error.message);
});
Weather in Delhi: Temperature: 32°C Weather: clear sky Humidity: 28% Wind Speed: 3.6 m/s
Code Description
fetch()
is used to send a GET request to the weather API.- We check
response.ok
to handle invalid cities or errors. data.main.temp
,data.weather[0].description
etc. extract key weather metrics.- Error handling with
.catch()
ensures graceful failure.
Question:
What happens if the user enters an invalid city name?
Answer: The API returns a 404 status, and our code throws an error with the message "City not found".
Step 4: Run in Node.js (with Readline)
This version lets users enter city names via command line:
// weather.js
const readline = require("readline");
const https = require("https");
const apiKey = "YOUR_API_KEY"; // Replace with your key
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question("Enter a city name: ", function(city) {
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`;
https.get(url, (res) => {
let data = "";
res.on("data", chunk => data += chunk);
res.on("end", () => {
const weather = JSON.parse(data);
if (weather.cod !== 200) {
console.error("Error:", weather.message);
} else {
console.log(`Weather in ${weather.name}:`);
console.log(`Temperature: ${weather.main.temp}°C`);
console.log(`Condition: ${weather.weather[0].description}`);
}
rl.close();
});
}).on("error", (e) => {
console.error("Failed to fetch:", e.message);
rl.close();
});
});
Enter a city name: Paris Weather in Paris: Temperature: 21°C Condition: few clouds
Best Practices
- Always validate API responses before accessing nested properties.
- Store API keys securely (e.g., in
.env
for real-world apps). - Handle network errors and empty inputs gracefully.
Wrap-up
This project helped you connect JavaScript to a real-world weather API. You now know how to fetch, parse, and display data dynamically using modern JavaScript techniques.
Comments
Loading comments...