





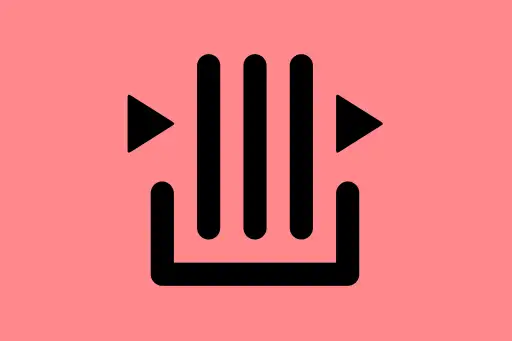
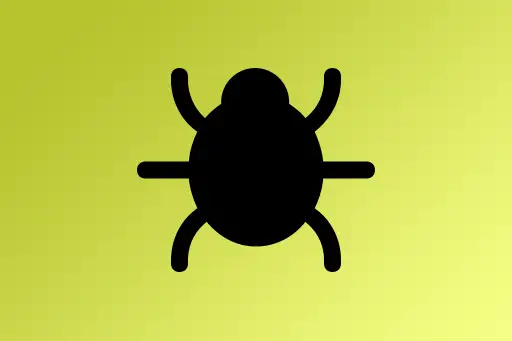
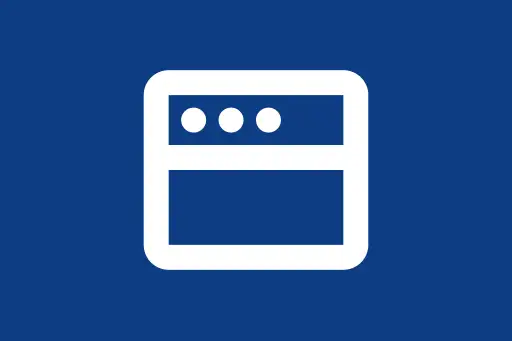
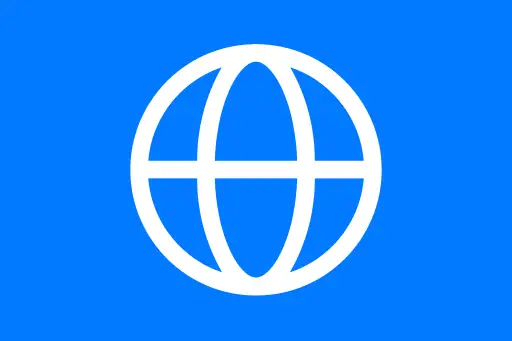
Modules in JavaScript
import & export
Next Topic ⮕JavaScript Callbacks and Callback Hell
Introduction to JavaScript Modules
Modules help in breaking a JavaScript codebase into reusable and manageable pieces. Before ES6, developers used patterns like IIFEs or libraries like RequireJS to emulate modularity. ES6 introduced native support for modules through export
and import
.
Why Use Modules?
- Helps organize code into separate files.
- Encourages reusability and separation of concerns.
- Improves maintainability and scalability of applications.
Types of Exports
- Named Export - Export multiple values by name.
- Default Export - Export a single value as the default.
Using Named Exports
Let's create a file mathUtils.js
and export multiple functions:
// mathUtils.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
Now, import them into another file:
// app.js
import { add, subtract } from './mathUtils.js';
console.log(add(10, 5));
console.log(subtract(10, 5));
15 5
Using Default Exports
Suppose a module has only one primary export, you can use export default
:
// logger.js
export default function log(message) {
console.log('Log:', message);
}
Importing a default export does not require curly braces:
// app.js
import log from './logger.js';
log('Modules are awesome!');
Log: Modules are awesome!
Can You Combine Named and Default Exports?
Yes, a file can contain both:
// helpers.js
export default function greet(name) {
return `Hello, ${name}`;
}
export const PI = 3.14;
// app.js
import greet, { PI } from './helpers.js';
console.log(greet('JavaScript'));
console.log(PI);
Hello, JavaScript 3.14
Running JavaScript Modules
If using in Node.js, ensure the file extension is .mjs
or add "type": "module"
to your package.json
.
node app.mjs
If using in browser, use type="module"
in your script tag (for completeness, but not needed here).
Common Questions
Can I rename an imported function?
Yes, using as
keyword:
import { add as sum } from './mathUtils.js';
console.log(sum(2, 3));
5
Can I import everything?
Yes, using * as
syntax:
import * as math from './mathUtils.js';
console.log(math.add(1, 2));
console.log(math.subtract(5, 3));
3 2
Summary
export
lets you share functions, variables, or objects.import
allows you to bring those into another file.- Use default export when the module has a single main value.
- Use named exports to share multiple utilities.
Mastering modules will help you write cleaner, modular, and maintainable code for large applications.
Comments
Loading comments...