





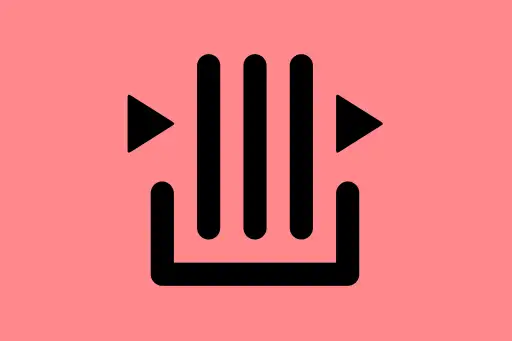
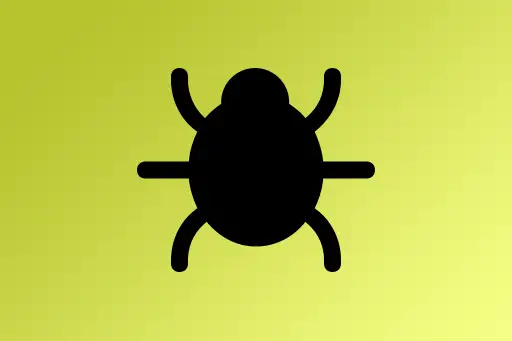
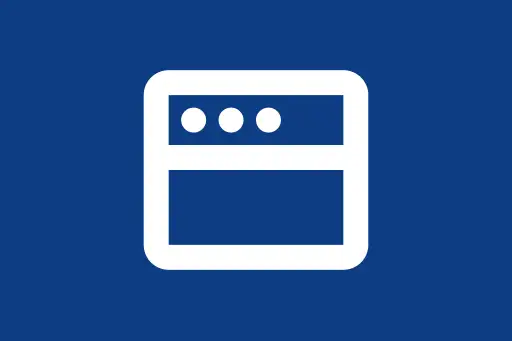
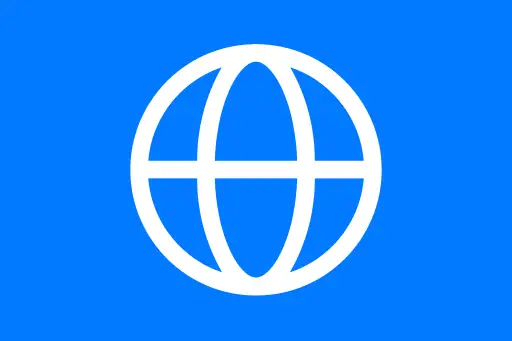
JavaScript
Form Validation
Next Topic ⮕JavaScript DOM Events and Event Delegation
Introduction to Form Validation
Form validation ensures that users provide valid and expected input before the data is processed. In real-world applications, it's important to prevent incorrect or malicious data from being submitted.
There are two types of validations:
- Client-side validation – Done using JavaScript before submission
- Server-side validation – Done on the server after submission
In this topic, we focus on pure JavaScript client-side validation using functions that simulate form input and logic—no HTML needed.
Example 1: Basic Required Field Check
This example demonstrates checking if the user has entered any value.
// Simulated input
const userInput = "";
// Validation function
function validateRequired(input) {
if (input.trim() === "") {
return "This field is required.";
} else {
return "Input accepted.";
}
}
console.log(validateRequired(userInput));
This field is required.
Example 2: Validate Email Format
Let’s validate whether an input is a valid email using a basic regex.
// Simulated input
const emailInput = "user@example.com";
// Validation function
function validateEmail(email) {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(email) ? "Valid email." : "Invalid email format.";
}
console.log(validateEmail(emailInput));
Valid email.
Question:
Why use regex
instead of just checking for @
and .
?
Answer: Regex ensures proper structure, avoiding common mistakes like @.
, user@@example
, or user@.com
.
Example 3: Password Length Check
Passwords should have a minimum number of characters. Here’s a validation function for that.
// Simulated input
const password = "pass123";
// Validation function
function validatePasswordLength(pwd) {
return pwd.length >= 8
? "Password length is acceptable."
: "Password must be at least 8 characters.";
}
console.log(validatePasswordLength(password));
Password must be at least 8 characters.
Example 4: Confirm Password Match
This example checks if the confirmation password matches the original password.
const password = "secret123";
const confirmPassword = "secret1234";
function validatePasswordMatch(pwd, confirmPwd) {
return pwd === confirmPwd
? "Passwords match."
: "Passwords do not match.";
}
console.log(validatePasswordMatch(password, confirmPassword));
Passwords do not match.
Example 5: Full Form Validation Simulation
This example combines all the above checks into one function to simulate a form submission check.
const formData = {
name: "John",
email: "john.doe@domain.com",
password: "pass123",
confirmPassword: "pass123"
};
function validateForm(data) {
const errors = [];
if (data.name.trim() === "") errors.push("Name is required.");
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(data.email)) errors.push("Invalid email format.");
if (data.password.length < 8)
errors.push("Password must be at least 8 characters.");
if (data.password !== data.confirmPassword)
errors.push("Passwords do not match.");
return errors.length > 0 ? errors : ["Form submitted successfully!"];
}
console.log(validateForm(formData));
[ "Password must be at least 8 characters." ]
Summary
- Validation ensures correct input before processing.
- Use
trim()
to clean strings and check for empty values. - Regex helps validate structured data like emails.
- Breaking validation into small reusable functions is a good practice.
Points to Remember
- Always validate on the client-side for better UX.
- Always validate again on the server for security.
- Regex is powerful but must be used carefully.
- JavaScript validations can be tested even in the console or Node.js.
Comments
Loading comments...