





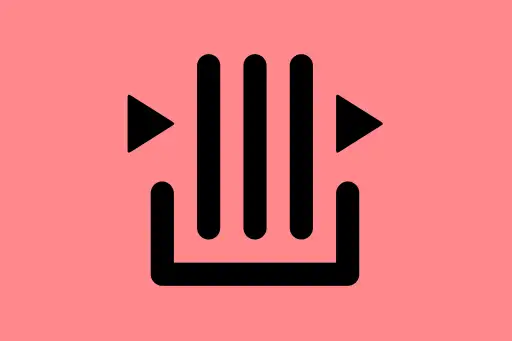
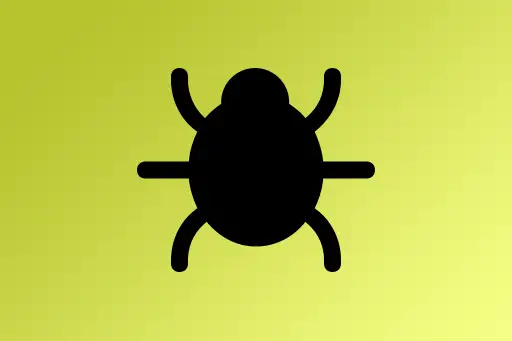
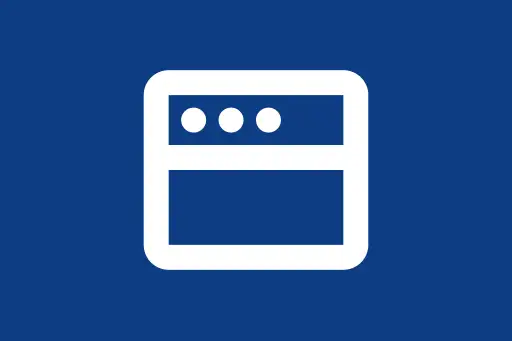
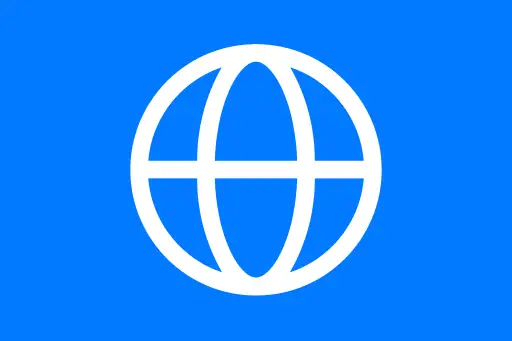
Console API and Debugging in Browser
JavaScript Debugging Tools
Understanding the Console API
The console
object in JavaScript provides access to the browser's debugging console. It includes methods that help developers output messages, warnings, errors, and even structured data like tables.
1. console.log()
This is the most commonly used method to print messages or variables to the console.
let user = "Alice";
console.log("Hello", user); // Outputs a string and a variable
Hello Alice
2. console.error()
Use this method to display error messages. It helps you highlight where something went wrong in your code.
console.error("Something went wrong!");
Something went wrong!
3. console.warn()
This displays a warning message without stopping the script execution. It’s useful for highlighting potential issues.
console.warn("This might be deprecated soon.");
This might be deprecated soon.
4. console.table()
Displays tabular data in a neat table format. Useful when debugging arrays of objects.
let users = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 }
];
console.table(users);
(index) | name | age --------------------- 0 | Alice | 25 1 | Bob | 30
5. console.group() and console.groupEnd()
These methods group related messages together, making the console output cleaner and more readable.
console.group("User Info");
console.log("Name: Alice");
console.log("Age: 25");
console.groupEnd();
▶ User Info Name: Alice Age: 25
6. console.time() and console.timeEnd()
Measure the time taken by a block of code to execute.
console.time("loop");
for (let i = 0; i < 1000000; i++) {}
console.timeEnd("loop");
loop: 2.345ms
Debugging Using Browser Tools
Every modern browser comes with built-in developer tools (DevTools). Press F12
or Ctrl+Shift+I
to open it. Here's how to debug effectively:
1. Setting Breakpoints
Breakpoints pause the execution of JavaScript code at a specific line. This allows you to inspect variables and the call stack.
- Open DevTools and go to the Sources tab.
- Click on the line number to set a breakpoint.
- Reload the page or run the function to trigger the breakpoint.
2. Watch Expressions and Call Stack
In DevTools, you can add expressions to watch their values at runtime. The Call Stack shows the nested function calls that led to the current point in code.
3. Step Over, Step Into, and Step Out
These controls let you move through the code line-by-line while debugging:
- Step Over: Executes the next line.
- Step Into: Moves into a called function.
- Step Out: Exits the current function.
Common Beginner Question:
Q: Why use console.table()
instead of console.log()
?
A: console.table()
is ideal for debugging structured data like arrays of objects. It makes it easier to compare rows visually.
Pro Tip:
Use debugger;
in your JavaScript code to automatically trigger DevTools and pause execution.
function multiply(a, b) {
debugger;
return a * b;
}
multiply(2, 3);
This will pause the script when run in the browser and open DevTools if available.
Comments
Loading comments...