





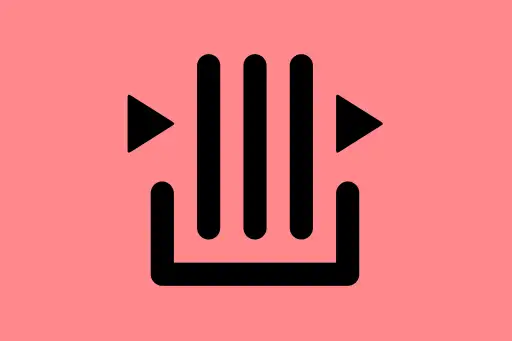
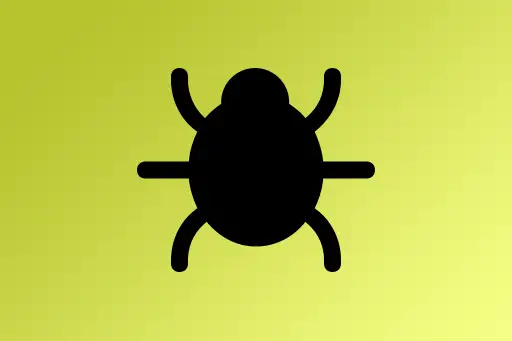
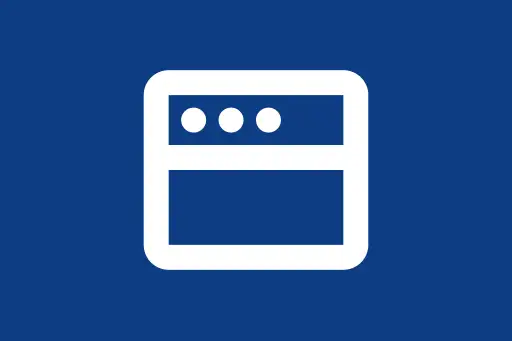
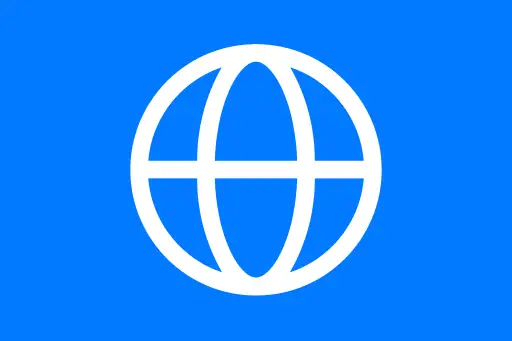
Getting Started with
JavaScript Browser APIs
Next Topic ⮕Local Storage in JavaScript - Store and Retrieve Data
What are Browser APIs?
Browser APIs (Application Programming Interfaces) are built-in features provided by modern web browsers that allow JavaScript to interact with the browser environment and perform specific tasks such as manipulating the DOM, storing data, handling events, or fetching data from the internet.
These are not part of the core JavaScript language, but they extend JavaScript's capabilities when running in the browser.
Common Browser APIs
- Console API
- Alert/Confirm/Prompt APIs
- setTimeout and setInterval
- Local Storage and Session Storage
- Location and History APIs
- Navigator API
- Fetch API
1. Console API
The console
API is used for logging information and debugging.
console.log("This is a log message");
console.warn("This is a warning");
console.error("This is an error");
This is a log message This is a warning This is an error
2. Alert, Confirm, and Prompt
These APIs allow interaction with the user through dialog boxes.
// Alert example
alert("Welcome to the JavaScript course!");
// Confirm example
const isConfirmed = confirm("Do you want to continue?");
console.log("User confirmed:", isConfirmed);
// Prompt example
const name = prompt("What is your name?");
console.log("User entered:", name);
Question: What is the difference between alert
and prompt
?
Answer: alert()
shows a message; prompt()
shows a message *and* asks for user input.
3. Timers: setTimeout and setInterval
These are used to schedule code execution after a delay or repeatedly at intervals.
// setTimeout example
setTimeout(() => {
console.log("This runs after 2 seconds");
}, 2000);
// setInterval example
let count = 0;
const intervalId = setInterval(() => {
console.log("Repeating message:", ++count);
if (count === 3) clearInterval(intervalId);
}, 1000);
This runs after 2 seconds Repeating message: 1 Repeating message: 2 Repeating message: 3
4. Local Storage API
localStorage
allows you to store key-value pairs in the browser that persist even after refreshing.
// Storing data
localStorage.setItem("username", "john_doe");
// Retrieving data
const user = localStorage.getItem("username");
console.log("Username from localStorage:", user);
// Removing data
localStorage.removeItem("username");
Question: Is data stored using localStorage
cleared when the page is refreshed?
Answer: No, localStorage
data persists until explicitly removed.
5. Location API
This API provides information about the current URL and allows redirection.
console.log("Current URL:", window.location.href);
console.log("Hostname:", window.location.hostname);
console.log("Pathname:", window.location.pathname);
// Redirecting to another URL
// window.location.href = "https://www.google.com";
Note: Redirection line is commented out to avoid unintended navigation during testing.
6. Navigator API
The navigator
object contains information about the browser.
console.log("Browser name:", navigator.appName);
console.log("Browser version:", navigator.appVersion);
console.log("User agent:", navigator.userAgent);
console.log("Online status:", navigator.onLine);
Output: Depends on the browser you are using.
7. Fetch API
Used to make HTTP requests from the browser.
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then(response => response.json())
.then(data => console.log("Fetched data:", data))
.catch(error => console.error("Error fetching data:", error));
Output: A JSON object with todo information.
Summary
- Browser APIs extend JavaScript's power inside the browser environment.
- You can access UI elements, manage data storage, communicate over the network, and more.
- They are easy to use and mostly work without any external libraries.
Practice Questions
- How does
localStorage
differ fromsessionStorage
? - What is the purpose of
setTimeout
? - How do you handle errors in a fetch request?