





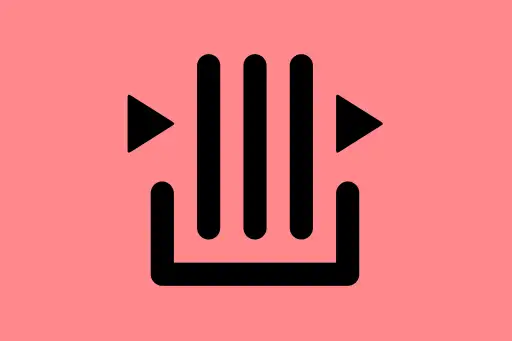
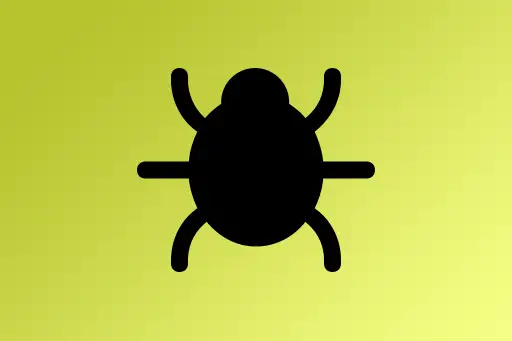
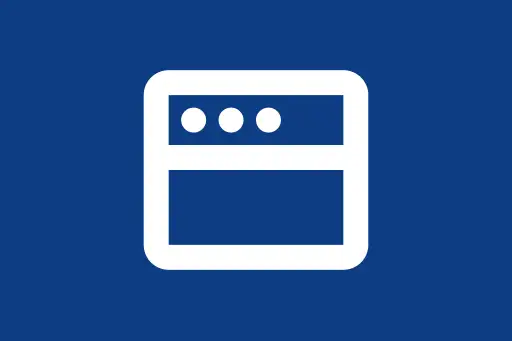
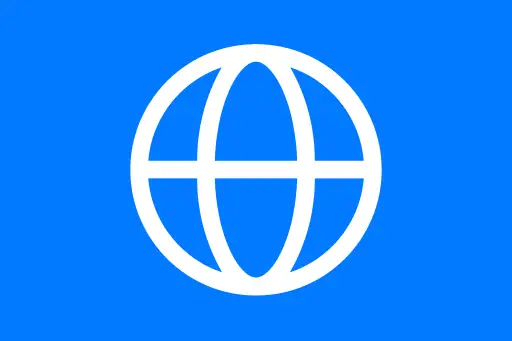
Arrow Functions in JavaScript
ES6 Syntax, Scope, and Usage
Introduction to Arrow Functions
Arrow functions, introduced in ES6, are a modern way to write functions in JavaScript. They are shorter, often more intuitive, and come with lexical this
binding—meaning they inherit the this
value from their surrounding context. In this tutorial, we'll dive deep into how they work, where to use them, and where they may surprise you.
Basic Syntax of Arrow Functions
The syntax of an arrow function is concise and clean:
const add = (a, b) => {
return a + b;
};
This is equivalent to a traditional function like:
function add(a, b) {
return a + b;
}
Single Expression Shorthand
If your function has only one expression and you want to return it, you can omit the return
keyword and the curly braces:
const multiply = (x, y) => x * y;
console.log(multiply(3, 4)); // 12
Arrow Function with One Parameter
If the arrow function takes only one parameter, parentheses are optional:
const square = x => x * x;
console.log(square(5)); // 25
Returning an Object
To return an object literal, wrap it in parentheses to avoid confusion with the function block:
const getUser = () => ({ name: "Alice", age: 30 });
console.log(getUser()); // { name: "Alice", age: 30 }
Lexical this
in Arrow Functions
The biggest shift with arrow functions is their handling of this
. Unlike regular functions, arrow functions do not bind their own this
. Instead, they inherit it from the parent scope.
function Timer() {
this.seconds = 0;
setInterval(() => {
this.seconds++;
console.log(this.seconds);
}, 1000);
}
new Timer();
// Logs incrementing seconds: 1, 2, 3, ...
If we had used a regular function inside setInterval
, this
would be undefined or refer to the global object, breaking the logic.
Arrow Functions Cannot Be Used As Constructors
Arrow functions are not meant to be used with new
keyword. They lack a [[Construct]]
method and will throw an error:
const Person = (name) => {
this.name = name;
};
const p = new Person("Bob"); // TypeError
No arguments
Object in Arrow Functions
Arrow functions do not have their own arguments
object. If you try to use it, you’ll get the arguments from the outer function scope.
const showArgs = () => {
console.log(arguments);
};
showArgs(1, 2); // ReferenceError: arguments is not defined
If you need arguments
, use a regular function.
Use Cases: Where Arrow Functions Shine
- Callback functions like
map
,filter
,reduce
,forEach
- Event handling in frameworks like React
- Simple utility functions with concise logic
const nums = [1, 2, 3, 4];
const doubled = nums.map(n => n * 2);
console.log(doubled); // [2, 4, 6, 8]
When to Avoid Arrow Functions
- When you need dynamic
this
(e.g., in event handlers that refer to the element) - When you need
arguments
object - When defining object methods that rely on
this
const user = {
name: "Ella",
greet: () => {
console.log("Hi, " + this.name);
}
};
user.greet(); // Hi, undefined
Here, this.name
is undefined because this
is not bound to the object.
Conclusion
Arrow functions offer a sleek and powerful way to write JavaScript. They simplify syntax and bring clarity in many scenarios—especially where lexical scoping of this
is beneficial. However, they are not a complete replacement for regular functions. Understanding where they fit and where they don’t will elevate your JavaScript fluency.