
- 1Find Maximum and Minimum in Array using Loop
- 2Find Second Largest in Array
- 3Find Second Smallest in Array
- 4Reverse Array using Two Pointers
- 5Check if Array is Sorted
- 6Remove Duplicates from Sorted Array
- 7Left Rotate an Array by One Place
- 8Left Rotate an Array by K Places
- 9Move Zeroes in Array to End
- 10Linear Search in Array
- 11Union of Two Arrays
- 12Find Missing Number in Array
- 13Max Consecutive Ones in Array
- 14Find Kth Smallest Element
- 15Longest Subarray with Given Sum (Positives)
- 16Longest Subarray with Given Sum (Positives and Negatives)
- 17Find Majority Element in Array (more than n/2 times)
- 18Find Majority Element in Array (more than n/3 times)
- 19Maximum Subarray Sum using Kadane's Algorithm
- 20Print Subarray with Maximum Sum
- 21Stock Buy and Sell
- 22Rearrange Array Alternating Positive and Negative Elements
- 23Next Permutation of Array
- 24Leaders in an Array
- 25Longest Consecutive Sequence in Array
- 26Count Subarrays with Given Sum
- 27Sort an Array of 0s, 1s, and 2s
- 28Two Sum Problem
- 29Three Sum Problem
- 304 Sum Problem
- 31Find Length of Largest Subarray with 0 Sum
- 32Find Maximum Product Subarray

- 1Binary Search in Array using Iteration
- 2Find Lower Bound in Sorted Array
- 3Find Upper Bound in Sorted Array
- 4Search Insert Position in Sorted Array (Lower Bound Approach)
- 5Floor and Ceil in Sorted Array
- 6First Occurrence in a Sorted Array
- 7Last Occurrence in a Sorted Array
- 8Count Occurrences in Sorted Array
- 9Search Element in a Rotated Sorted Array
- 10Search in Rotated Sorted Array with Duplicates
- 11Minimum in Rotated Sorted Array
- 12Find Rotation Count in Sorted Array
- 13Search Single Element in Sorted Array
- 14Find Peak Element in Array
- 15Square Root using Binary Search
- 16Nth Root of a Number using Binary Search
- 17Koko Eating Bananas
- 18Minimum Days to Make M Bouquets
- 19Find the Smallest Divisor Given a Threshold
- 20Capacity to Ship Packages within D Days
- 21Kth Missing Positive Number
- 22Aggressive Cows Problem
- 23Allocate Minimum Number of Pages
- 24Split Array - Minimize Largest Sum
- 25Painter's Partition Problem
- 26Minimize Maximum Distance Between Gas Stations
- 27Median of Two Sorted Arrays of Different Sizes
- 28K-th Element of Two Sorted Arrays
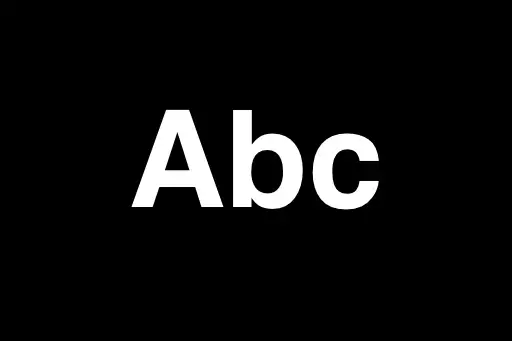
- 1Reverse Words in a String
- 2Find the Largest Odd Number in a Numeric String
- 3Find Longest Common Prefix in Array of Strings
- 4Find Longest Common Substring in Two Strings
- 5Check If Two Strings Are Isomorphic - Optimal HashMap Solution
- 6Check String Rotation using Concatenation - Optimal Approach
- 7Check if Two Strings Are Anagrams - Optimal Approach
- 8Sort Characters by Frequency - Optimal HashMap and Heap Approach
- 9Find Longest Palindromic Substring - Dynamic Programming Approach
- 10Find Longest Palindromic Substring Without Dynamic Programming
- 11Remove Outermost Parentheses in String
- 12Find Maximum Nesting Depth of Parentheses - Optimal Stack-Free Solution
- 13Convert Roman Numerals to Integer - Efficient Approach
- 14Convert Integer to Roman Numeral - Step-by-Step for Beginners
- 15Implement Atoi - Convert String to Integer in Java
- 16Count Number of Substrings in a String - Explanation with Formula
- 17Edit Distance Problem
- 18Calculate Sum of Beauty of All Substrings - Optimal Approach
- 19Reverse Each Word in a String - Optimal Approach

- 1Check if i-th Bit is Set
- 2Check if a Number is Even/Odd
- 3Check if a Number is Power of 2
- 4Count Number of Set Bits
- 5Swap Two Numbers using XOR
- 6Divide Two Integers without using Multiplication, Division and Modulus Operator
- 7Count Number of Bits to Flip to Convert A to B
- 8Find the Number that Appears Odd Number of Times
- 9Power Set
- 10Find XOR of Numbers from L to R
- 11Prime Factors of a Number
- 12All Divisors of Number
- 13Sieve of Eratosthenes
- 14Find Prime Factorisation of a Number using Sieve
- 15Power(n, x)


- 1Preorder Traversal of a Binary Tree using Recursion
- 2Preorder Traversal of a Binary Tree using Iteration
- 3Inorder Traversal of a Binary Tree using Recursion
- 4Inorder Traversal of a Binary Tree using Iteration
- 5Postorder Traversal of a Binary Tree Using Recursion
- 6Postorder Traversal of a Binary Tree using Iteration
- 7Level Order Traversal of a Binary Tree using Recursion
- 8Level Order Traversal of a Binary Tree using Iteration
- 9Reverse Level Order Traversal of a Binary Tree using Iteration
- 10Reverse Level Order Traversal of a Binary Tree using Recursion
- 11Find Height of a Binary Tree
- 12Find Diameter of a Binary Tree
- 13Find Mirror of a Binary Tree
- 14Left View of a Binary Tree
- 15Right View of a Binary Tree
- 16Top View of a Binary Tree
- 17Bottom View of a Binary Tree
- 18Zigzag Traversal of a Binary Tree
- 19Check if a Binary Tree is Balanced
- 20Diagonal Traversal of a Binary Tree
- 21Boundary Traversal of a Binary Tree
- 22Construct a Binary Tree from a String with Bracket Representation
- 23Convert a Binary Tree into a Doubly Linked List
- 24Convert a Binary Tree into a Sum Tree
- 25Find Minimum Swaps Required to Convert a Binary Tree into a BST
- 26Check if a Binary Tree is a Sum Tree
- 27Check if All Leaf Nodes are at the Same Level in a Binary Tree
- 28Lowest Common Ancestor (LCA) in a Binary Tree
- 29Solve the Tree Isomorphism Problem
- 30Check if a Binary Tree Contains Duplicate Subtrees of Size 2 or More
- 31Check if Two Binary Trees are Mirror Images
- 32Calculate the Sum of Nodes on the Longest Path from Root to Leaf in a Binary Tree
- 33Print All Paths in a Binary Tree with a Given Sum
- 34Find the Distance Between Two Nodes in a Binary Tree
- 35Find the kth Ancestor of a Node in a Binary Tree
- 36Find All Duplicate Subtrees in a Binary Tree

- 1Find a Value in a Binary Search Tree
- 2Delete a Node in a Binary Search Tree
- 3Find the Minimum Value in a Binary Search Tree
- 4Find the Maximum Value in a Binary Search Tree
- 5Find the Inorder Successor in a Binary Search Tree
- 6Find the Inorder Predecessor in a Binary Search Tree
- 7Check if a Binary Tree is a Binary Search Tree
- 8Find the Lowest Common Ancestor of Two Nodes in a Binary Search Tree
- 9Convert a Binary Tree into a Binary Search Tree
- 10Balance a Binary Search Tree
- 11Merge Two Binary Search Trees
- 12Find the kth Largest Element in a Binary Search Tree
- 13Find the kth Smallest Element in a Binary Search Tree
- 14Flatten a Binary Search Tree into a Sorted List

- 1Breadth-First Search in Graphs
- 2Depth-First Search in Graphs
- 3Number of Provinces in an Undirected Graph
- 4Connected Components in a Matrix
- 5Rotten Oranges Problem - BFS in Matrix
- 6Flood Fill Algorithm - Graph Based
- 7Detect Cycle in an Undirected Graph using DFS
- 8Detect Cycle in an Undirected Graph using BFS
- 9Distance of Nearest Cell Having 1 - Grid BFS
- 10Surrounded Regions in Matrix using Graph Traversal
- 11Number of Enclaves in Grid
- 12Word Ladder - Shortest Transformation using Graph
- 13Word Ladder II - All Shortest Transformation Sequences
- 14Number of Distinct Islands using DFS
- 15Check if a Graph is Bipartite using DFS
- 16Topological Sort Using DFS
- 17Topological Sort using Kahn's Algorithm
- 18Cycle Detection in Directed Graph using BFS
- 19Course Schedule - Task Ordering with Prerequisites
- 20Course Schedule 2 - Task Ordering Using Topological Sort
- 21Find Eventual Safe States in a Directed Graph
- 22Alien Dictionary Character Order
- 23Shortest Path in Undirected Graph with Unit Distance
- 24Shortest Path in DAG using Topological Sort
- 25Dijkstra's Algorithm Using Set - Shortest Path in Graph
- 26Dijkstra’s Algorithm Using Priority Queue
- 27Shortest Distance in a Binary Maze using BFS
- 28Path With Minimum Effort in Grid using Graphs
- 29Cheapest Flights Within K Stops - Graph Problem
- 30Number of Ways to Reach Destination in Shortest Time - Graph Problem
- 31Minimum Multiplications to Reach End - Graph BFS
- 32Bellman-Ford Algorithm for Shortest Paths
- 33Floyd Warshall Algorithm for All-Pairs Shortest Path
- 34Find the City With the Fewest Reachable Neighbours
- 35Minimum Spanning Tree in Graphs
- 36Prim's Algorithm for Minimum Spanning Tree
- 37Disjoint Set (Union-Find) with Union by Rank and Path Compression
- 38Kruskal's Algorithm - Minimum Spanning Tree
- 39Minimum Operations to Make Network Connected
- 40Most Stones Removed with Same Row or Column
- 41Accounts Merge Problem using Disjoint Set Union
- 42Number of Islands II - Online Queries using DSU
- 43Making a Large Island Using DSU
- 44Bridges in Graph using Tarjan's Algorithm
- 45Articulation Points in Graphs
- 46Strongly Connected Components using Kosaraju's Algorithm
Tree and Graph Traversal Techniques in DSA | BFS & DFS Explained
Tree and Graph Traversal — Core of DSA Navigation
- Traversal is the process of visiting nodes in a specific order.
- Used in tree/graph problems for search, validation, and processing.
- Common traversal methods: BFS (Breadth-First Search) and DFS (Depth-First Search).
What is Tree/Graph Traversal?
Traversal means visiting all nodes in a tree or graph structure, usually to perform operations like searching, pathfinding, or calculating values.
Traversal helps explore the structure systematically — whether we go deep (DFS) or wide (BFS) depends on the goal and constraints.
Types of Traversal
- Breadth-First Search (BFS) – Visit all neighbors level by level before going deeper.
- Depth-First Search (DFS) – Go deep into one path before backtracking.
- In trees: additional DFS types like Inorder, Preorder, Postorder.
1. Breadth-First Search (BFS)
What is BFS? Breadth-First Search (BFS) is a method used to traverse or search through a graph or tree. The main idea behind BFS is to start from a given node (often called the source or root) and then explore all its immediate neighbors (nodes directly connected to it). After visiting all neighbors at the current level, it moves to the next level of neighbors — hence the name breadth-first.
Why BFS is Important: BFS is useful when you need to find the shortest path in an unweighted graph, or when you want to visit all nodes level by level, like in a tree. It is also the basis for many real-world applications like GPS navigation systems, friend suggestion features on social media, and even solving puzzles or mazes.
How Does BFS Work?
BFS uses a queue data structure to keep track of the nodes to visit next. A queue follows the First-In-First-Out (FIFO) principle — just like a line at a ticket counter. This ensures that nodes are visited in the correct order: first those closest to the start node, then those further away.
Pseudocode
This pseudocode outlines the basic steps of BFS in a very readable way:
function BFS(graph, start):
create empty queue // This queue will keep track of nodes to visit
mark start as visited // We remember that we’ve already visited this node
enqueue start // Add the starting node to the queue
while queue not empty: // While there are still nodes to process
node = dequeue // Remove the front node from the queue
process(node) // Do something with this node (like print it)
for each neighbor of node: // Check each connected node (neighbor)
if not visited: // If we haven’t visited it yet
mark visited // Mark it as visited to avoid revisiting
enqueue neighbor // Add it to the queue to visit later
Step-by-step Example: Imagine a graph where Node A is connected to B and C, and B is connected to D.
- Start at A → visit A, enqueue B and C
- Visit B → enqueue D
- Visit C → no more new neighbors
- Visit D → end
The order of traversal: A → B → C → D
Example Use Cases:
- Shortest path in unweighted graphs: BFS ensures the shortest number of edges to reach a node.
- Level-order traversal in trees: Visit all nodes level by level (first level, then second, and so on).
- Web crawlers, friend recommendations: BFS helps find nearby pages/friends in a network.
Time and Space Complexity:
- Time Complexity: O(V + E)
You visit each Vertice (node) and each Edge once. So it's very efficient. - Space Complexity: O(V)
You store visited nodes and the queue — both grow with the number of vertices.
- BFS explores a graph/tree in layers (breadth-wise).
- It uses a queue to manage the order of node visits.
- Perfect when you want the shortest path or need to visit nodes level-by-level.
- Easy to implement and very common in real-world applications.
2. Depth-First Search (DFS)
What is DFS?
Depth-First Search (DFS) is a method used to traverse or explore a graph or tree structure. The main idea behind DFS is to go as deep as possible into a branch, visiting nodes, and only when you can’t go any further, you backtrack and explore another path.
How it works:
- You start from a selected node (in graphs) or the root (in trees).
- Visit the node and mark it as visited to avoid visiting it again (especially important in graphs).
- Then, for each unvisited neighbor, repeat the same process.
- This continues until all possible paths from the starting node have been explored.
DFS can be implemented in two main ways:
- Recursive approach: Using function calls to go deeper (easier and natural for trees).
- Iterative approach: Using a stack data structure to control the traversal manually.
Pseudocode (Recursive)
function DFS(graph, node, visited):
if node is not visited:
mark node as visited
process(node)
for neighbor in graph[node]:
DFS(graph, neighbor, visited)
Explanation:
graph
is represented as an adjacency list (each node points to a list of neighbors).visited
is a set or array to track visited nodes.- The function visits the node, marks it as visited, processes it (e.g., prints it), and then recursively visits all its unvisited neighbors.
Pseudocode (Iterative)
function DFS(graph, start):
create empty stack
mark start as visited
push start
while stack not empty:
node = pop
process(node)
for neighbor in graph[node]:
if not visited:
mark visited
push neighbor
Explanation:
- Instead of recursive function calls, we use a
stack
to keep track of nodes to visit. - We start with the initial node and push it to the stack.
- While the stack is not empty, pop a node, process it, and push its unvisited neighbors.
Example Use Cases of DFS in Graphs:
- Cycle detection: Check if a graph has any loops.
- Topological sort: Useful in scheduling tasks with dependencies (only works in Directed Acyclic Graphs).
- Connected components: Find all groups of nodes that are connected to each other.
- Maze solving: Traverse paths in games or puzzles.
Time and Space Complexity:
- Time Complexity: O(V + E), where V = number of vertices and E = number of edges. Each node and edge is visited once.
- Space Complexity: O(V), due to recursion stack or the explicit stack and visited set.
3. Tree-Specific DFS Traversals
In trees, DFS is commonly split into 3 types of traversal based on the order in which nodes are visited:
Inorder (Left → Node → Right)
function inorder(node):
if node is not null:
inorder(node.left)
process(node)
inorder(node.right)
Use: In Binary Search Trees (BST), inorder traversal gives sorted order of elements.
Preorder (Node → Left → Right)
function preorder(node):
if node is not null:
process(node)
preorder(node.left)
preorder(node.right)
Use: Used for copying or serializing the tree structure.
Postorder (Left → Right → Node)
function postorder(node):
if node is not null:
postorder(node.left)
postorder(node.right)
process(node)
Use: Often used when deleting or freeing nodes in a tree, or evaluating postfix expressions.
Summary of Use Cases:
- Inorder: Retrieves values from BSTs in sorted order.
- Preorder: Tree construction or exporting structure.
- Postorder: Useful for deleting nodes or evaluating trees.
DFS is a foundational concept in both trees and graphs. Understanding how it works will help you solve a wide range of problems — from searching mazes, building compilers, to designing AI for games.
Try visualizing each traversal on paper or using visualization tools to see how the order changes — it makes understanding the patterns much easier!
When to Use BFS vs DFS
Feature | BFS | DFS |
---|---|---|
Search Type | Level-wise | Depth-wise |
Data Structure | Queue | Stack / Recursion |
Shortest Path | Yes (Unweighted Graph) | No |
Memory Usage | High (due to queue) | Less (recursive stack) |
Backtracking | No | Yes |
Applications of Graph/Tree Traversal
- Shortest paths (BFS in unweighted graphs)
- Cycle detection (DFS)
- Topological sorting
- Spanning trees (DFS, BFS for MSTs)
- Network flows and connectivity
- Parsing expressions and evaluating trees
Advantages and Disadvantages
BFS Advantages
- Guaranteed shortest path in unweighted graphs
- Systematic level-by-level traversal
DFS Advantages
- Memory efficient on sparse graphs
- Can be implemented with recursion
- Useful for backtracking problems
Disadvantages
- BFS can consume more memory (queue)
- DFS may get stuck in cycles if not handled
- Traversal order varies — needs careful handling for reproducibility
Conclusion
Tree and graph traversal techniques are fundamental for exploring structures in DSA. Whether you're checking paths, finding components, or evaluating nodes, BFS and DFS provide the foundation.
Choose traversal based on your problem — BFS for shortest paths and levels, DFS for depth exploration and recursive solutions. Mastering these will unlock powerful strategies across a wide range of problems.
Comments
Loading comments...