
- 1Overview of DSA Problem Solving Techniques
- 2Brute Force Technique in DSA
- 3Greedy Algorithm Technique in DSA
- 4Divide and Conquer Technique in DSA
- 5Dynamic Programming Technique in DSA
- 6Backtracking Technique in DSA
- 7Recursion Technique in DSA
- 8Sliding Window Technique in DSA
- 9Two Pointers Technique
- 10Binary Search Technique
- 11Tree / Graph Traversal Technique in DSA
- 12Bit Manipulation Technique in DSA
- 13Hashing Technique
- 14Heaps Technique in DSA
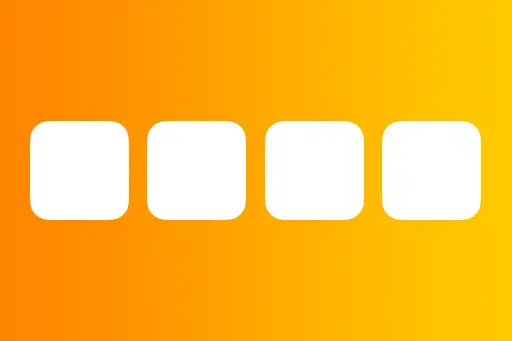
- 1Find Maximum and Minimum in Array using Loop
- 2Find Second Largest in Array
- 3Find Second Smallest in Array
- 4Reverse Array using Two Pointers
- 5Check if Array is Sorted
- 6Remove Duplicates from Sorted Array
- 7Left Rotate an Array by One Place
- 8Left Rotate an Array by K Places
- 9Move Zeroes in Array to End
- 10Linear Search in Array
- 11Union of Two Arrays
- 12Find Missing Number in Array
- 13Max Consecutive Ones in Array
- 14Find Kth Smallest Element
- 15Longest Subarray with Given Sum (Positives)
- 16Longest Subarray with Given Sum (Positives and Negatives)
- 17Find Majority Element in Array (more than n/2 times)
- 18Find Majority Element in Array (more than n/3 times)
- 19Maximum Subarray Sum using Kadane's Algorithm
- 20Print Subarray with Maximum Sum
- 21Stock Buy and Sell
- 22Rearrange Array Alternating Positive and Negative Elements
- 23Next Permutation of Array
- 24Leaders in an Array
- 25Longest Consecutive Sequence in Array
- 26Count Subarrays with Given Sum
- 27Sort an Array of 0s, 1s, and 2s
- 28Two Sum Problem
- 29Three Sum Problem
- 304 Sum Problem
- 31Find Length of Largest Subarray with 0 Sum
- 32Find Maximum Product Subarray

- 1Binary Search in Array using Iteration
- 2Find Lower Bound in Sorted Array
- 3Find Upper Bound in Sorted Array
- 4Search Insert Position in Sorted Array (Lower Bound Approach)
- 5Floor and Ceil in Sorted Array
- 6First Occurrence in a Sorted Array
- 7Last Occurrence in a Sorted Array
- 8Count Occurrences in Sorted Array
- 9Search Element in a Rotated Sorted Array
- 10Search in Rotated Sorted Array with Duplicates
- 11Minimum in Rotated Sorted Array
- 12Find Rotation Count in Sorted Array
- 13Search Single Element in Sorted Array
- 14Find Peak Element in Array
- 15Square Root using Binary Search
- 16Nth Root of a Number using Binary Search
- 17Koko Eating Bananas
- 18Minimum Days to Make M Bouquets
- 19Find the Smallest Divisor Given a Threshold
- 20Capacity to Ship Packages within D Days
- 21Kth Missing Positive Number
- 22Aggressive Cows Problem
- 23Allocate Minimum Number of Pages
- 24Split Array - Minimize Largest Sum
- 25Painter's Partition Problem
- 26Minimize Maximum Distance Between Gas Stations
- 27Median of Two Sorted Arrays of Different Sizes
- 28K-th Element of Two Sorted Arrays
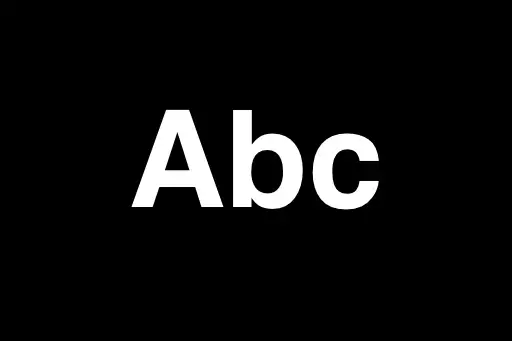
- 1Reverse Words in a String
- 2Find the Largest Odd Number in a Numeric String
- 3Find Longest Common Prefix in Array of Strings
- 4Check If Two Strings Are Isomorphic - Optimal HashMap Solution
- 5Check String Rotation using Concatenation - Optimal Approach
- 6Check if Two Strings Are Anagrams - Optimal Approach
- 7Sort Characters by Frequency - Optimal HashMap and Heap Approach
- 8Find Longest Palindromic Substring - Dynamic Programming Approach
- 9Find Longest Palindromic Substring Without Dynamic Programming
- 10Remove Outermost Parentheses in String
- 11Find Maximum Nesting Depth of Parentheses - Optimal Stack-Free Solution
- 12Convert Roman Numerals to Integer - Efficient Approach
- 13Convert Integer to Roman Numeral - Step-by-Step for Beginners
- 14Implement Atoi - Convert String to Integer in Java
- 15Count Number of Substrings in a String - Explanation with Formula
- 16Edit Distance Problem
- 17Calculate Sum of Beauty of All Substrings - Optimal Approach
- 18Reverse Each Word in a String - Optimal Approach

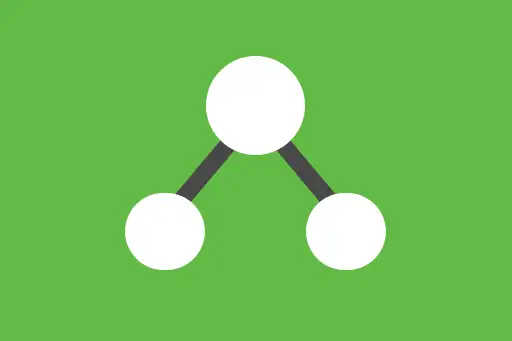
- 1Preorder Traversal of a Binary Tree using Recursion
- 2Preorder Traversal of a Binary Tree using Iteration
- 3Inorder Traversal of a Binary Tree using Recursion
- 4Inorder Traversal of a Binary Tree using Iteration
- 5Postorder Traversal of a Binary Tree Using Recursion
- 6Postorder Traversal of a Binary Tree using Iteration
- 7Level Order Traversal of a Binary Tree using Recursion
- 8Level Order Traversal of a Binary Tree using Iteration
- 9Reverse Level Order Traversal of a Binary Tree using Iteration
- 10Reverse Level Order Traversal of a Binary Tree using Recursion
- 11Find Height of a Binary Tree
- 12Find Diameter of a Binary Tree
- 13Find Mirror of a Binary Tree
- 14Left View of a Binary Tree
- 15Right View of a Binary Tree
- 16Top View of a Binary Tree
- 17Bottom View of a Binary Tree
- 18Zigzag Traversal of a Binary Tree
- 19Check if a Binary Tree is Balanced
- 20Diagonal Traversal of a Binary Tree
- 21Boundary Traversal of a Binary Tree
- 22Construct a Binary Tree from a String with Bracket Representation
- 23Convert a Binary Tree into a Doubly Linked List
- 24Convert a Binary Tree into a Sum Tree
- 25Find Minimum Swaps Required to Convert a Binary Tree into a BST
- 26Check if a Binary Tree is a Sum Tree
- 27Check if All Leaf Nodes are at the Same Level in a Binary Tree
- 28Lowest Common Ancestor (LCA) in a Binary Tree
- 29Solve the Tree Isomorphism Problem
- 30Check if a Binary Tree Contains Duplicate Subtrees of Size 2 or More
- 31Check if Two Binary Trees are Mirror Images
- 32Calculate the Sum of Nodes on the Longest Path from Root to Leaf in a Binary Tree
- 33Print All Paths in a Binary Tree with a Given Sum
- 34Find the Distance Between Two Nodes in a Binary Tree
- 35Find the kth Ancestor of a Node in a Binary Tree
- 36Find All Duplicate Subtrees in a Binary Tree
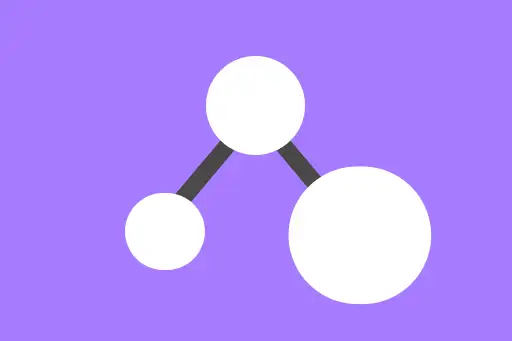
- 1Find a Value in a Binary Search Tree
- 2Delete a Node in a Binary Search Tree
- 3Find the Minimum Value in a Binary Search Tree
- 4Find the Maximum Value in a Binary Search Tree
- 5Find the Inorder Successor in a Binary Search Tree
- 6Find the Inorder Predecessor in a Binary Search Tree
- 7Check if a Binary Tree is a Binary Search Tree
- 8Find the Lowest Common Ancestor of Two Nodes in a Binary Search Tree
- 9Convert a Binary Tree into a Binary Search Tree
- 10Balance a Binary Search Tree
- 11Merge Two Binary Search Trees
- 12Find the kth Largest Element in a Binary Search Tree
- 13Find the kth Smallest Element in a Binary Search Tree
- 14Flatten a Binary Search Tree into a Sorted List

- 1Breadth-First Search in Graphs
- 2Depth-First Search in Graphs
- 3Number of Provinces in an Undirected Graph
- 4Connected Components in a Matrix
- 5Rotten Oranges Problem - BFS in Matrix
- 6Flood Fill Algorithm - Graph Based
- 7Detect Cycle in an Undirected Graph using DFS
- 8Detect Cycle in an Undirected Graph using BFS
- 9Distance of Nearest Cell Having 1 - Grid BFS
- 10Surrounded Regions in Matrix using Graph Traversal
- 11Number of Enclaves in Grid
- 12Word Ladder - Shortest Transformation using Graph
- 13Word Ladder II - All Shortest Transformation Sequences
- 14Number of Distinct Islands using DFS
- 15Check if a Graph is Bipartite using DFS
- 16Topological Sort Using DFS
- 17Topological Sort using Kahn's Algorithm
- 18Cycle Detection in Directed Graph using BFS
- 19Course Schedule - Task Ordering with Prerequisites
- 20Course Schedule 2 - Task Ordering Using Topological Sort
- 21Find Eventual Safe States in a Directed Graph
- 22Alien Dictionary Character Order
- 23Shortest Path in Undirected Graph with Unit Distance
- 24Shortest Path in DAG using Topological Sort
- 25Dijkstra's Algorithm Using Set - Shortest Path in Graph
- 26Dijkstra’s Algorithm Using Priority Queue
- 27Shortest Distance in a Binary Maze using BFS
- 28Path With Minimum Effort in Grid using Graphs
- 29Cheapest Flights Within K Stops - Graph Problem
- 30Number of Ways to Reach Destination in Shortest Time - Graph Problem
- 31Minimum Multiplications to Reach End - Graph BFS
- 32Bellman-Ford Algorithm for Shortest Paths
- 33Floyd Warshall Algorithm for All-Pairs Shortest Path
- 34Find the City With the Fewest Reachable Neighbours
- 35Minimum Spanning Tree in Graphs
- 36Prim's Algorithm for Minimum Spanning Tree
- 37Disjoint Set (Union-Find) with Union by Rank and Path Compression
- 38Kruskal's Algorithm - Minimum Spanning Tree
- 39Minimum Operations to Make Network Connected
- 40Most Stones Removed with Same Row or Column
- 41Accounts Merge Problem using Disjoint Set Union
- 42Number of Islands II - Online Queries using DSU
- 43Making a Large Island Using DSU
- 44Bridges in Graph using Tarjan's Algorithm
- 45Articulation Points in Graphs
- 46Strongly Connected Components using Kosaraju's Algorithm
Calculate the Sum of Nodes on the Longest Path from Root to Leaf in a Binary Tree
⬅ Previous TopicCheck if Two Binary Trees are Mirror Images
Next Topic ⮕Print All Paths in a Binary Tree with a Given Sum
Next Topic ⮕Print All Paths in a Binary Tree with a Given Sum
Problem Statement
Examples
Solution
Algorithm Steps
Code
Python
Java
JavaScript
C
C++
C#
Kotlin
Swift
Go
Php
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def longestPathSum(root):
if not root:
return 0
# If leaf node, return its value
if not root.left and not root.right:
return root.val
leftSum = longestPathSum(root.left)
rightSum = longestPathSum(root.right)
return root.val + max(leftSum, rightSum)
# Example usage:
if __name__ == '__main__':
# Construct binary tree:
# 1
# / \
# 2 3
# / / \
# 4 5 6
root = TreeNode(1, TreeNode(2, TreeNode(4)), TreeNode(3, TreeNode(5), TreeNode(6)))
print("Longest path sum:", longestPathSum(root))