
- 1Overview of DSA Problem Solving Techniques
- 2Brute Force Technique in DSA
- 3Greedy Algorithm Technique in DSA
- 4Divide and Conquer Technique in DSA
- 5Dynamic Programming Technique in DSA
- 6Backtracking Technique in DSA
- 7Recursion Technique in DSA
- 8Sliding Window Technique in DSA
- 9Two Pointers Technique
- 10Binary Search Technique
- 11Tree / Graph Traversal Technique in DSA
- 12Bit Manipulation Technique in DSA
- 13Hashing Technique
- 14Heaps Technique in DSA

- 1Find Maximum and Minimum in Array using Loop
- 2Find Second Largest in Array
- 3Find Second Smallest in Array
- 4Reverse Array using Two Pointers
- 5Check if Array is Sorted
- 6Remove Duplicates from Sorted Array
- 7Left Rotate an Array by One Place
- 8Left Rotate an Array by K Places
- 9Move Zeroes in Array to End
- 10Linear Search in Array
- 11Union of Two Arrays
- 12Find Missing Number in Array
- 13Max Consecutive Ones in Array
- 14Find Kth Smallest Element
- 15Longest Subarray with Given Sum (Positives)
- 16Longest Subarray with Given Sum (Positives and Negatives)
- 17Find Majority Element in Array (more than n/2 times)
- 18Find Majority Element in Array (more than n/3 times)
- 19Maximum Subarray Sum using Kadane's Algorithm
- 20Print Subarray with Maximum Sum
- 21Stock Buy and Sell
- 22Rearrange Array Alternating Positive and Negative Elements
- 23Next Permutation of Array
- 24Leaders in an Array
- 25Longest Consecutive Sequence in Array
- 26Count Subarrays with Given Sum
- 27Sort an Array of 0s, 1s, and 2s
- 28Two Sum Problem
- 29Three Sum Problem
- 304 Sum Problem
- 31Find Length of Largest Subarray with 0 Sum
- 32Find Maximum Product Subarray

- 1Binary Search in Array using Iteration
- 2Find Lower Bound in Sorted Array
- 3Find Upper Bound in Sorted Array
- 4Search Insert Position in Sorted Array (Lower Bound Approach)
- 5Floor and Ceil in Sorted Array
- 6First Occurrence in a Sorted Array
- 7Last Occurrence in a Sorted Array
- 8Count Occurrences in Sorted Array
- 9Search Element in a Rotated Sorted Array
- 10Search in Rotated Sorted Array with Duplicates
- 11Minimum in Rotated Sorted Array
- 12Find Rotation Count in Sorted Array
- 13Search Single Element in Sorted Array
- 14Find Peak Element in Array
- 15Square Root using Binary Search
- 16Nth Root of a Number using Binary Search
- 17Koko Eating Bananas
- 18Minimum Days to Make M Bouquets
- 19Find the Smallest Divisor Given a Threshold
- 20Capacity to Ship Packages within D Days
- 21Kth Missing Positive Number
- 22Aggressive Cows Problem
- 23Allocate Minimum Number of Pages
- 24Split Array - Minimize Largest Sum
- 25Painter's Partition Problem
- 26Minimize Maximum Distance Between Gas Stations
- 27Median of Two Sorted Arrays of Different Sizes
- 28K-th Element of Two Sorted Arrays
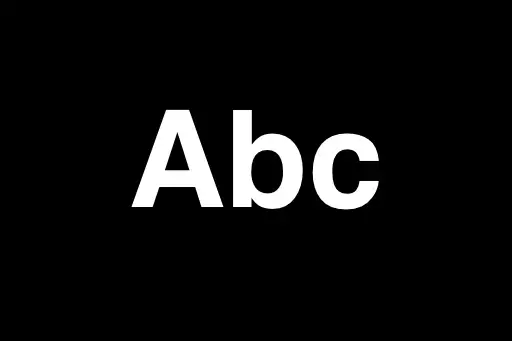
- 1Reverse Words in a String
- 2Find the Largest Odd Number in a Numeric String
- 3Find Longest Common Prefix in Array of Strings
- 4Find Longest Common Substring
- 5Check If Two Strings Are Isomorphic - Optimal HashMap Solution
- 6Check String Rotation using Concatenation - Optimal Approach
- 7Check if Two Strings Are Anagrams - Optimal Approach
- 8Sort Characters by Frequency - Optimal HashMap and Heap Approach
- 9Find Longest Palindromic Substring - Dynamic Programming Approach
- 10Find Longest Palindromic Substring Without Dynamic Programming
- 11Remove Outermost Parentheses in String
- 12Find Maximum Nesting Depth of Parentheses - Optimal Stack-Free Solution
- 13Convert Roman Numerals to Integer - Efficient Approach
- 14Convert Integer to Roman Numeral - Step-by-Step for Beginners
- 15Implement Atoi - Convert String to Integer in Java
- 16Count Number of Substrings in a String - Explanation with Formula
- 17Edit Distance Problem
- 18Calculate Sum of Beauty of All Substrings - Optimal Approach
- 19Reverse Each Word in a String - Optimal Approach

- 1Check if i-th bit is set
- 2Check if a Number is Even/Odd
- 3Check if a Number is Power of 2
- 4Count Number of Set Bits
- 5Swap Two Numbers using XOR
- 6Divide Two Integers without using Multiplication, Division and Modulus Operator
- 7Count Number of Bits to Flip to Convert A to B
- 8Find the Number that Appears Odd Number of Times
- 9Power Set
- 10Find XOR of Numbers from L to R
- 11Prime Factors of a Number
- 12All Divisors of Number
- 13Sieve of Eratosthenes
- 14Find Prime Factorisation of a Number using Sieve
- 15Power(n,x)


- 1Preorder Traversal of a Binary Tree using Recursion
- 2Preorder Traversal of a Binary Tree using Iteration
- 3Inorder Traversal of a Binary Tree using Recursion
- 4Inorder Traversal of a Binary Tree using Iteration
- 5Postorder Traversal of a Binary Tree Using Recursion
- 6Postorder Traversal of a Binary Tree using Iteration
- 7Level Order Traversal of a Binary Tree using Recursion
- 8Level Order Traversal of a Binary Tree using Iteration
- 9Reverse Level Order Traversal of a Binary Tree using Iteration
- 10Reverse Level Order Traversal of a Binary Tree using Recursion
- 11Find Height of a Binary Tree
- 12Find Diameter of a Binary Tree
- 13Find Mirror of a Binary Tree
- 14Left View of a Binary Tree
- 15Right View of a Binary Tree
- 16Top View of a Binary Tree
- 17Bottom View of a Binary Tree
- 18Zigzag Traversal of a Binary Tree
- 19Check if a Binary Tree is Balanced
- 20Diagonal Traversal of a Binary Tree
- 21Boundary Traversal of a Binary Tree
- 22Construct a Binary Tree from a String with Bracket Representation
- 23Convert a Binary Tree into a Doubly Linked List
- 24Convert a Binary Tree into a Sum Tree
- 25Find Minimum Swaps Required to Convert a Binary Tree into a BST
- 26Check if a Binary Tree is a Sum Tree
- 27Check if All Leaf Nodes are at the Same Level in a Binary Tree
- 28Lowest Common Ancestor (LCA) in a Binary Tree
- 29Solve the Tree Isomorphism Problem
- 30Check if a Binary Tree Contains Duplicate Subtrees of Size 2 or More
- 31Check if Two Binary Trees are Mirror Images
- 32Calculate the Sum of Nodes on the Longest Path from Root to Leaf in a Binary Tree
- 33Print All Paths in a Binary Tree with a Given Sum
- 34Find the Distance Between Two Nodes in a Binary Tree
- 35Find the kth Ancestor of a Node in a Binary Tree
- 36Find All Duplicate Subtrees in a Binary Tree

- 1Find a Value in a Binary Search Tree
- 2Delete a Node in a Binary Search Tree
- 3Find the Minimum Value in a Binary Search Tree
- 4Find the Maximum Value in a Binary Search Tree
- 5Find the Inorder Successor in a Binary Search Tree
- 6Find the Inorder Predecessor in a Binary Search Tree
- 7Check if a Binary Tree is a Binary Search Tree
- 8Find the Lowest Common Ancestor of Two Nodes in a Binary Search Tree
- 9Convert a Binary Tree into a Binary Search Tree
- 10Balance a Binary Search Tree
- 11Merge Two Binary Search Trees
- 12Find the kth Largest Element in a Binary Search Tree
- 13Find the kth Smallest Element in a Binary Search Tree
- 14Flatten a Binary Search Tree into a Sorted List

- 1Breadth-First Search in Graphs
- 2Depth-First Search in Graphs
- 3Number of Provinces in an Undirected Graph
- 4Connected Components in a Matrix
- 5Rotten Oranges Problem - BFS in Matrix
- 6Flood Fill Algorithm - Graph Based
- 7Detect Cycle in an Undirected Graph using DFS
- 8Detect Cycle in an Undirected Graph using BFS
- 9Distance of Nearest Cell Having 1 - Grid BFS
- 10Surrounded Regions in Matrix using Graph Traversal
- 11Number of Enclaves in Grid
- 12Word Ladder - Shortest Transformation using Graph
- 13Word Ladder II - All Shortest Transformation Sequences
- 14Number of Distinct Islands using DFS
- 15Check if a Graph is Bipartite using DFS
- 16Topological Sort Using DFS
- 17Topological Sort using Kahn's Algorithm
- 18Cycle Detection in Directed Graph using BFS
- 19Course Schedule - Task Ordering with Prerequisites
- 20Course Schedule 2 - Task Ordering Using Topological Sort
- 21Find Eventual Safe States in a Directed Graph
- 22Alien Dictionary Character Order
- 23Shortest Path in Undirected Graph with Unit Distance
- 24Shortest Path in DAG using Topological Sort
- 25Dijkstra's Algorithm Using Set - Shortest Path in Graph
- 26Dijkstra’s Algorithm Using Priority Queue
- 27Shortest Distance in a Binary Maze using BFS
- 28Path With Minimum Effort in Grid using Graphs
- 29Cheapest Flights Within K Stops - Graph Problem
- 30Number of Ways to Reach Destination in Shortest Time - Graph Problem
- 31Minimum Multiplications to Reach End - Graph BFS
- 32Bellman-Ford Algorithm for Shortest Paths
- 33Floyd Warshall Algorithm for All-Pairs Shortest Path
- 34Find the City With the Fewest Reachable Neighbours
- 35Minimum Spanning Tree in Graphs
- 36Prim's Algorithm for Minimum Spanning Tree
- 37Disjoint Set (Union-Find) with Union by Rank and Path Compression
- 38Kruskal's Algorithm - Minimum Spanning Tree
- 39Minimum Operations to Make Network Connected
- 40Most Stones Removed with Same Row or Column
- 41Accounts Merge Problem using Disjoint Set Union
- 42Number of Islands II - Online Queries using DSU
- 43Making a Large Island Using DSU
- 44Bridges in Graph using Tarjan's Algorithm
- 45Articulation Points in Graphs
- 46Strongly Connected Components using Kosaraju's Algorithm
Brute Force Technique in DSA | Examples with Time Complexities
Next Topic ⮕Greedy Algorithm Technique in DSA
Brute Force in a Nut Shell
- Try all possible combinations or options.
- Easy to implement, but inefficient for large inputs.
What is Brute Force in DSA?
The Brute Force Technique is a straightforward method of solving problems by checking all possible solutions and selecting the correct one. It does not involve any optimization or shortcuts and is often the first approach that comes to mind when solving a problem. While it’s simple to implement, brute force methods are usually inefficient for large inputs due to their high time complexity.
Examples of Brute Force Techniques
1. Linear Search
Problem: Find if an element x
exists in an array.
Brute Force Explanation:
The brute force method for linear search involves scanning each element in the array from the beginning to the end. We do not skip any element or use any optimized lookup (like binary search). If we find the target element x
, we immediately return its index. If we reach the end of the array and haven't found the element, we return -1 indicating it is not present.
// Pseudocode
for i from 0 to n-1:
if arr[i] == x:
return i
return -1
Time Complexity: O(n) — In the worst case, we may need to check all n
elements of the array.
Space Complexity: O(1) — No extra space is used.
2. Checking for Duplicates in an Array
Problem: Given an array of size n
, determine if any value appears at least twice. Return true
if any duplicates exist, otherwise return false
.
Brute Force Approach
This approach uses two nested loops to compare each element with every other element that comes after it. If any two elements are equal, it confirms the presence of a duplicate.
// Pseudocode
for i from 0 to n-1:
for j from i+1 to n-1:
if arr[i] == arr[j]:
return true // Duplicate found
return false // No duplicates found
Step-by-Step Explanation:
- The outer loop selects each element one by one starting from index
0
ton-1
. - The inner loop compares the selected element with all the elements that appear after it.
- If a match is found (i.e.,
arr[i] == arr[j]
), it means a duplicate exists, and we returntrue
. - If no match is found even after all comparisons, we return
false
.
Time Complexity:
- Best Case: O(1) – If the duplicate is found early (e.g., first two elements).
- Worst Case: O(n²) – If the array has all unique elements or duplicate is at the end.
- Average Case: O(n²)
Space Complexity:
- O(1) – No extra space is used; only a few variables for indexing and comparison.
Note: This method is simple and intuitive but inefficient for large arrays. More efficient solutions use Hashing or Sorting techniques with O(n) or O(n log n) time.
3. Maximum Subarray Sum (Naive Way)
Problem: Find the contiguous subarray within a one-dimensional array of numbers that has the largest sum.
Brute Force Approach:
We generate all possible subarrays and calculate the sum for each of them. For every starting index i
, we iterate through all possible ending indices j
such that j ≥ i
. We keep track of the maximum sum encountered during these iterations.
// Pseudocode
maxSum = -infinity
for i from 0 to n-1:
sum = 0
for j from i to n-1:
sum += arr[j]
maxSum = max(maxSum, sum)
return maxSum
Explanation:
- We use two nested loops.
- The outer loop picks the starting index of the subarray.
- The inner loop picks the ending index and calculates the sum from
i
toj
. - The maximum of all such sums is stored and returned as the result.
Time Complexity: O(n²) — because there are approximately n(n+1)/2 subarrays and we compute each sum in constant time using a cumulative approach inside the nested loop.
Space Complexity: O(1) — no extra space is used apart from variables to store sum and maxSum.
4. String Matching (Naive Pattern Search)
Problem: Given a text of length n
and a pattern of length m
, check if the pattern exists in the text and return its starting index if found.
Brute Force Idea: Try matching the pattern at every possible position in the text. For each position i
in the text (from 0
to n - m
), compare the pattern with the substring of text starting from i
. If all characters match, we found the pattern.
// Pseudocode
for i from 0 to n - m:
match = true
for j from 0 to m - 1:
if text[i + j] != pattern[j]:
match = false
break
if match == true:
return i
return -1
Time Complexity: O(n × m)
Explanation: In the worst case, for each of the n - m + 1
starting positions in the text, we may compare up to m
characters with the pattern. Hence, the overall complexity becomes O(n × m)
. This is acceptable for small inputs but inefficient for long texts or large patterns.
When to Use: This brute force approach is useful when performance is not a concern or when pattern matching is needed only occasionally in small strings. It is also a good starting point before optimizing using advanced algorithms like KMP or Rabin-Karp.
Advantages and Disadvantages of Brute Force Technique
Advantages
- Simple to Understand: Brute force algorithms are easy to write and understand, making them ideal for beginners.
- Works for All Problems: It can be applied to almost any problem without needing a deep understanding of advanced concepts.
- Baseline for Optimization: Serves as a good starting point to develop and test problem-solving logic before optimizing.
- Guaranteed Correctness: Since all possibilities are explored, the solution (if found) is guaranteed to be correct.
Disadvantages
- Poor Time Complexity: Brute force methods often involve high time complexity (e.g., O(n²), O(n!) etc.), making them inefficient for large inputs.
- Not Scalable: As the size of input increases, brute force becomes computationally expensive and impractical.
- No Use of Problem Constraints: Brute force ignores patterns, constraints, or mathematical properties that could reduce computation.
- Wastes Resources: It can consume more memory and processing time than optimized approaches.
Conclusion
Brute force techniques are simple and effective for small datasets or when correctness is more important than efficiency. However, they become impractical for large inputs due to high time complexity. Understanding brute force is essential as a foundation before learning optimized approaches.
Comments
Loading comments...