
- 1Overview of DSA Problem Solving Techniques
- 2Brute Force Technique in DSA
- 3Greedy Algorithm Technique in DSA
- 4Divide and Conquer Technique in DSA
- 5Dynamic Programming Technique in DSA
- 6Backtracking Technique in DSA
- 7Recursion Technique in DSA
- 8Sliding Window Technique in DSA
- 9Two Pointers Technique
- 10Binary Search Technique
- 11Tree / Graph Traversal Technique in DSA
- 12Bit Manipulation Technique in DSA
- 13Hashing Technique
- 14Heaps Technique in DSA

- 1Find Maximum and Minimum in Array using Loop
- 2Find Second Largest in Array
- 3Find Second Smallest in Array
- 4Reverse Array using Two Pointers
- 5Check if Array is Sorted
- 6Remove Duplicates from Sorted Array
- 7Left Rotate an Array by One Place
- 8Left Rotate an Array by K Places
- 9Move Zeroes in Array to End
- 10Linear Search in Array
- 11Union of Two Arrays
- 12Find Missing Number in Array
- 13Max Consecutive Ones in Array
- 14Find Kth Smallest Element
- 15Longest Subarray with Given Sum (Positives)
- 16Longest Subarray with Given Sum (Positives and Negatives)
- 17Find Majority Element in Array (more than n/2 times)
- 18Find Majority Element in Array (more than n/3 times)
- 19Maximum Subarray Sum using Kadane's Algorithm
- 20Print Subarray with Maximum Sum
- 21Stock Buy and Sell
- 22Rearrange Array Alternating Positive and Negative Elements
- 23Next Permutation of Array
- 24Leaders in an Array
- 25Longest Consecutive Sequence in Array
- 26Count Subarrays with Given Sum
- 27Sort an Array of 0s, 1s, and 2s
- 28Two Sum Problem
- 29Three Sum Problem
- 304 Sum Problem
- 31Find Length of Largest Subarray with 0 Sum
- 32Find Maximum Product Subarray

- 1Binary Search in Array using Iteration
- 2Find Lower Bound in Sorted Array
- 3Find Upper Bound in Sorted Array
- 4Search Insert Position in Sorted Array (Lower Bound Approach)
- 5Floor and Ceil in Sorted Array
- 6First Occurrence in a Sorted Array
- 7Last Occurrence in a Sorted Array
- 8Count Occurrences in Sorted Array
- 9Search Element in a Rotated Sorted Array
- 10Search in Rotated Sorted Array with Duplicates
- 11Minimum in Rotated Sorted Array
- 12Find Rotation Count in Sorted Array
- 13Search Single Element in Sorted Array
- 14Find Peak Element in Array
- 15Square Root using Binary Search
- 16Nth Root of a Number using Binary Search
- 17Koko Eating Bananas
- 18Minimum Days to Make M Bouquets
- 19Find the Smallest Divisor Given a Threshold
- 20Capacity to Ship Packages within D Days
- 21Kth Missing Positive Number
- 22Aggressive Cows Problem
- 23Allocate Minimum Number of Pages
- 24Split Array - Minimize Largest Sum
- 25Painter's Partition Problem
- 26Minimize Maximum Distance Between Gas Stations
- 27Median of Two Sorted Arrays of Different Sizes
- 28K-th Element of Two Sorted Arrays
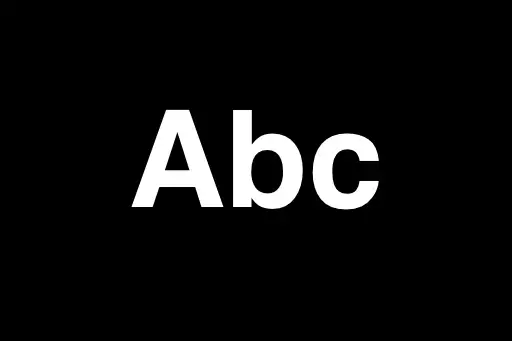
- 1Reverse Words in a String
- 2Find the Largest Odd Number in a Numeric String
- 3Find Longest Common Prefix in Array of Strings
- 4Find Longest Common Substring
- 5Check If Two Strings Are Isomorphic - Optimal HashMap Solution
- 6Check String Rotation using Concatenation - Optimal Approach
- 7Check if Two Strings Are Anagrams - Optimal Approach
- 8Sort Characters by Frequency - Optimal HashMap and Heap Approach
- 9Find Longest Palindromic Substring - Dynamic Programming Approach
- 10Find Longest Palindromic Substring Without Dynamic Programming
- 11Remove Outermost Parentheses in String
- 12Find Maximum Nesting Depth of Parentheses - Optimal Stack-Free Solution
- 13Convert Roman Numerals to Integer - Efficient Approach
- 14Convert Integer to Roman Numeral - Step-by-Step for Beginners
- 15Implement Atoi - Convert String to Integer in Java
- 16Count Number of Substrings in a String - Explanation with Formula
- 17Edit Distance Problem
- 18Calculate Sum of Beauty of All Substrings - Optimal Approach
- 19Reverse Each Word in a String - Optimal Approach

- 1Check if i-th bit is set
- 2Check if a Number is Even/Odd
- 3Check if a Number is Power of 2
- 4Count Number of Set Bits
- 5Swap Two Numbers using XOR
- 6Divide Two Integers without using Multiplication, Division and Modulus Operator
- 7Count Number of Bits to Flip to Convert A to B
- 8Find the Number that Appears Odd Number of Times
- 9Power Set
- 10Find XOR of Numbers from L to R
- 11Prime Factors of a Number
- 12All Divisors of Number
- 13Sieve of Eratosthenes
- 14Find Prime Factorisation of a Number using Sieve
- 15Power(n,x)


- 1Preorder Traversal of a Binary Tree using Recursion
- 2Preorder Traversal of a Binary Tree using Iteration
- 3Inorder Traversal of a Binary Tree using Recursion
- 4Inorder Traversal of a Binary Tree using Iteration
- 5Postorder Traversal of a Binary Tree Using Recursion
- 6Postorder Traversal of a Binary Tree using Iteration
- 7Level Order Traversal of a Binary Tree using Recursion
- 8Level Order Traversal of a Binary Tree using Iteration
- 9Reverse Level Order Traversal of a Binary Tree using Iteration
- 10Reverse Level Order Traversal of a Binary Tree using Recursion
- 11Find Height of a Binary Tree
- 12Find Diameter of a Binary Tree
- 13Find Mirror of a Binary Tree
- 14Left View of a Binary Tree
- 15Right View of a Binary Tree
- 16Top View of a Binary Tree
- 17Bottom View of a Binary Tree
- 18Zigzag Traversal of a Binary Tree
- 19Check if a Binary Tree is Balanced
- 20Diagonal Traversal of a Binary Tree
- 21Boundary Traversal of a Binary Tree
- 22Construct a Binary Tree from a String with Bracket Representation
- 23Convert a Binary Tree into a Doubly Linked List
- 24Convert a Binary Tree into a Sum Tree
- 25Find Minimum Swaps Required to Convert a Binary Tree into a BST
- 26Check if a Binary Tree is a Sum Tree
- 27Check if All Leaf Nodes are at the Same Level in a Binary Tree
- 28Lowest Common Ancestor (LCA) in a Binary Tree
- 29Solve the Tree Isomorphism Problem
- 30Check if a Binary Tree Contains Duplicate Subtrees of Size 2 or More
- 31Check if Two Binary Trees are Mirror Images
- 32Calculate the Sum of Nodes on the Longest Path from Root to Leaf in a Binary Tree
- 33Print All Paths in a Binary Tree with a Given Sum
- 34Find the Distance Between Two Nodes in a Binary Tree
- 35Find the kth Ancestor of a Node in a Binary Tree
- 36Find All Duplicate Subtrees in a Binary Tree

- 1Find a Value in a Binary Search Tree
- 2Delete a Node in a Binary Search Tree
- 3Find the Minimum Value in a Binary Search Tree
- 4Find the Maximum Value in a Binary Search Tree
- 5Find the Inorder Successor in a Binary Search Tree
- 6Find the Inorder Predecessor in a Binary Search Tree
- 7Check if a Binary Tree is a Binary Search Tree
- 8Find the Lowest Common Ancestor of Two Nodes in a Binary Search Tree
- 9Convert a Binary Tree into a Binary Search Tree
- 10Balance a Binary Search Tree
- 11Merge Two Binary Search Trees
- 12Find the kth Largest Element in a Binary Search Tree
- 13Find the kth Smallest Element in a Binary Search Tree
- 14Flatten a Binary Search Tree into a Sorted List

- 1Breadth-First Search in Graphs
- 2Depth-First Search in Graphs
- 3Number of Provinces in an Undirected Graph
- 4Connected Components in a Matrix
- 5Rotten Oranges Problem - BFS in Matrix
- 6Flood Fill Algorithm - Graph Based
- 7Detect Cycle in an Undirected Graph using DFS
- 8Detect Cycle in an Undirected Graph using BFS
- 9Distance of Nearest Cell Having 1 - Grid BFS
- 10Surrounded Regions in Matrix using Graph Traversal
- 11Number of Enclaves in Grid
- 12Word Ladder - Shortest Transformation using Graph
- 13Word Ladder II - All Shortest Transformation Sequences
- 14Number of Distinct Islands using DFS
- 15Check if a Graph is Bipartite using DFS
- 16Topological Sort Using DFS
- 17Topological Sort using Kahn's Algorithm
- 18Cycle Detection in Directed Graph using BFS
- 19Course Schedule - Task Ordering with Prerequisites
- 20Course Schedule 2 - Task Ordering Using Topological Sort
- 21Find Eventual Safe States in a Directed Graph
- 22Alien Dictionary Character Order
- 23Shortest Path in Undirected Graph with Unit Distance
- 24Shortest Path in DAG using Topological Sort
- 25Dijkstra's Algorithm Using Set - Shortest Path in Graph
- 26Dijkstra’s Algorithm Using Priority Queue
- 27Shortest Distance in a Binary Maze using BFS
- 28Path With Minimum Effort in Grid using Graphs
- 29Cheapest Flights Within K Stops - Graph Problem
- 30Number of Ways to Reach Destination in Shortest Time - Graph Problem
- 31Minimum Multiplications to Reach End - Graph BFS
- 32Bellman-Ford Algorithm for Shortest Paths
- 33Floyd Warshall Algorithm for All-Pairs Shortest Path
- 34Find the City With the Fewest Reachable Neighbours
- 35Minimum Spanning Tree in Graphs
- 36Prim's Algorithm for Minimum Spanning Tree
- 37Disjoint Set (Union-Find) with Union by Rank and Path Compression
- 38Kruskal's Algorithm - Minimum Spanning Tree
- 39Minimum Operations to Make Network Connected
- 40Most Stones Removed with Same Row or Column
- 41Accounts Merge Problem using Disjoint Set Union
- 42Number of Islands II - Online Queries using DSU
- 43Making a Large Island Using DSU
- 44Bridges in Graph using Tarjan's Algorithm
- 45Articulation Points in Graphs
- 46Strongly Connected Components using Kosaraju's Algorithm
Bit Manipulation Technique in DSA | Concepts & Applications
What is the Bit Manipulation Technique?
Bit Manipulation is a technique in DSA where we use binary representations of given data and binary operations to solve problems efficiently.
Example: Binary Representation of 57
Let’s break down the number 57 into its binary form and explain it using powers of two.
In binary, 57 is represented as:
To understand how numbers are represented in binary, let’s take the decimal number 57 and convert it into binary step by step.
Step 1: Determine the Highest Power of 2 Less Than or Equal to 57
We start by finding the highest power of 2 less than or equal to 57. That’s 2⁵ = 32
.
Now subtract:
57 - 32 = 25
Step 2: Continue Subtracting Lower Powers of 2
The next largest power of 2 that fits into 25 is 2⁴ = 16
:
25 - 16 = 9
Then 2³ = 8
fits into 9:
9 - 8 = 1
Finally, 2⁰ = 1
fits into 1:
1 - 1 = 0
Step 3: List the Powers of 2 That Were Used
- 2⁵ = 32
- 2⁴ = 16
- 2³ = 8
- 2⁰ = 1
So, we can say:
57 = 2⁵ + 2⁴ + 2³ + 2⁰ = 32 + 16 + 8 + 1
Step 4: Mark These Positions in Binary
Binary digits (bits) represent powers of two from right to left:
Bit Position
Power of 2:
Used in 57?:
This gives us the binary representation of 57:
Binary Representation of First Twenty Numbers
Decimal | Binary |
---|---|
1 | 00000001 |
2 | 00000010 |
3 | 00000011 |
4 | 00000100 |
5 | 00000101 |
6 | 00000110 |
7 | 00000111 |
8 | 00001000 |
9 | 00001001 |
10 | 00001010 |
11 | 00001011 |
12 | 00001100 |
13 | 00001101 |
14 | 00001110 |
15 | 00001111 |
16 | 00010000 |
17 | 00010001 |
18 | 00010010 |
19 | 00010011 |
20 | 00010100 |
Application of Bit Manipulation Technique: Our First Usecase - Checking If a Number is Even
1. Using the Modulus Operator (Conventional Method)
The most common way to check if a number is even is by using the modulus operator:
if num % 2 == 0:
print("Even")
else:
print("Odd")
This checks if the remainder when the number is divided by 2 is zero. If true, the number is even.
2. Using Bit Manipulation (Efficient Technique)
An optimized way to check if a number is even is to use a bitwise AND operation:
if (num & 1) == 0:
print("Even")
else:
print("Odd")
This works because in binary, all even numbers have a least significant bit (LSB) of 0, and odd numbers have LSB of 1.
The expression num & 1
isolates the LSB:
- If LSB is 0, the number is even
- If LSB is 1, the number is odd
3. Why Bit Manipulation is More Efficient
-
The modulus (%) operator takes more time:
When we usenum % 2
to check if a number is even, the computer has to perform a division operation to find the remainder. Division takes more steps for the computer to complete, especially compared to simpler operations. -
Bitwise AND (&) is very fast:
Bitwise operations likenum & 1
work directly on the binary form of the number. These operations are handled at a very low level by the computer — they usually take just one quick step, making them much faster than division. -
Better for loops and repeated checks:
If you need to check hundreds or thousands of numbers — like in a loop — using bit manipulation saves a lot of time. This is important in areas like mobile apps, games, or sensors where speed and performance matter. -
Think of it like this:
Using%
is like using a calculator to divide by 2 every time. Using&
is like glancing at the last digit in binary — it’s instant and efficient. -
In short:
num % 2
= Slower (involves division)num & 1
= Faster (uses binary and needs just one quick step)
4. Example Comparison
Let us check if Decimal: 6 is even or odd.
LSB = 0 → 6 is Even
Let us check if Decimal: 7 is even or odd.
LSB = 1 → 7 is Odd
Therefore, using num & 1
is a faster and more efficient method to check for even or odd numbers,
especially when performing large-scale or repeated computations.
Common Use Cases of Bit Manipulation
- Checking if a number is even or odd
num & 1
returns 0 if even, 1 if odd. - Swapping two variables without using a temporary variable
a = a ^ b; b = a ^ b; a = a ^ b;
- Checking if a number is a power of two
(num & (num - 1)) == 0
fornum > 0
- Counting the number of 1s (set bits) in a number
Known as “Hamming Weight” – can be done usingBrian Kernighan’s algorithm
. - Toggling a specific bit
Use XOR:num ^ (1 << i)
toggles theith
bit. - Setting or clearing a bit
- Set:num | (1 << i)
- Clear:num & ~(1 << i)
- Finding the only odd occurring number in an array
XOR all elements: duplicates cancel out, and the odd one remains. - Fast multiplication/division by powers of two
- Multiply:num << k
→ equivalent tonum * 2k
- Divide:num >> k
→ equivalent tonum / 2k
- Subset generation using bits
For a set ofn
elements, use integers from0
to2n - 1
as masks to generate all subsets. - Bitmask-based Dynamic Programming
Used in optimization problems like Traveling Salesman Problem (TSP), where the state space is represented using bits.
How to Identify If a Problem Needs Bit Manipulation
As a beginner, look for the following clues in a problem:
- You are working with powers of two.
For example, checking if a number is 1, 2, 4, 8, 16... → Think: Bit manipulation. - The problem talks about ON/OFF, True/False, or binary states.
Representing such states compactly can be done using individual bits. - You need to track multiple boolean flags or states efficiently.
Use a bitmask where each bit represents one flag. - You are told to solve something with “no extra space” or “constant space.”
Bit tricks often let you store multiple things in one integer using bitwise operations. - The problem involves toggling, flipping, or switching values.
XOR (^
) is your friend here. - You need to optimize performance in loops with large data.
Bitwise operations are faster than arithmetic operations like*, /, %
. - You need to generate all combinations or subsets.
Using integers and checking their bits can help loop through all possible subsets.
Bitwise Operators
Bit Manipulation techniques rely heavily on bitwise operators. These operators work at the binary level and are extremely useful for efficient computations, especially in low-level or performance-critical programming. Understanding each operator helps you apply them effectively.
& (Bitwise AND)
The &
operator compares each bit of two numbers and returns 1 only if both bits are 1. Otherwise, it returns 0.
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a & b # 0001 → 1
| (Bitwise OR)
The |
operator compares each bit of two numbers and returns 1 if either of the bits is 1.
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a | b # 0111 → 7
^ (Bitwise XOR)
The ^
operator (exclusive OR) returns 1 if the bits are different, and 0 if they are the same.
It's often used to toggle bits or find unique elements.
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a ^ b # 0110 → 6
~ (Bitwise NOT)
The ~
operator inverts each bit of the number — 0s become 1s, and 1s become 0s.
It effectively returns the negative value of the number minus one (i.e., ~n = -n - 1
).
a = 5 # Binary: 00000101
result = ~a # 11111010 → -6
<< (Left Shift)
The <<
operator shifts all bits to the left by a given number of positions.
Each shift multiplies the number by 2.
a = 5 # Binary: 00000101
result = a << 1 # 00001010 → 10 (5 × 2)
result = a << 2 # 00010100 → 20 (5 × 4)
>> (Right Shift)
The >>
operator shifts all bits to the right by a given number of positions.
Each shift divides the number by 2 (discarding the remainder).
a = 5 # Binary: 00000101
result = a >> 1 # 00000010 → 2 (5 // 2)
result = a >> 2 # 00000001 → 1 (5 // 4)
Summary Table
Operator | Name | Description |
---|---|---|
& | AND | 1 if both bits are 1 |
| | OR | 1 if either bit is 1 |
^ | XOR | 1 if bits are different |
~ | NOT | Inverts all bits |
<< | Left Shift | Shifts bits left (×2 per shift) |
>> | Right Shift | Shifts bits right (÷2 per shift) |
Example 2: Check if a Number is a Power of Two — Explained for Beginners
To determine if a number is a power of two using bit manipulation, we rely on the fact that powers of two have a very unique pattern in binary:
- 1 in binary →
0001
- 2 in binary →
0010
- 4 in binary →
0100
- 8 in binary →
1000
Notice a pattern? Every power of two has exactly one bit set to 1 and all other bits are 0.
This is the key to our trick: if a number n
is a power of two, then n
has exactly one set bit. And here’s what happens when you subtract 1 from such a number:
n = 8
(binary =1000
)n - 1 = 7
(binary =0111
)
Now, let’s perform a bitwise AND between n
and n - 1
:
1000 (8)
& 0111 (7)
= 0000
The result is 0
. This will only happen for numbers that are powers of two.
Step-by-Step Breakdown:
- Check if
n
is greater than 0 (since 0 and negatives are not powers of 2). - Use bitwise AND:
n & (n - 1)
- If the result is 0, then
n
is a power of 2. Otherwise, it’s not.
Why Bit Manipulation?
Bit manipulation provides an incredibly fast and elegant solution here. Instead of looping or dividing repeatedly to check powers of 2, we do just one &
operation:
- Time Complexity: O(1)
- Space Complexity: O(1)
This is far more efficient than traditional methods like looping, especially for performance-critical applications like embedded systems, competitive programming, and large-scale simulations.
Pseudocode
// Pseudocode
function isPowerOfTwo(n):
return n > 0 and (n & (n - 1)) == 0
Examples:
n = 16
→ binary =10000
→n & (n-1) = 10000 & 01111 = 0
→ Power of 2n = 18
→ binary =10010
→n & (n-1) ≠ 0
→ ❌ Not a power of 2
Note: This method only works for positive integers. If n ≤ 0
, it's not a power of 2.
Advantages of Bit Manipulation
- Extremely fast (hardware-level operations)
- Memory-efficient
- Elegant for solving subset/flag-based problems
Disadvantages
- Harder to read and debug
- Tricky for beginners to understand and use safely
Conclusion
Bit Manipulation is an efficient and low-level technique in DSA. It is particularly useful when solving problems related to flags, parity, and optimization. Mastering bit operations can lead to elegant and performant solutions for a variety of tricky problems.
Comments
Loading comments...