Course Index
Overview of Techniques14
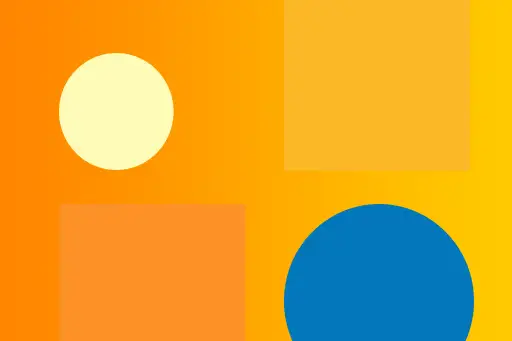
- 1Overview of DSA Problem Solving Techniques
- 2Brute Force Technique in DSA
- 3Greedy Algorithm Technique in DSA
- 4Divide and Conquer Technique in DSA
- 5Dynamic Programming Technique in DSA
- 6Backtracking Technique in DSA
- 7Recursion Technique in DSA
- 8Sliding Window Technique in DSA
- 9Two Pointers Technique
- 10Binary Search Technique
- 11Tree / Graph Traversal Technique in DSA
- 12Bit Manipulation Technique in DSA
- 13Hashing Technique
- 14Heaps Technique in DSA
Sorting10

Arrays32
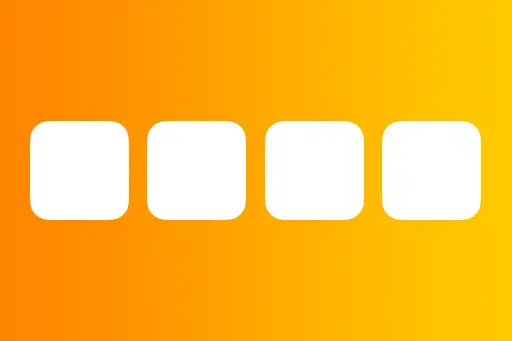
- 1Find Maximum and Minimum in Array using Loop
- 2Find Second Largest in Array
- 3Find Second Smallest in Array
- 4Reverse Array using Two Pointers
- 5Check if Array is Sorted
- 6Remove Duplicates from Sorted Array
- 7Left Rotate an Array by One Place
- 8Left Rotate an Array by K Places
- 9Move Zeroes in Array to End
- 10Linear Search in Array
- 11Union of Two Arrays
- 12Find Missing Number in Array
- 13Max Consecutive Ones in Array
- 14Find Kth Smallest Element
- 15Longest Subarray with Given Sum (Positives)
- 16Longest Subarray with Given Sum (Positives and Negatives)
- 17Find Majority Element in Array (more than n/2 times)
- 18Find Majority Element in Array (more than n/3 times)
- 19Maximum Subarray Sum using Kadane's Algorithm
- 20Print Subarray with Maximum Sum
- 21Stock Buy and Sell
- 22Rearrange Array Alternating Positive and Negative Elements
- 23Next Permutation of Array
- 24Leaders in an Array
- 25Longest Consecutive Sequence in Array
- 26Count Subarrays with Given Sum
- 27Sort an Array of 0s, 1s, and 2s
- 28Two Sum Problem
- 29Three Sum Problem
- 304 Sum Problem
- 31Find Length of Largest Subarray with 0 Sum
- 32Find Maximum Product Subarray
Arrays - Binary Search28

- 1Binary Search in Array using Iteration
- 2Find Lower Bound in Sorted Array
- 3Find Upper Bound in Sorted Array
- 4Search Insert Position in Sorted Array (Lower Bound Approach)
- 5Floor and Ceil in Sorted Array
- 6First Occurrence in a Sorted Array
- 7Last Occurrence in a Sorted Array
- 8Count Occurrences in Sorted Array
- 9Search Element in a Rotated Sorted Array
- 10Search in Rotated Sorted Array with Duplicates
- 11Minimum in Rotated Sorted Array
- 12Find Rotation Count in Sorted Array
- 13Search Single Element in Sorted Array
- 14Find Peak Element in Array
- 15Square Root using Binary Search
- 16Nth Root of a Number using Binary Search
- 17Koko Eating Bananas
- 18Minimum Days to Make M Bouquets
- 19Find the Smallest Divisor Given a Threshold
- 20Capacity to Ship Packages within D Days
- 21Kth Missing Positive Number
- 22Aggressive Cows Problem
- 23Allocate Minimum Number of Pages
- 24Split Array - Minimize Largest Sum
- 25Painter's Partition Problem
- 26Minimize Maximum Distance Between Gas Stations
- 27Median of Two Sorted Arrays of Different Sizes
- 28K-th Element of Two Sorted Arrays
Strings18
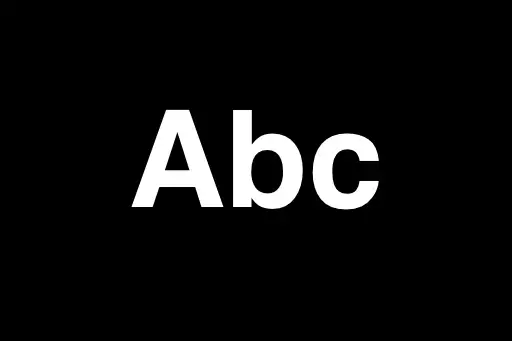
- 1Reverse Words in a String
- 2Find the Largest Odd Number in a Numeric String
- 3Find Longest Common Prefix in Array of Strings
- 4Check If Two Strings Are Isomorphic - Optimal HashMap Solution
- 5Check String Rotation using Concatenation - Optimal Approach
- 6Check if Two Strings Are Anagrams - Optimal Approach
- 7Sort Characters by Frequency - Optimal HashMap and Heap Approach
- 8Find Longest Palindromic Substring - Dynamic Programming Approach
- 9Find Longest Palindromic Substring Without Dynamic Programming
- 10Remove Outermost Parentheses in String
- 11Find Maximum Nesting Depth of Parentheses - Optimal Stack-Free Solution
- 12Convert Roman Numerals to Integer - Efficient Approach
- 13Convert Integer to Roman Numeral - Step-by-Step for Beginners
- 14Implement Atoi - Convert String to Integer in Java
- 15Count Number of Substrings in a String - Explanation with Formula
- 16Edit Distance Problem
- 17Calculate Sum of Beauty of All Substrings - Optimal Approach
- 18Reverse Each Word in a String - Optimal Approach
Matrix - Binary Search5

Binary Trees36
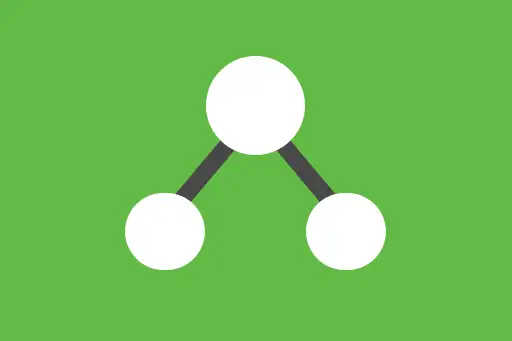
- 1Preorder Traversal of a Binary Tree using Recursion
- 2Preorder Traversal of a Binary Tree using Iteration
- 3Postorder Traversal of a Binary Tree Using Recursion
- 4Postorder Traversal of a Binary Tree using Iteration
- 5Level Order Traversal of a Binary Tree using Recursion
- 6Level Order Traversal of a Binary Tree using Iteration
- 7Reverse Level Order Traversal of a Binary Tree using Iteration
- 8Reverse Level Order Traversal of a Binary Tree using Recursion
- 9Find Height of a Binary Tree
- 10Find Diameter of a Binary Tree
- 11Find Mirror of a Binary Tree - Todo
- 12Inorder Traversal of a Binary Tree using Recursion
- 13Inorder Traversal of a Binary Tree using Iteration
- 14Left View of a Binary Tree
- 15Right View of a Binary Tree
- 16Top View of a Binary Tree
- 17Bottom View of a Binary Tree
- 18Zigzag Traversal of a Binary Tree
- 19Check if a Binary Tree is Balanced
- 20Diagonal Traversal of a Binary Tree
- 21Boundary Traversal of a Binary Tree
- 22Construct a Binary Tree from a String with Bracket Representation
- 23Convert a Binary Tree into a Doubly Linked List
- 24Convert a Binary Tree into a Sum Tree
- 25Find Minimum Swaps Required to Convert a Binary Tree into a BST
- 26Check if a Binary Tree is a Sum Tree
- 27Check if All Leaf Nodes are at the Same Level in a Binary Tree
- 28Lowest Common Ancestor (LCA) in a Binary Tree
- 29Solve the Tree Isomorphism Problem
- 30Check if a Binary Tree Contains Duplicate Subtrees of Size 2 or More
- 31Check if Two Binary Trees are Mirror Images
- 32Calculate the Sum of Nodes on the Longest Path from Root to Leaf in a Binary Tree
- 33Print All Paths in a Binary Tree with a Given Sum
- 34Find the Distance Between Two Nodes in a Binary Tree
- 35Find the kth Ancestor of a Node in a Binary Tree
- 36Find All Duplicate Subtrees in a Binary Tree
Binary Search Trees14
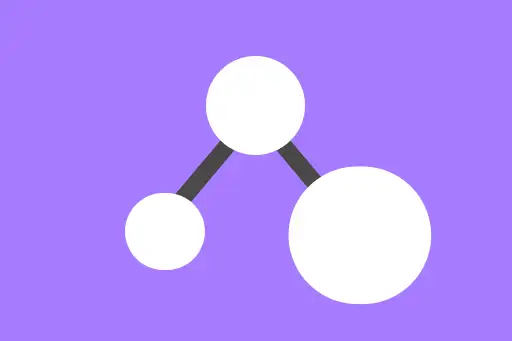
- 1Find a Value in a Binary Search Tree
- 2Delete a Node in a Binary Search Tree
- 3Find the Minimum Value in a Binary Search Tree
- 4Find the Maximum Value in a Binary Search Tree
- 5Find the Inorder Successor in a Binary Search Tree
- 6Find the Inorder Predecessor in a Binary Search Tree
- 7Check if a Binary Tree is a Binary Search Tree
- 8Find the Lowest Common Ancestor of Two Nodes in a Binary Search Tree
- 9Convert a Binary Tree into a Binary Search Tree
- 10Balance a Binary Search Tree
- 11Merge Two Binary Search Trees
- 12Find the kth Largest Element in a Binary Search Tree
- 13Find the kth Smallest Element in a Binary Search Tree
- 14Flatten a Binary Search Tree into a Sorted List