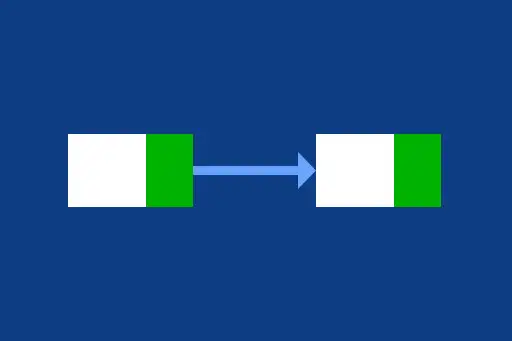
Singly linked lists are one of the most fundamental and flexible linear data structures in computer science. Unlike arrays, which store elements in contiguous memory, a singly linked list consists of nodes where each node stores a value and a reference to the next node. This structure allows dynamic memory usage and efficient insertions and deletions.
What is a Singly Linked List?
A singly linked list is a collection of nodes arranged in a linear sequence. Each node contains two parts: a data field that stores the actual value and a next pointer that points to the next node in the sequence. The list starts from a special node called the head
, and the last node's next pointer is null
, indicating the end of the list.
Key Features of Singly Linked Lists
1. Dynamic Memory Allocation
Linked lists do not require predefined size. Nodes are allocated dynamically as elements are added, which makes them suitable for situations where the size of the data is unknown or changes frequently.
2. Sequential Access
Unlike arrays, linked lists do not support direct access by index. To reach a particular node, traversal must begin from the head and proceed node by node, following the next
pointers.
3. Efficient Insertions and Deletions
Inserting or deleting elements at the beginning or middle of a linked list is efficient because it only involves reassigning pointers. There is no need to shift elements as in arrays.
4. No Wasted Memory
Since memory is allocated as needed, there is no unused or pre-allocated space like in arrays. This can be more memory-efficient, especially in systems with limited resources.
5. Pointer-Based Structure
Each node maintains a reference to the next, which allows the structure to grow or shrink as needed. However, this also means extra space is required for storing pointers in addition to data.
Common Use Cases of Singly Linked Lists
1. Dynamic Data Structures
Linked lists are ideal for dynamic data structures like stacks, queues, and hash tables (with chaining). They support efficient memory usage and can grow or shrink easily.
2. Implementation of Adjacency Lists
In graph algorithms, adjacency lists often use linked lists to store neighbors of each node. This allows efficient traversal and storage for sparse graphs.
3. Undo/Redo Functionality
Applications like text editors or IDEs use singly linked lists to maintain history of operations. Each action forms a node, and navigating back and forth uses pointer manipulation.
4. Streaming Buffers and Real-Time Processing
In systems where data is streamed (e.g., audio processing, log collection), singly linked lists provide a flexible way to capture and process inputs sequentially without knowing the total size in advance.
5. Low-Level Memory Management
Singly linked lists are commonly used in custom memory allocators and garbage collectors for managing blocks of memory or keeping track of free lists.