
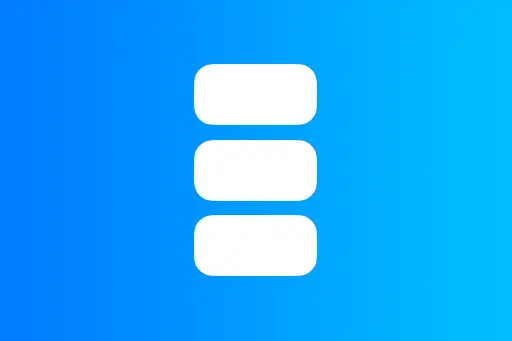
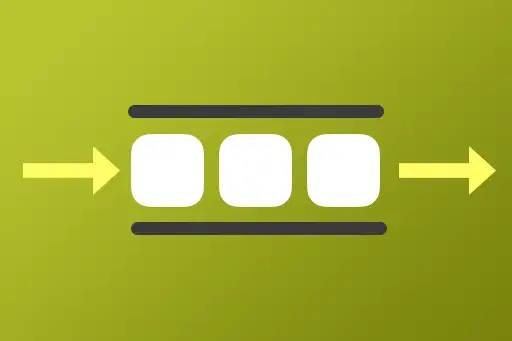
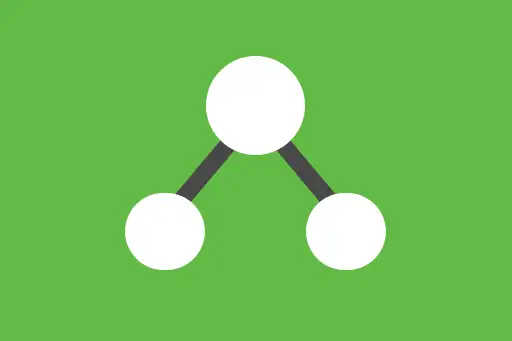

Binary Tree Introduction
Binary trees are a fundamental hierarchical data structure where each node has at most two children: one on the left and one on the right. Unlike linear structures like arrays and linked lists, binary trees allow data to be stored in a branching format, making them efficient for searching, sorting, and hierarchical representation.
What is a Binary Tree?
A binary tree is made up of nodes connected by edges. Each node contains three parts: the data, a reference to the left child, and a reference to the right child. The tree starts from the root
node, and every node can recursively act as the root of its own subtree.
Key Features of Binary Trees
1. Hierarchical Structure
Binary trees represent data hierarchically. Each parent node can have up to two children, making the structure suitable for recursive processing and decision-based logic (like decision trees).
2. Recursive Nature
Binary trees are inherently recursive. Each subtree is itself a binary tree, allowing recursive algorithms to traverse or manipulate them efficiently using techniques like in-order, pre-order, and post-order traversal.
3. Flexible Shape
Binary trees do not need to be complete or balanced. Nodes can have 0, 1, or 2 children, which allows them to represent a wide range of configurations including degenerate (linked list-like) trees or full trees.
4. Memory Efficient Linking
Each node only stores two references (left and right), making the memory footprint predictable. Unlike arrays, binary trees do not require contiguous memory, enabling better space utilization for large datasets.
5. Foundation for Complex Structures
Binary trees are the basis for many advanced data structures such as binary search trees (BSTs), heaps, segment trees, and AVL trees — each optimizing different operations like search, insert, and balance.
Common Use Cases of Binary Trees
1. Binary Search Trees (BSTs)
Binary trees can be arranged as BSTs where the left child is smaller and the right child is greater than the parent. This property allows fast O(log N)
search, insert, and delete operations in balanced trees.
2. Expression Parsing
In compilers and interpreters, binary trees are used to parse and evaluate mathematical expressions. Each internal node is an operator, and leaves are operands, forming an expression tree.
3. Hierarchical Data Representation
Binary trees are used to model hierarchical data such as file systems, organizational charts, and family trees. Their recursive structure aligns well with real-world nested data.
4. Priority Queues and Heaps
Binary heaps (a special type of binary tree) are used to implement priority queues, which are essential in algorithms like Dijkstra’s shortest path, A* search, and job scheduling systems.
5. Balanced Tree Structures
Balanced binary trees like AVL and Red-Black trees maintain height balance automatically, ensuring optimal time complexity for dynamic sets and map structures in databases and in-memory stores.
Comments
Loading comments...